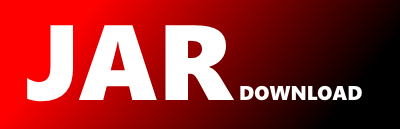
com.pulumi.azurenative.botservice.outputs.SlackChannelPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.botservice.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SlackChannelPropertiesResponse {
/**
* @return The Slack client id
*
*/
private @Nullable String clientId;
/**
* @return The Slack client secret. Value only returned through POST to the action Channel List API, otherwise empty.
*
*/
private @Nullable String clientSecret;
/**
* @return Whether this channel is enabled for the bot
*
*/
private Boolean isEnabled;
/**
* @return Whether this channel is validated for the bot
*
*/
private Boolean isValidated;
/**
* @return The Slack landing page Url
*
*/
private @Nullable String landingPageUrl;
/**
* @return The Sms auth token
*
*/
private String lastSubmissionId;
/**
* @return The Slack redirect action
*
*/
private String redirectAction;
/**
* @return Whether to register the settings before OAuth validation is performed. Recommended to True.
*
*/
private @Nullable Boolean registerBeforeOAuthFlow;
/**
* @return The Slack permission scopes.
*
*/
private @Nullable String scopes;
/**
* @return The Slack signing secret.
*
*/
private @Nullable String signingSecret;
/**
* @return The Slack verification token. Value only returned through POST to the action Channel List API, otherwise empty.
*
*/
private @Nullable String verificationToken;
private SlackChannelPropertiesResponse() {}
/**
* @return The Slack client id
*
*/
public Optional clientId() {
return Optional.ofNullable(this.clientId);
}
/**
* @return The Slack client secret. Value only returned through POST to the action Channel List API, otherwise empty.
*
*/
public Optional clientSecret() {
return Optional.ofNullable(this.clientSecret);
}
/**
* @return Whether this channel is enabled for the bot
*
*/
public Boolean isEnabled() {
return this.isEnabled;
}
/**
* @return Whether this channel is validated for the bot
*
*/
public Boolean isValidated() {
return this.isValidated;
}
/**
* @return The Slack landing page Url
*
*/
public Optional landingPageUrl() {
return Optional.ofNullable(this.landingPageUrl);
}
/**
* @return The Sms auth token
*
*/
public String lastSubmissionId() {
return this.lastSubmissionId;
}
/**
* @return The Slack redirect action
*
*/
public String redirectAction() {
return this.redirectAction;
}
/**
* @return Whether to register the settings before OAuth validation is performed. Recommended to True.
*
*/
public Optional registerBeforeOAuthFlow() {
return Optional.ofNullable(this.registerBeforeOAuthFlow);
}
/**
* @return The Slack permission scopes.
*
*/
public Optional scopes() {
return Optional.ofNullable(this.scopes);
}
/**
* @return The Slack signing secret.
*
*/
public Optional signingSecret() {
return Optional.ofNullable(this.signingSecret);
}
/**
* @return The Slack verification token. Value only returned through POST to the action Channel List API, otherwise empty.
*
*/
public Optional verificationToken() {
return Optional.ofNullable(this.verificationToken);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SlackChannelPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String clientId;
private @Nullable String clientSecret;
private Boolean isEnabled;
private Boolean isValidated;
private @Nullable String landingPageUrl;
private String lastSubmissionId;
private String redirectAction;
private @Nullable Boolean registerBeforeOAuthFlow;
private @Nullable String scopes;
private @Nullable String signingSecret;
private @Nullable String verificationToken;
public Builder() {}
public Builder(SlackChannelPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.clientId = defaults.clientId;
this.clientSecret = defaults.clientSecret;
this.isEnabled = defaults.isEnabled;
this.isValidated = defaults.isValidated;
this.landingPageUrl = defaults.landingPageUrl;
this.lastSubmissionId = defaults.lastSubmissionId;
this.redirectAction = defaults.redirectAction;
this.registerBeforeOAuthFlow = defaults.registerBeforeOAuthFlow;
this.scopes = defaults.scopes;
this.signingSecret = defaults.signingSecret;
this.verificationToken = defaults.verificationToken;
}
@CustomType.Setter
public Builder clientId(@Nullable String clientId) {
this.clientId = clientId;
return this;
}
@CustomType.Setter
public Builder clientSecret(@Nullable String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
@CustomType.Setter
public Builder isEnabled(Boolean isEnabled) {
if (isEnabled == null) {
throw new MissingRequiredPropertyException("SlackChannelPropertiesResponse", "isEnabled");
}
this.isEnabled = isEnabled;
return this;
}
@CustomType.Setter
public Builder isValidated(Boolean isValidated) {
if (isValidated == null) {
throw new MissingRequiredPropertyException("SlackChannelPropertiesResponse", "isValidated");
}
this.isValidated = isValidated;
return this;
}
@CustomType.Setter
public Builder landingPageUrl(@Nullable String landingPageUrl) {
this.landingPageUrl = landingPageUrl;
return this;
}
@CustomType.Setter
public Builder lastSubmissionId(String lastSubmissionId) {
if (lastSubmissionId == null) {
throw new MissingRequiredPropertyException("SlackChannelPropertiesResponse", "lastSubmissionId");
}
this.lastSubmissionId = lastSubmissionId;
return this;
}
@CustomType.Setter
public Builder redirectAction(String redirectAction) {
if (redirectAction == null) {
throw new MissingRequiredPropertyException("SlackChannelPropertiesResponse", "redirectAction");
}
this.redirectAction = redirectAction;
return this;
}
@CustomType.Setter
public Builder registerBeforeOAuthFlow(@Nullable Boolean registerBeforeOAuthFlow) {
this.registerBeforeOAuthFlow = registerBeforeOAuthFlow;
return this;
}
@CustomType.Setter
public Builder scopes(@Nullable String scopes) {
this.scopes = scopes;
return this;
}
@CustomType.Setter
public Builder signingSecret(@Nullable String signingSecret) {
this.signingSecret = signingSecret;
return this;
}
@CustomType.Setter
public Builder verificationToken(@Nullable String verificationToken) {
this.verificationToken = verificationToken;
return this;
}
public SlackChannelPropertiesResponse build() {
final var _resultValue = new SlackChannelPropertiesResponse();
_resultValue.clientId = clientId;
_resultValue.clientSecret = clientSecret;
_resultValue.isEnabled = isEnabled;
_resultValue.isValidated = isValidated;
_resultValue.landingPageUrl = landingPageUrl;
_resultValue.lastSubmissionId = lastSubmissionId;
_resultValue.redirectAction = redirectAction;
_resultValue.registerBeforeOAuthFlow = registerBeforeOAuthFlow;
_resultValue.scopes = scopes;
_resultValue.signingSecret = signingSecret;
_resultValue.verificationToken = verificationToken;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy