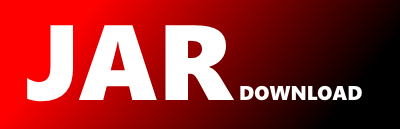
com.pulumi.azurenative.billing.BillingFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.billing;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.billing.inputs.GetAssociatedTenantArgs;
import com.pulumi.azurenative.billing.inputs.GetAssociatedTenantPlainArgs;
import com.pulumi.azurenative.billing.inputs.GetBillingProfileArgs;
import com.pulumi.azurenative.billing.inputs.GetBillingProfilePlainArgs;
import com.pulumi.azurenative.billing.inputs.GetBillingRoleAssignmentByBillingAccountArgs;
import com.pulumi.azurenative.billing.inputs.GetBillingRoleAssignmentByBillingAccountPlainArgs;
import com.pulumi.azurenative.billing.inputs.GetBillingRoleAssignmentByDepartmentArgs;
import com.pulumi.azurenative.billing.inputs.GetBillingRoleAssignmentByDepartmentPlainArgs;
import com.pulumi.azurenative.billing.inputs.GetBillingRoleAssignmentByEnrollmentAccountArgs;
import com.pulumi.azurenative.billing.inputs.GetBillingRoleAssignmentByEnrollmentAccountPlainArgs;
import com.pulumi.azurenative.billing.inputs.GetInvoiceSectionArgs;
import com.pulumi.azurenative.billing.inputs.GetInvoiceSectionPlainArgs;
import com.pulumi.azurenative.billing.inputs.ListBillingAccountInvoiceSectionsByCreateSubscriptionPermissionArgs;
import com.pulumi.azurenative.billing.inputs.ListBillingAccountInvoiceSectionsByCreateSubscriptionPermissionPlainArgs;
import com.pulumi.azurenative.billing.outputs.GetAssociatedTenantResult;
import com.pulumi.azurenative.billing.outputs.GetBillingProfileResult;
import com.pulumi.azurenative.billing.outputs.GetBillingRoleAssignmentByBillingAccountResult;
import com.pulumi.azurenative.billing.outputs.GetBillingRoleAssignmentByDepartmentResult;
import com.pulumi.azurenative.billing.outputs.GetBillingRoleAssignmentByEnrollmentAccountResult;
import com.pulumi.azurenative.billing.outputs.GetInvoiceSectionResult;
import com.pulumi.azurenative.billing.outputs.ListBillingAccountInvoiceSectionsByCreateSubscriptionPermissionResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class BillingFunctions {
/**
* Gets an associated tenant by ID.
* Azure REST API version: 2024-04-01.
*
*/
public static Output getAssociatedTenant(GetAssociatedTenantArgs args) {
return getAssociatedTenant(args, InvokeOptions.Empty);
}
/**
* Gets an associated tenant by ID.
* Azure REST API version: 2024-04-01.
*
*/
public static CompletableFuture getAssociatedTenantPlain(GetAssociatedTenantPlainArgs args) {
return getAssociatedTenantPlain(args, InvokeOptions.Empty);
}
/**
* Gets an associated tenant by ID.
* Azure REST API version: 2024-04-01.
*
*/
public static Output getAssociatedTenant(GetAssociatedTenantArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:billing:getAssociatedTenant", TypeShape.of(GetAssociatedTenantResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an associated tenant by ID.
* Azure REST API version: 2024-04-01.
*
*/
public static CompletableFuture getAssociatedTenantPlain(GetAssociatedTenantPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:billing:getAssociatedTenant", TypeShape.of(GetAssociatedTenantResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a billing profile by its ID. The operation is supported for billing accounts with agreement type Microsoft Customer Agreement and Microsoft Partner Agreement.
* Azure REST API version: 2024-04-01.
*
*/
public static Output getBillingProfile(GetBillingProfileArgs args) {
return getBillingProfile(args, InvokeOptions.Empty);
}
/**
* Gets a billing profile by its ID. The operation is supported for billing accounts with agreement type Microsoft Customer Agreement and Microsoft Partner Agreement.
* Azure REST API version: 2024-04-01.
*
*/
public static CompletableFuture getBillingProfilePlain(GetBillingProfilePlainArgs args) {
return getBillingProfilePlain(args, InvokeOptions.Empty);
}
/**
* Gets a billing profile by its ID. The operation is supported for billing accounts with agreement type Microsoft Customer Agreement and Microsoft Partner Agreement.
* Azure REST API version: 2024-04-01.
*
*/
public static Output getBillingProfile(GetBillingProfileArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:billing:getBillingProfile", TypeShape.of(GetBillingProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a billing profile by its ID. The operation is supported for billing accounts with agreement type Microsoft Customer Agreement and Microsoft Partner Agreement.
* Azure REST API version: 2024-04-01.
*
*/
public static CompletableFuture getBillingProfilePlain(GetBillingProfilePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:billing:getBillingProfile", TypeShape.of(GetBillingProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a role assignment for the caller on a billing account. The operation is supported for billing accounts with agreement type Microsoft Partner Agreement, Microsoft Customer Agreement or Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getBillingRoleAssignmentByBillingAccount(GetBillingRoleAssignmentByBillingAccountArgs args) {
return getBillingRoleAssignmentByBillingAccount(args, InvokeOptions.Empty);
}
/**
* Gets a role assignment for the caller on a billing account. The operation is supported for billing accounts with agreement type Microsoft Partner Agreement, Microsoft Customer Agreement or Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getBillingRoleAssignmentByBillingAccountPlain(GetBillingRoleAssignmentByBillingAccountPlainArgs args) {
return getBillingRoleAssignmentByBillingAccountPlain(args, InvokeOptions.Empty);
}
/**
* Gets a role assignment for the caller on a billing account. The operation is supported for billing accounts with agreement type Microsoft Partner Agreement, Microsoft Customer Agreement or Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getBillingRoleAssignmentByBillingAccount(GetBillingRoleAssignmentByBillingAccountArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:billing:getBillingRoleAssignmentByBillingAccount", TypeShape.of(GetBillingRoleAssignmentByBillingAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a role assignment for the caller on a billing account. The operation is supported for billing accounts with agreement type Microsoft Partner Agreement, Microsoft Customer Agreement or Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getBillingRoleAssignmentByBillingAccountPlain(GetBillingRoleAssignmentByBillingAccountPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:billing:getBillingRoleAssignmentByBillingAccount", TypeShape.of(GetBillingRoleAssignmentByBillingAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a role assignment for the caller on a department. The operation is supported only for billing accounts with agreement type Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getBillingRoleAssignmentByDepartment(GetBillingRoleAssignmentByDepartmentArgs args) {
return getBillingRoleAssignmentByDepartment(args, InvokeOptions.Empty);
}
/**
* Gets a role assignment for the caller on a department. The operation is supported only for billing accounts with agreement type Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getBillingRoleAssignmentByDepartmentPlain(GetBillingRoleAssignmentByDepartmentPlainArgs args) {
return getBillingRoleAssignmentByDepartmentPlain(args, InvokeOptions.Empty);
}
/**
* Gets a role assignment for the caller on a department. The operation is supported only for billing accounts with agreement type Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getBillingRoleAssignmentByDepartment(GetBillingRoleAssignmentByDepartmentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:billing:getBillingRoleAssignmentByDepartment", TypeShape.of(GetBillingRoleAssignmentByDepartmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a role assignment for the caller on a department. The operation is supported only for billing accounts with agreement type Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getBillingRoleAssignmentByDepartmentPlain(GetBillingRoleAssignmentByDepartmentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:billing:getBillingRoleAssignmentByDepartment", TypeShape.of(GetBillingRoleAssignmentByDepartmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a role assignment for the caller on a enrollment Account. The operation is supported only for billing accounts with agreement type Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getBillingRoleAssignmentByEnrollmentAccount(GetBillingRoleAssignmentByEnrollmentAccountArgs args) {
return getBillingRoleAssignmentByEnrollmentAccount(args, InvokeOptions.Empty);
}
/**
* Gets a role assignment for the caller on a enrollment Account. The operation is supported only for billing accounts with agreement type Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getBillingRoleAssignmentByEnrollmentAccountPlain(GetBillingRoleAssignmentByEnrollmentAccountPlainArgs args) {
return getBillingRoleAssignmentByEnrollmentAccountPlain(args, InvokeOptions.Empty);
}
/**
* Gets a role assignment for the caller on a enrollment Account. The operation is supported only for billing accounts with agreement type Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getBillingRoleAssignmentByEnrollmentAccount(GetBillingRoleAssignmentByEnrollmentAccountArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:billing:getBillingRoleAssignmentByEnrollmentAccount", TypeShape.of(GetBillingRoleAssignmentByEnrollmentAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a role assignment for the caller on a enrollment Account. The operation is supported only for billing accounts with agreement type Enterprise Agreement.
* Azure REST API version: 2019-10-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getBillingRoleAssignmentByEnrollmentAccountPlain(GetBillingRoleAssignmentByEnrollmentAccountPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:billing:getBillingRoleAssignmentByEnrollmentAccount", TypeShape.of(GetBillingRoleAssignmentByEnrollmentAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an invoice section by its ID. The operation is supported only for billing accounts with agreement type Microsoft Customer Agreement.
* Azure REST API version: 2024-04-01.
*
*/
public static Output getInvoiceSection(GetInvoiceSectionArgs args) {
return getInvoiceSection(args, InvokeOptions.Empty);
}
/**
* Gets an invoice section by its ID. The operation is supported only for billing accounts with agreement type Microsoft Customer Agreement.
* Azure REST API version: 2024-04-01.
*
*/
public static CompletableFuture getInvoiceSectionPlain(GetInvoiceSectionPlainArgs args) {
return getInvoiceSectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets an invoice section by its ID. The operation is supported only for billing accounts with agreement type Microsoft Customer Agreement.
* Azure REST API version: 2024-04-01.
*
*/
public static Output getInvoiceSection(GetInvoiceSectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:billing:getInvoiceSection", TypeShape.of(GetInvoiceSectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an invoice section by its ID. The operation is supported only for billing accounts with agreement type Microsoft Customer Agreement.
* Azure REST API version: 2024-04-01.
*
*/
public static CompletableFuture getInvoiceSectionPlain(GetInvoiceSectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:billing:getInvoiceSection", TypeShape.of(GetInvoiceSectionResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the invoice sections for which the user has permission to create Azure subscriptions. The operation is supported only for billing accounts with agreement type Microsoft Customer Agreement.
* Azure REST API version: 2020-05-01.
*
* Other available API versions: 2019-10-01-preview, 2024-04-01.
*
*/
public static Output listBillingAccountInvoiceSectionsByCreateSubscriptionPermission(ListBillingAccountInvoiceSectionsByCreateSubscriptionPermissionArgs args) {
return listBillingAccountInvoiceSectionsByCreateSubscriptionPermission(args, InvokeOptions.Empty);
}
/**
* Lists the invoice sections for which the user has permission to create Azure subscriptions. The operation is supported only for billing accounts with agreement type Microsoft Customer Agreement.
* Azure REST API version: 2020-05-01.
*
* Other available API versions: 2019-10-01-preview, 2024-04-01.
*
*/
public static CompletableFuture listBillingAccountInvoiceSectionsByCreateSubscriptionPermissionPlain(ListBillingAccountInvoiceSectionsByCreateSubscriptionPermissionPlainArgs args) {
return listBillingAccountInvoiceSectionsByCreateSubscriptionPermissionPlain(args, InvokeOptions.Empty);
}
/**
* Lists the invoice sections for which the user has permission to create Azure subscriptions. The operation is supported only for billing accounts with agreement type Microsoft Customer Agreement.
* Azure REST API version: 2020-05-01.
*
* Other available API versions: 2019-10-01-preview, 2024-04-01.
*
*/
public static Output listBillingAccountInvoiceSectionsByCreateSubscriptionPermission(ListBillingAccountInvoiceSectionsByCreateSubscriptionPermissionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:billing:listBillingAccountInvoiceSectionsByCreateSubscriptionPermission", TypeShape.of(ListBillingAccountInvoiceSectionsByCreateSubscriptionPermissionResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the invoice sections for which the user has permission to create Azure subscriptions. The operation is supported only for billing accounts with agreement type Microsoft Customer Agreement.
* Azure REST API version: 2020-05-01.
*
* Other available API versions: 2019-10-01-preview, 2024-04-01.
*
*/
public static CompletableFuture listBillingAccountInvoiceSectionsByCreateSubscriptionPermissionPlain(ListBillingAccountInvoiceSectionsByCreateSubscriptionPermissionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:billing:listBillingAccountInvoiceSectionsByCreateSubscriptionPermission", TypeShape.of(ListBillingAccountInvoiceSectionsByCreateSubscriptionPermissionResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy