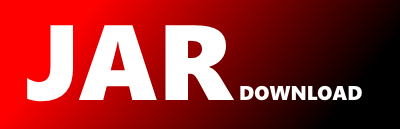
com.pulumi.azurenative.cache.CacheFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cache;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.cache.inputs.GetAccessPolicyArgs;
import com.pulumi.azurenative.cache.inputs.GetAccessPolicyAssignmentArgs;
import com.pulumi.azurenative.cache.inputs.GetAccessPolicyAssignmentPlainArgs;
import com.pulumi.azurenative.cache.inputs.GetAccessPolicyPlainArgs;
import com.pulumi.azurenative.cache.inputs.GetDatabaseArgs;
import com.pulumi.azurenative.cache.inputs.GetDatabasePlainArgs;
import com.pulumi.azurenative.cache.inputs.GetEnterprisePrivateEndpointConnectionArgs;
import com.pulumi.azurenative.cache.inputs.GetEnterprisePrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.cache.inputs.GetFirewallRuleArgs;
import com.pulumi.azurenative.cache.inputs.GetFirewallRulePlainArgs;
import com.pulumi.azurenative.cache.inputs.GetLinkedServerArgs;
import com.pulumi.azurenative.cache.inputs.GetLinkedServerPlainArgs;
import com.pulumi.azurenative.cache.inputs.GetPatchScheduleArgs;
import com.pulumi.azurenative.cache.inputs.GetPatchSchedulePlainArgs;
import com.pulumi.azurenative.cache.inputs.GetPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.cache.inputs.GetPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.cache.inputs.GetRedisArgs;
import com.pulumi.azurenative.cache.inputs.GetRedisEnterpriseArgs;
import com.pulumi.azurenative.cache.inputs.GetRedisEnterprisePlainArgs;
import com.pulumi.azurenative.cache.inputs.GetRedisPlainArgs;
import com.pulumi.azurenative.cache.inputs.ListDatabaseKeysArgs;
import com.pulumi.azurenative.cache.inputs.ListDatabaseKeysPlainArgs;
import com.pulumi.azurenative.cache.inputs.ListRedisKeysArgs;
import com.pulumi.azurenative.cache.inputs.ListRedisKeysPlainArgs;
import com.pulumi.azurenative.cache.outputs.GetAccessPolicyAssignmentResult;
import com.pulumi.azurenative.cache.outputs.GetAccessPolicyResult;
import com.pulumi.azurenative.cache.outputs.GetDatabaseResult;
import com.pulumi.azurenative.cache.outputs.GetEnterprisePrivateEndpointConnectionResult;
import com.pulumi.azurenative.cache.outputs.GetFirewallRuleResult;
import com.pulumi.azurenative.cache.outputs.GetLinkedServerResult;
import com.pulumi.azurenative.cache.outputs.GetPatchScheduleResult;
import com.pulumi.azurenative.cache.outputs.GetPrivateEndpointConnectionResult;
import com.pulumi.azurenative.cache.outputs.GetRedisEnterpriseResult;
import com.pulumi.azurenative.cache.outputs.GetRedisResult;
import com.pulumi.azurenative.cache.outputs.ListDatabaseKeysResult;
import com.pulumi.azurenative.cache.outputs.ListRedisKeysResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class CacheFunctions {
/**
* Gets the detailed information about an access policy of a redis cache
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getAccessPolicy(GetAccessPolicyArgs args) {
return getAccessPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the detailed information about an access policy of a redis cache
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getAccessPolicyPlain(GetAccessPolicyPlainArgs args) {
return getAccessPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the detailed information about an access policy of a redis cache
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getAccessPolicy(GetAccessPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:getAccessPolicy", TypeShape.of(GetAccessPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the detailed information about an access policy of a redis cache
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getAccessPolicyPlain(GetAccessPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:getAccessPolicy", TypeShape.of(GetAccessPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the list of assignments for an access policy of a redis cache
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2023-08-01, 2024-03-01, 2024-04-01-preview, 2024-09-01-preview.
*
*/
public static Output getAccessPolicyAssignment(GetAccessPolicyAssignmentArgs args) {
return getAccessPolicyAssignment(args, InvokeOptions.Empty);
}
/**
* Gets the list of assignments for an access policy of a redis cache
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2023-08-01, 2024-03-01, 2024-04-01-preview, 2024-09-01-preview.
*
*/
public static CompletableFuture getAccessPolicyAssignmentPlain(GetAccessPolicyAssignmentPlainArgs args) {
return getAccessPolicyAssignmentPlain(args, InvokeOptions.Empty);
}
/**
* Gets the list of assignments for an access policy of a redis cache
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2023-08-01, 2024-03-01, 2024-04-01-preview, 2024-09-01-preview.
*
*/
public static Output getAccessPolicyAssignment(GetAccessPolicyAssignmentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:getAccessPolicyAssignment", TypeShape.of(GetAccessPolicyAssignmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the list of assignments for an access policy of a redis cache
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2023-08-01, 2024-03-01, 2024-04-01-preview, 2024-09-01-preview.
*
*/
public static CompletableFuture getAccessPolicyAssignmentPlain(GetAccessPolicyAssignmentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:getAccessPolicyAssignment", TypeShape.of(GetAccessPolicyAssignmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about a database in a RedisEnterprise cluster.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static Output getDatabase(GetDatabaseArgs args) {
return getDatabase(args, InvokeOptions.Empty);
}
/**
* Gets information about a database in a RedisEnterprise cluster.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static CompletableFuture getDatabasePlain(GetDatabasePlainArgs args) {
return getDatabasePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about a database in a RedisEnterprise cluster.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static Output getDatabase(GetDatabaseArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:getDatabase", TypeShape.of(GetDatabaseResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about a database in a RedisEnterprise cluster.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static CompletableFuture getDatabasePlain(GetDatabasePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:getDatabase", TypeShape.of(GetDatabaseResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the RedisEnterprise cluster.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static Output getEnterprisePrivateEndpointConnection(GetEnterprisePrivateEndpointConnectionArgs args) {
return getEnterprisePrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the RedisEnterprise cluster.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static CompletableFuture getEnterprisePrivateEndpointConnectionPlain(GetEnterprisePrivateEndpointConnectionPlainArgs args) {
return getEnterprisePrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the RedisEnterprise cluster.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static Output getEnterprisePrivateEndpointConnection(GetEnterprisePrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:getEnterprisePrivateEndpointConnection", TypeShape.of(GetEnterprisePrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the RedisEnterprise cluster.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static CompletableFuture getEnterprisePrivateEndpointConnectionPlain(GetEnterprisePrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:getEnterprisePrivateEndpointConnection", TypeShape.of(GetEnterprisePrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a single firewall rule in a specified redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getFirewallRule(GetFirewallRuleArgs args) {
return getFirewallRule(args, InvokeOptions.Empty);
}
/**
* Gets a single firewall rule in a specified redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getFirewallRulePlain(GetFirewallRulePlainArgs args) {
return getFirewallRulePlain(args, InvokeOptions.Empty);
}
/**
* Gets a single firewall rule in a specified redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getFirewallRule(GetFirewallRuleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:getFirewallRule", TypeShape.of(GetFirewallRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a single firewall rule in a specified redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getFirewallRulePlain(GetFirewallRulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:getFirewallRule", TypeShape.of(GetFirewallRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the detailed information about a linked server of a redis cache (requires Premium SKU).
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getLinkedServer(GetLinkedServerArgs args) {
return getLinkedServer(args, InvokeOptions.Empty);
}
/**
* Gets the detailed information about a linked server of a redis cache (requires Premium SKU).
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getLinkedServerPlain(GetLinkedServerPlainArgs args) {
return getLinkedServerPlain(args, InvokeOptions.Empty);
}
/**
* Gets the detailed information about a linked server of a redis cache (requires Premium SKU).
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getLinkedServer(GetLinkedServerArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:getLinkedServer", TypeShape.of(GetLinkedServerResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the detailed information about a linked server of a redis cache (requires Premium SKU).
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getLinkedServerPlain(GetLinkedServerPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:getLinkedServer", TypeShape.of(GetLinkedServerResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the patching schedule of a redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getPatchSchedule(GetPatchScheduleArgs args) {
return getPatchSchedule(args, InvokeOptions.Empty);
}
/**
* Gets the patching schedule of a redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getPatchSchedulePlain(GetPatchSchedulePlainArgs args) {
return getPatchSchedulePlain(args, InvokeOptions.Empty);
}
/**
* Gets the patching schedule of a redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getPatchSchedule(GetPatchScheduleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:getPatchSchedule", TypeShape.of(GetPatchScheduleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the patching schedule of a redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getPatchSchedulePlain(GetPatchSchedulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:getPatchSchedule", TypeShape.of(GetPatchScheduleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args) {
return getPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args) {
return getPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the redis cache.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Redis cache (resource description).
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2015-08-01, 2017-02-01, 2019-07-01, 2020-06-01, 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getRedis(GetRedisArgs args) {
return getRedis(args, InvokeOptions.Empty);
}
/**
* Gets a Redis cache (resource description).
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2015-08-01, 2017-02-01, 2019-07-01, 2020-06-01, 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getRedisPlain(GetRedisPlainArgs args) {
return getRedisPlain(args, InvokeOptions.Empty);
}
/**
* Gets a Redis cache (resource description).
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2015-08-01, 2017-02-01, 2019-07-01, 2020-06-01, 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output getRedis(GetRedisArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:getRedis", TypeShape.of(GetRedisResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Redis cache (resource description).
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2015-08-01, 2017-02-01, 2019-07-01, 2020-06-01, 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture getRedisPlain(GetRedisPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:getRedis", TypeShape.of(GetRedisResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about a RedisEnterprise cluster
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2020-10-01-preview, 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static Output getRedisEnterprise(GetRedisEnterpriseArgs args) {
return getRedisEnterprise(args, InvokeOptions.Empty);
}
/**
* Gets information about a RedisEnterprise cluster
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2020-10-01-preview, 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static CompletableFuture getRedisEnterprisePlain(GetRedisEnterprisePlainArgs args) {
return getRedisEnterprisePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about a RedisEnterprise cluster
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2020-10-01-preview, 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static Output getRedisEnterprise(GetRedisEnterpriseArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:getRedisEnterprise", TypeShape.of(GetRedisEnterpriseResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about a RedisEnterprise cluster
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2020-10-01-preview, 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static CompletableFuture getRedisEnterprisePlain(GetRedisEnterprisePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:getRedisEnterprise", TypeShape.of(GetRedisEnterpriseResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the access keys for the RedisEnterprise database.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static Output listDatabaseKeys(ListDatabaseKeysArgs args) {
return listDatabaseKeys(args, InvokeOptions.Empty);
}
/**
* Retrieves the access keys for the RedisEnterprise database.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static CompletableFuture listDatabaseKeysPlain(ListDatabaseKeysPlainArgs args) {
return listDatabaseKeysPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the access keys for the RedisEnterprise database.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static Output listDatabaseKeys(ListDatabaseKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:listDatabaseKeys", TypeShape.of(ListDatabaseKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the access keys for the RedisEnterprise database.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-07-01, 2023-08-01-preview, 2023-10-01-preview, 2023-11-01, 2024-02-01, 2024-03-01-preview, 2024-06-01-preview, 2024-09-01-preview.
*
*/
public static CompletableFuture listDatabaseKeysPlain(ListDatabaseKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:listDatabaseKeys", TypeShape.of(ListDatabaseKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieve a Redis cache's access keys. This operation requires write permission to the cache resource.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2015-08-01, 2017-02-01, 2019-07-01, 2020-06-01, 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output listRedisKeys(ListRedisKeysArgs args) {
return listRedisKeys(args, InvokeOptions.Empty);
}
/**
* Retrieve a Redis cache's access keys. This operation requires write permission to the cache resource.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2015-08-01, 2017-02-01, 2019-07-01, 2020-06-01, 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture listRedisKeysPlain(ListRedisKeysPlainArgs args) {
return listRedisKeysPlain(args, InvokeOptions.Empty);
}
/**
* Retrieve a Redis cache's access keys. This operation requires write permission to the cache resource.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2015-08-01, 2017-02-01, 2019-07-01, 2020-06-01, 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static Output listRedisKeys(ListRedisKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cache:listRedisKeys", TypeShape.of(ListRedisKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieve a Redis cache's access keys. This operation requires write permission to the cache resource.
* Azure REST API version: 2023-04-01.
*
* Other available API versions: 2015-08-01, 2017-02-01, 2019-07-01, 2020-06-01, 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
*/
public static CompletableFuture listRedisKeysPlain(ListRedisKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cache:listRedisKeys", TypeShape.of(ListRedisKeysResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy