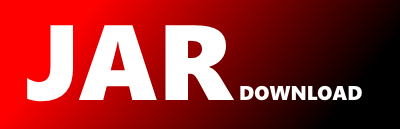
com.pulumi.azurenative.azurestackhci.outputs.SubnetResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurestackhci.outputs;
import com.pulumi.azurenative.azurestackhci.outputs.IPPoolResponse;
import com.pulumi.azurenative.azurestackhci.outputs.RouteTableResponse;
import com.pulumi.azurenative.azurestackhci.outputs.SubnetPropertiesFormatResponseIpConfigurationReferences;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SubnetResponse {
/**
* @return The address prefix for the subnet: Cidr for this subnet - IPv4, IPv6.
*
*/
private @Nullable String addressPrefix;
/**
* @return List of address prefixes for the subnet.
*
*/
private @Nullable List addressPrefixes;
/**
* @return IPAllocationMethod - The IP address allocation method. Possible values include: 'Static', 'Dynamic'
*
*/
private @Nullable String ipAllocationMethod;
/**
* @return IPConfigurationReferences - list of IPConfigurationReferences
*
*/
private @Nullable List ipConfigurationReferences;
/**
* @return network associated pool of IP Addresses
*
*/
private @Nullable List ipPools;
/**
* @return Name - The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
private @Nullable String name;
/**
* @return Route table resource.
*
*/
private @Nullable RouteTableResponse routeTable;
/**
* @return Vlan to use for the subnet
*
*/
private @Nullable Integer vlan;
private SubnetResponse() {}
/**
* @return The address prefix for the subnet: Cidr for this subnet - IPv4, IPv6.
*
*/
public Optional addressPrefix() {
return Optional.ofNullable(this.addressPrefix);
}
/**
* @return List of address prefixes for the subnet.
*
*/
public List addressPrefixes() {
return this.addressPrefixes == null ? List.of() : this.addressPrefixes;
}
/**
* @return IPAllocationMethod - The IP address allocation method. Possible values include: 'Static', 'Dynamic'
*
*/
public Optional ipAllocationMethod() {
return Optional.ofNullable(this.ipAllocationMethod);
}
/**
* @return IPConfigurationReferences - list of IPConfigurationReferences
*
*/
public List ipConfigurationReferences() {
return this.ipConfigurationReferences == null ? List.of() : this.ipConfigurationReferences;
}
/**
* @return network associated pool of IP Addresses
*
*/
public List ipPools() {
return this.ipPools == null ? List.of() : this.ipPools;
}
/**
* @return Name - The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Route table resource.
*
*/
public Optional routeTable() {
return Optional.ofNullable(this.routeTable);
}
/**
* @return Vlan to use for the subnet
*
*/
public Optional vlan() {
return Optional.ofNullable(this.vlan);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SubnetResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String addressPrefix;
private @Nullable List addressPrefixes;
private @Nullable String ipAllocationMethod;
private @Nullable List ipConfigurationReferences;
private @Nullable List ipPools;
private @Nullable String name;
private @Nullable RouteTableResponse routeTable;
private @Nullable Integer vlan;
public Builder() {}
public Builder(SubnetResponse defaults) {
Objects.requireNonNull(defaults);
this.addressPrefix = defaults.addressPrefix;
this.addressPrefixes = defaults.addressPrefixes;
this.ipAllocationMethod = defaults.ipAllocationMethod;
this.ipConfigurationReferences = defaults.ipConfigurationReferences;
this.ipPools = defaults.ipPools;
this.name = defaults.name;
this.routeTable = defaults.routeTable;
this.vlan = defaults.vlan;
}
@CustomType.Setter
public Builder addressPrefix(@Nullable String addressPrefix) {
this.addressPrefix = addressPrefix;
return this;
}
@CustomType.Setter
public Builder addressPrefixes(@Nullable List addressPrefixes) {
this.addressPrefixes = addressPrefixes;
return this;
}
public Builder addressPrefixes(String... addressPrefixes) {
return addressPrefixes(List.of(addressPrefixes));
}
@CustomType.Setter
public Builder ipAllocationMethod(@Nullable String ipAllocationMethod) {
this.ipAllocationMethod = ipAllocationMethod;
return this;
}
@CustomType.Setter
public Builder ipConfigurationReferences(@Nullable List ipConfigurationReferences) {
this.ipConfigurationReferences = ipConfigurationReferences;
return this;
}
public Builder ipConfigurationReferences(SubnetPropertiesFormatResponseIpConfigurationReferences... ipConfigurationReferences) {
return ipConfigurationReferences(List.of(ipConfigurationReferences));
}
@CustomType.Setter
public Builder ipPools(@Nullable List ipPools) {
this.ipPools = ipPools;
return this;
}
public Builder ipPools(IPPoolResponse... ipPools) {
return ipPools(List.of(ipPools));
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder routeTable(@Nullable RouteTableResponse routeTable) {
this.routeTable = routeTable;
return this;
}
@CustomType.Setter
public Builder vlan(@Nullable Integer vlan) {
this.vlan = vlan;
return this;
}
public SubnetResponse build() {
final var _resultValue = new SubnetResponse();
_resultValue.addressPrefix = addressPrefix;
_resultValue.addressPrefixes = addressPrefixes;
_resultValue.ipAllocationMethod = ipAllocationMethod;
_resultValue.ipConfigurationReferences = ipConfigurationReferences;
_resultValue.ipPools = ipPools;
_resultValue.name = name;
_resultValue.routeTable = routeTable;
_resultValue.vlan = vlan;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy