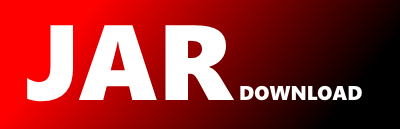
com.pulumi.azurenative.billing.inputs.BillingProfilePropertiesBillToArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.billing.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Billing address.
*
*/
public final class BillingProfilePropertiesBillToArgs extends com.pulumi.resources.ResourceArgs {
public static final BillingProfilePropertiesBillToArgs Empty = new BillingProfilePropertiesBillToArgs();
/**
* Address line 1.
*
*/
@Import(name="addressLine1", required=true)
private Output addressLine1;
/**
* @return Address line 1.
*
*/
public Output addressLine1() {
return this.addressLine1;
}
/**
* Address line 2.
*
*/
@Import(name="addressLine2")
private @Nullable Output addressLine2;
/**
* @return Address line 2.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy