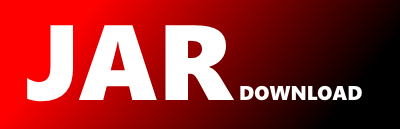
com.pulumi.azurenative.billing.outputs.BillingProfilePropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.billing.outputs;
import com.pulumi.azurenative.billing.outputs.AzurePlanResponse;
import com.pulumi.azurenative.billing.outputs.BillingProfilePropertiesResponseBillTo;
import com.pulumi.azurenative.billing.outputs.BillingProfilePropertiesResponseCurrentPaymentTerm;
import com.pulumi.azurenative.billing.outputs.BillingProfilePropertiesResponseIndirectRelationshipInfo;
import com.pulumi.azurenative.billing.outputs.BillingProfilePropertiesResponseShipTo;
import com.pulumi.azurenative.billing.outputs.BillingProfilePropertiesResponseSoldTo;
import com.pulumi.azurenative.billing.outputs.PaymentTermResponse;
import com.pulumi.azurenative.billing.outputs.SpendingLimitDetailsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class BillingProfilePropertiesResponse {
/**
* @return Billing address.
*
*/
private @Nullable BillingProfilePropertiesResponseBillTo billTo;
/**
* @return Identifies the billing relationship represented by the billing profile. The billing relationship may be between Microsoft, the customer, and/or a third-party.
*
*/
private String billingRelationshipType;
/**
* @return The currency in which the charges for the billing profile are billed.
*
*/
private String currency;
/**
* @return The current payment term of the billing profile.
*
*/
private @Nullable BillingProfilePropertiesResponseCurrentPaymentTerm currentPaymentTerm;
/**
* @return The name of the billing profile.
*
*/
private @Nullable String displayName;
/**
* @return Information about the enabled azure plans.
*
*/
private @Nullable List enabledAzurePlans;
/**
* @return Indicates whether user has read access to the billing profile.
*
*/
private Boolean hasReadAccess;
/**
* @return Identifies the billing profile that is linked to another billing profile in indirect purchase motion.
*
*/
private @Nullable BillingProfilePropertiesResponseIndirectRelationshipInfo indirectRelationshipInfo;
/**
* @return The day of the month when the invoice for the billing profile is generated.
*
*/
private Integer invoiceDay;
/**
* @return Flag controlling whether the invoices for the billing profile are sent through email.
*
*/
private @Nullable Boolean invoiceEmailOptIn;
/**
* @return The list of email addresses to receive invoices by email for the billing profile.
*
*/
private @Nullable List invoiceRecipients;
/**
* @return The other payment terms of the billing profile.
*
*/
private List otherPaymentTerms;
/**
* @return The default purchase order number that will appear on the invoices generated for the billing profile.
*
*/
private @Nullable String poNumber;
/**
* @return The provisioning state of the resource during a long-running operation.
*
*/
private String provisioningState;
/**
* @return The default address where the products are shipped, or the services are being used. If a ship to is not specified for a product or a subscription, then this address will be used.
*
*/
private @Nullable BillingProfilePropertiesResponseShipTo shipTo;
/**
* @return The address of the individual or organization that is responsible for the billing account.
*
*/
private @Nullable BillingProfilePropertiesResponseSoldTo soldTo;
/**
* @return The billing profile spending limit.
*
*/
private String spendingLimit;
/**
* @return The details of billing profile spending limit.
*
*/
private List spendingLimitDetails;
/**
* @return The status of the billing profile.
*
*/
private String status;
/**
* @return Reason for the specified billing profile status.
*
*/
private String statusReasonCode;
/**
* @return The system generated unique identifier for a billing profile.
*
*/
private String systemId;
/**
* @return Dictionary of metadata associated with the resource. Maximum key/value length supported of 256 characters. Keys/value should not empty value nor null. Keys can not contain < > % & \ ? /
*
*/
private @Nullable Map tags;
/**
* @return Identifies the cloud environments that are associated with a billing profile. This is a system managed optional field and gets updated as the billing profile gets associated with accounts in various clouds.
*
*/
private List targetClouds;
private BillingProfilePropertiesResponse() {}
/**
* @return Billing address.
*
*/
public Optional billTo() {
return Optional.ofNullable(this.billTo);
}
/**
* @return Identifies the billing relationship represented by the billing profile. The billing relationship may be between Microsoft, the customer, and/or a third-party.
*
*/
public String billingRelationshipType() {
return this.billingRelationshipType;
}
/**
* @return The currency in which the charges for the billing profile are billed.
*
*/
public String currency() {
return this.currency;
}
/**
* @return The current payment term of the billing profile.
*
*/
public Optional currentPaymentTerm() {
return Optional.ofNullable(this.currentPaymentTerm);
}
/**
* @return The name of the billing profile.
*
*/
public Optional displayName() {
return Optional.ofNullable(this.displayName);
}
/**
* @return Information about the enabled azure plans.
*
*/
public List enabledAzurePlans() {
return this.enabledAzurePlans == null ? List.of() : this.enabledAzurePlans;
}
/**
* @return Indicates whether user has read access to the billing profile.
*
*/
public Boolean hasReadAccess() {
return this.hasReadAccess;
}
/**
* @return Identifies the billing profile that is linked to another billing profile in indirect purchase motion.
*
*/
public Optional indirectRelationshipInfo() {
return Optional.ofNullable(this.indirectRelationshipInfo);
}
/**
* @return The day of the month when the invoice for the billing profile is generated.
*
*/
public Integer invoiceDay() {
return this.invoiceDay;
}
/**
* @return Flag controlling whether the invoices for the billing profile are sent through email.
*
*/
public Optional invoiceEmailOptIn() {
return Optional.ofNullable(this.invoiceEmailOptIn);
}
/**
* @return The list of email addresses to receive invoices by email for the billing profile.
*
*/
public List invoiceRecipients() {
return this.invoiceRecipients == null ? List.of() : this.invoiceRecipients;
}
/**
* @return The other payment terms of the billing profile.
*
*/
public List otherPaymentTerms() {
return this.otherPaymentTerms;
}
/**
* @return The default purchase order number that will appear on the invoices generated for the billing profile.
*
*/
public Optional poNumber() {
return Optional.ofNullable(this.poNumber);
}
/**
* @return The provisioning state of the resource during a long-running operation.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The default address where the products are shipped, or the services are being used. If a ship to is not specified for a product or a subscription, then this address will be used.
*
*/
public Optional shipTo() {
return Optional.ofNullable(this.shipTo);
}
/**
* @return The address of the individual or organization that is responsible for the billing account.
*
*/
public Optional soldTo() {
return Optional.ofNullable(this.soldTo);
}
/**
* @return The billing profile spending limit.
*
*/
public String spendingLimit() {
return this.spendingLimit;
}
/**
* @return The details of billing profile spending limit.
*
*/
public List spendingLimitDetails() {
return this.spendingLimitDetails;
}
/**
* @return The status of the billing profile.
*
*/
public String status() {
return this.status;
}
/**
* @return Reason for the specified billing profile status.
*
*/
public String statusReasonCode() {
return this.statusReasonCode;
}
/**
* @return The system generated unique identifier for a billing profile.
*
*/
public String systemId() {
return this.systemId;
}
/**
* @return Dictionary of metadata associated with the resource. Maximum key/value length supported of 256 characters. Keys/value should not empty value nor null. Keys can not contain < > % & \ ? /
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Identifies the cloud environments that are associated with a billing profile. This is a system managed optional field and gets updated as the billing profile gets associated with accounts in various clouds.
*
*/
public List targetClouds() {
return this.targetClouds;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(BillingProfilePropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable BillingProfilePropertiesResponseBillTo billTo;
private String billingRelationshipType;
private String currency;
private @Nullable BillingProfilePropertiesResponseCurrentPaymentTerm currentPaymentTerm;
private @Nullable String displayName;
private @Nullable List enabledAzurePlans;
private Boolean hasReadAccess;
private @Nullable BillingProfilePropertiesResponseIndirectRelationshipInfo indirectRelationshipInfo;
private Integer invoiceDay;
private @Nullable Boolean invoiceEmailOptIn;
private @Nullable List invoiceRecipients;
private List otherPaymentTerms;
private @Nullable String poNumber;
private String provisioningState;
private @Nullable BillingProfilePropertiesResponseShipTo shipTo;
private @Nullable BillingProfilePropertiesResponseSoldTo soldTo;
private String spendingLimit;
private List spendingLimitDetails;
private String status;
private String statusReasonCode;
private String systemId;
private @Nullable Map tags;
private List targetClouds;
public Builder() {}
public Builder(BillingProfilePropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.billTo = defaults.billTo;
this.billingRelationshipType = defaults.billingRelationshipType;
this.currency = defaults.currency;
this.currentPaymentTerm = defaults.currentPaymentTerm;
this.displayName = defaults.displayName;
this.enabledAzurePlans = defaults.enabledAzurePlans;
this.hasReadAccess = defaults.hasReadAccess;
this.indirectRelationshipInfo = defaults.indirectRelationshipInfo;
this.invoiceDay = defaults.invoiceDay;
this.invoiceEmailOptIn = defaults.invoiceEmailOptIn;
this.invoiceRecipients = defaults.invoiceRecipients;
this.otherPaymentTerms = defaults.otherPaymentTerms;
this.poNumber = defaults.poNumber;
this.provisioningState = defaults.provisioningState;
this.shipTo = defaults.shipTo;
this.soldTo = defaults.soldTo;
this.spendingLimit = defaults.spendingLimit;
this.spendingLimitDetails = defaults.spendingLimitDetails;
this.status = defaults.status;
this.statusReasonCode = defaults.statusReasonCode;
this.systemId = defaults.systemId;
this.tags = defaults.tags;
this.targetClouds = defaults.targetClouds;
}
@CustomType.Setter
public Builder billTo(@Nullable BillingProfilePropertiesResponseBillTo billTo) {
this.billTo = billTo;
return this;
}
@CustomType.Setter
public Builder billingRelationshipType(String billingRelationshipType) {
if (billingRelationshipType == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "billingRelationshipType");
}
this.billingRelationshipType = billingRelationshipType;
return this;
}
@CustomType.Setter
public Builder currency(String currency) {
if (currency == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "currency");
}
this.currency = currency;
return this;
}
@CustomType.Setter
public Builder currentPaymentTerm(@Nullable BillingProfilePropertiesResponseCurrentPaymentTerm currentPaymentTerm) {
this.currentPaymentTerm = currentPaymentTerm;
return this;
}
@CustomType.Setter
public Builder displayName(@Nullable String displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder enabledAzurePlans(@Nullable List enabledAzurePlans) {
this.enabledAzurePlans = enabledAzurePlans;
return this;
}
public Builder enabledAzurePlans(AzurePlanResponse... enabledAzurePlans) {
return enabledAzurePlans(List.of(enabledAzurePlans));
}
@CustomType.Setter
public Builder hasReadAccess(Boolean hasReadAccess) {
if (hasReadAccess == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "hasReadAccess");
}
this.hasReadAccess = hasReadAccess;
return this;
}
@CustomType.Setter
public Builder indirectRelationshipInfo(@Nullable BillingProfilePropertiesResponseIndirectRelationshipInfo indirectRelationshipInfo) {
this.indirectRelationshipInfo = indirectRelationshipInfo;
return this;
}
@CustomType.Setter
public Builder invoiceDay(Integer invoiceDay) {
if (invoiceDay == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "invoiceDay");
}
this.invoiceDay = invoiceDay;
return this;
}
@CustomType.Setter
public Builder invoiceEmailOptIn(@Nullable Boolean invoiceEmailOptIn) {
this.invoiceEmailOptIn = invoiceEmailOptIn;
return this;
}
@CustomType.Setter
public Builder invoiceRecipients(@Nullable List invoiceRecipients) {
this.invoiceRecipients = invoiceRecipients;
return this;
}
public Builder invoiceRecipients(String... invoiceRecipients) {
return invoiceRecipients(List.of(invoiceRecipients));
}
@CustomType.Setter
public Builder otherPaymentTerms(List otherPaymentTerms) {
if (otherPaymentTerms == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "otherPaymentTerms");
}
this.otherPaymentTerms = otherPaymentTerms;
return this;
}
public Builder otherPaymentTerms(PaymentTermResponse... otherPaymentTerms) {
return otherPaymentTerms(List.of(otherPaymentTerms));
}
@CustomType.Setter
public Builder poNumber(@Nullable String poNumber) {
this.poNumber = poNumber;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder shipTo(@Nullable BillingProfilePropertiesResponseShipTo shipTo) {
this.shipTo = shipTo;
return this;
}
@CustomType.Setter
public Builder soldTo(@Nullable BillingProfilePropertiesResponseSoldTo soldTo) {
this.soldTo = soldTo;
return this;
}
@CustomType.Setter
public Builder spendingLimit(String spendingLimit) {
if (spendingLimit == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "spendingLimit");
}
this.spendingLimit = spendingLimit;
return this;
}
@CustomType.Setter
public Builder spendingLimitDetails(List spendingLimitDetails) {
if (spendingLimitDetails == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "spendingLimitDetails");
}
this.spendingLimitDetails = spendingLimitDetails;
return this;
}
public Builder spendingLimitDetails(SpendingLimitDetailsResponse... spendingLimitDetails) {
return spendingLimitDetails(List.of(spendingLimitDetails));
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder statusReasonCode(String statusReasonCode) {
if (statusReasonCode == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "statusReasonCode");
}
this.statusReasonCode = statusReasonCode;
return this;
}
@CustomType.Setter
public Builder systemId(String systemId) {
if (systemId == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "systemId");
}
this.systemId = systemId;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder targetClouds(List targetClouds) {
if (targetClouds == null) {
throw new MissingRequiredPropertyException("BillingProfilePropertiesResponse", "targetClouds");
}
this.targetClouds = targetClouds;
return this;
}
public Builder targetClouds(String... targetClouds) {
return targetClouds(List.of(targetClouds));
}
public BillingProfilePropertiesResponse build() {
final var _resultValue = new BillingProfilePropertiesResponse();
_resultValue.billTo = billTo;
_resultValue.billingRelationshipType = billingRelationshipType;
_resultValue.currency = currency;
_resultValue.currentPaymentTerm = currentPaymentTerm;
_resultValue.displayName = displayName;
_resultValue.enabledAzurePlans = enabledAzurePlans;
_resultValue.hasReadAccess = hasReadAccess;
_resultValue.indirectRelationshipInfo = indirectRelationshipInfo;
_resultValue.invoiceDay = invoiceDay;
_resultValue.invoiceEmailOptIn = invoiceEmailOptIn;
_resultValue.invoiceRecipients = invoiceRecipients;
_resultValue.otherPaymentTerms = otherPaymentTerms;
_resultValue.poNumber = poNumber;
_resultValue.provisioningState = provisioningState;
_resultValue.shipTo = shipTo;
_resultValue.soldTo = soldTo;
_resultValue.spendingLimit = spendingLimit;
_resultValue.spendingLimitDetails = spendingLimitDetails;
_resultValue.status = status;
_resultValue.statusReasonCode = statusReasonCode;
_resultValue.systemId = systemId;
_resultValue.tags = tags;
_resultValue.targetClouds = targetClouds;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy