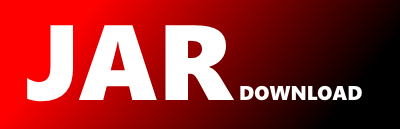
com.pulumi.azurenative.cdn.outputs.GetEndpointResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cdn.outputs;
import com.pulumi.azurenative.cdn.outputs.DeepCreatedCustomDomainResponse;
import com.pulumi.azurenative.cdn.outputs.DeepCreatedOriginGroupResponse;
import com.pulumi.azurenative.cdn.outputs.DeepCreatedOriginResponse;
import com.pulumi.azurenative.cdn.outputs.EndpointPropertiesUpdateParametersResponseDeliveryPolicy;
import com.pulumi.azurenative.cdn.outputs.EndpointPropertiesUpdateParametersResponseWebApplicationFirewallPolicyLink;
import com.pulumi.azurenative.cdn.outputs.GeoFilterResponse;
import com.pulumi.azurenative.cdn.outputs.ResourceReferenceResponse;
import com.pulumi.azurenative.cdn.outputs.SystemDataResponse;
import com.pulumi.azurenative.cdn.outputs.UrlSigningKeyResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetEndpointResult {
/**
* @return List of content types on which compression applies. The value should be a valid MIME type.
*
*/
private @Nullable List contentTypesToCompress;
/**
* @return The custom domains under the endpoint.
*
*/
private List customDomains;
/**
* @return A reference to the origin group.
*
*/
private @Nullable ResourceReferenceResponse defaultOriginGroup;
/**
* @return A policy that specifies the delivery rules to be used for an endpoint.
*
*/
private @Nullable EndpointPropertiesUpdateParametersResponseDeliveryPolicy deliveryPolicy;
/**
* @return List of rules defining the user's geo access within a CDN endpoint. Each geo filter defines an access rule to a specified path or content, e.g. block APAC for path /pictures/
*
*/
private @Nullable List geoFilters;
/**
* @return The host name of the endpoint structured as {endpointName}.{DNSZone}, e.g. contoso.azureedge.net
*
*/
private String hostName;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return Indicates whether content compression is enabled on CDN. Default value is false. If compression is enabled, content will be served as compressed if user requests for a compressed version. Content won't be compressed on CDN when requested content is smaller than 1 byte or larger than 1 MB.
*
*/
private @Nullable Boolean isCompressionEnabled;
/**
* @return Indicates whether HTTP traffic is allowed on the endpoint. Default value is true. At least one protocol (HTTP or HTTPS) must be allowed.
*
*/
private @Nullable Boolean isHttpAllowed;
/**
* @return Indicates whether HTTPS traffic is allowed on the endpoint. Default value is true. At least one protocol (HTTP or HTTPS) must be allowed.
*
*/
private @Nullable Boolean isHttpsAllowed;
/**
* @return Resource location.
*
*/
private String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Specifies what scenario the customer wants this CDN endpoint to optimize for, e.g. Download, Media services. With this information, CDN can apply scenario driven optimization.
*
*/
private @Nullable String optimizationType;
/**
* @return The origin groups comprising of origins that are used for load balancing the traffic based on availability.
*
*/
private @Nullable List originGroups;
/**
* @return The host header value sent to the origin with each request. This property at Endpoint is only allowed when endpoint uses single origin and can be overridden by the same property specified at origin.If you leave this blank, the request hostname determines this value. Azure CDN origins, such as Web Apps, Blob Storage, and Cloud Services require this host header value to match the origin hostname by default.
*
*/
private @Nullable String originHostHeader;
/**
* @return A directory path on the origin that CDN can use to retrieve content from, e.g. contoso.cloudapp.net/originpath.
*
*/
private @Nullable String originPath;
/**
* @return The source of the content being delivered via CDN.
*
*/
private List origins;
/**
* @return Path to a file hosted on the origin which helps accelerate delivery of the dynamic content and calculate the most optimal routes for the CDN. This is relative to the origin path. This property is only relevant when using a single origin.
*
*/
private @Nullable String probePath;
/**
* @return Provisioning status of the endpoint.
*
*/
private String provisioningState;
/**
* @return Defines how CDN caches requests that include query strings. You can ignore any query strings when caching, bypass caching to prevent requests that contain query strings from being cached, or cache every request with a unique URL.
*
*/
private @Nullable String queryStringCachingBehavior;
/**
* @return Resource status of the endpoint.
*
*/
private String resourceState;
/**
* @return Read only system data
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return List of keys used to validate the signed URL hashes.
*
*/
private @Nullable List urlSigningKeys;
/**
* @return Defines the Web Application Firewall policy for the endpoint (if applicable)
*
*/
private @Nullable EndpointPropertiesUpdateParametersResponseWebApplicationFirewallPolicyLink webApplicationFirewallPolicyLink;
private GetEndpointResult() {}
/**
* @return List of content types on which compression applies. The value should be a valid MIME type.
*
*/
public List contentTypesToCompress() {
return this.contentTypesToCompress == null ? List.of() : this.contentTypesToCompress;
}
/**
* @return The custom domains under the endpoint.
*
*/
public List customDomains() {
return this.customDomains;
}
/**
* @return A reference to the origin group.
*
*/
public Optional defaultOriginGroup() {
return Optional.ofNullable(this.defaultOriginGroup);
}
/**
* @return A policy that specifies the delivery rules to be used for an endpoint.
*
*/
public Optional deliveryPolicy() {
return Optional.ofNullable(this.deliveryPolicy);
}
/**
* @return List of rules defining the user's geo access within a CDN endpoint. Each geo filter defines an access rule to a specified path or content, e.g. block APAC for path /pictures/
*
*/
public List geoFilters() {
return this.geoFilters == null ? List.of() : this.geoFilters;
}
/**
* @return The host name of the endpoint structured as {endpointName}.{DNSZone}, e.g. contoso.azureedge.net
*
*/
public String hostName() {
return this.hostName;
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return Indicates whether content compression is enabled on CDN. Default value is false. If compression is enabled, content will be served as compressed if user requests for a compressed version. Content won't be compressed on CDN when requested content is smaller than 1 byte or larger than 1 MB.
*
*/
public Optional isCompressionEnabled() {
return Optional.ofNullable(this.isCompressionEnabled);
}
/**
* @return Indicates whether HTTP traffic is allowed on the endpoint. Default value is true. At least one protocol (HTTP or HTTPS) must be allowed.
*
*/
public Optional isHttpAllowed() {
return Optional.ofNullable(this.isHttpAllowed);
}
/**
* @return Indicates whether HTTPS traffic is allowed on the endpoint. Default value is true. At least one protocol (HTTP or HTTPS) must be allowed.
*
*/
public Optional isHttpsAllowed() {
return Optional.ofNullable(this.isHttpsAllowed);
}
/**
* @return Resource location.
*
*/
public String location() {
return this.location;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Specifies what scenario the customer wants this CDN endpoint to optimize for, e.g. Download, Media services. With this information, CDN can apply scenario driven optimization.
*
*/
public Optional optimizationType() {
return Optional.ofNullable(this.optimizationType);
}
/**
* @return The origin groups comprising of origins that are used for load balancing the traffic based on availability.
*
*/
public List originGroups() {
return this.originGroups == null ? List.of() : this.originGroups;
}
/**
* @return The host header value sent to the origin with each request. This property at Endpoint is only allowed when endpoint uses single origin and can be overridden by the same property specified at origin.If you leave this blank, the request hostname determines this value. Azure CDN origins, such as Web Apps, Blob Storage, and Cloud Services require this host header value to match the origin hostname by default.
*
*/
public Optional originHostHeader() {
return Optional.ofNullable(this.originHostHeader);
}
/**
* @return A directory path on the origin that CDN can use to retrieve content from, e.g. contoso.cloudapp.net/originpath.
*
*/
public Optional originPath() {
return Optional.ofNullable(this.originPath);
}
/**
* @return The source of the content being delivered via CDN.
*
*/
public List origins() {
return this.origins;
}
/**
* @return Path to a file hosted on the origin which helps accelerate delivery of the dynamic content and calculate the most optimal routes for the CDN. This is relative to the origin path. This property is only relevant when using a single origin.
*
*/
public Optional probePath() {
return Optional.ofNullable(this.probePath);
}
/**
* @return Provisioning status of the endpoint.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Defines how CDN caches requests that include query strings. You can ignore any query strings when caching, bypass caching to prevent requests that contain query strings from being cached, or cache every request with a unique URL.
*
*/
public Optional queryStringCachingBehavior() {
return Optional.ofNullable(this.queryStringCachingBehavior);
}
/**
* @return Resource status of the endpoint.
*
*/
public String resourceState() {
return this.resourceState;
}
/**
* @return Read only system data
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return List of keys used to validate the signed URL hashes.
*
*/
public List urlSigningKeys() {
return this.urlSigningKeys == null ? List.of() : this.urlSigningKeys;
}
/**
* @return Defines the Web Application Firewall policy for the endpoint (if applicable)
*
*/
public Optional webApplicationFirewallPolicyLink() {
return Optional.ofNullable(this.webApplicationFirewallPolicyLink);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetEndpointResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List contentTypesToCompress;
private List customDomains;
private @Nullable ResourceReferenceResponse defaultOriginGroup;
private @Nullable EndpointPropertiesUpdateParametersResponseDeliveryPolicy deliveryPolicy;
private @Nullable List geoFilters;
private String hostName;
private String id;
private @Nullable Boolean isCompressionEnabled;
private @Nullable Boolean isHttpAllowed;
private @Nullable Boolean isHttpsAllowed;
private String location;
private String name;
private @Nullable String optimizationType;
private @Nullable List originGroups;
private @Nullable String originHostHeader;
private @Nullable String originPath;
private List origins;
private @Nullable String probePath;
private String provisioningState;
private @Nullable String queryStringCachingBehavior;
private String resourceState;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
private @Nullable List urlSigningKeys;
private @Nullable EndpointPropertiesUpdateParametersResponseWebApplicationFirewallPolicyLink webApplicationFirewallPolicyLink;
public Builder() {}
public Builder(GetEndpointResult defaults) {
Objects.requireNonNull(defaults);
this.contentTypesToCompress = defaults.contentTypesToCompress;
this.customDomains = defaults.customDomains;
this.defaultOriginGroup = defaults.defaultOriginGroup;
this.deliveryPolicy = defaults.deliveryPolicy;
this.geoFilters = defaults.geoFilters;
this.hostName = defaults.hostName;
this.id = defaults.id;
this.isCompressionEnabled = defaults.isCompressionEnabled;
this.isHttpAllowed = defaults.isHttpAllowed;
this.isHttpsAllowed = defaults.isHttpsAllowed;
this.location = defaults.location;
this.name = defaults.name;
this.optimizationType = defaults.optimizationType;
this.originGroups = defaults.originGroups;
this.originHostHeader = defaults.originHostHeader;
this.originPath = defaults.originPath;
this.origins = defaults.origins;
this.probePath = defaults.probePath;
this.provisioningState = defaults.provisioningState;
this.queryStringCachingBehavior = defaults.queryStringCachingBehavior;
this.resourceState = defaults.resourceState;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
this.urlSigningKeys = defaults.urlSigningKeys;
this.webApplicationFirewallPolicyLink = defaults.webApplicationFirewallPolicyLink;
}
@CustomType.Setter
public Builder contentTypesToCompress(@Nullable List contentTypesToCompress) {
this.contentTypesToCompress = contentTypesToCompress;
return this;
}
public Builder contentTypesToCompress(String... contentTypesToCompress) {
return contentTypesToCompress(List.of(contentTypesToCompress));
}
@CustomType.Setter
public Builder customDomains(List customDomains) {
if (customDomains == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "customDomains");
}
this.customDomains = customDomains;
return this;
}
public Builder customDomains(DeepCreatedCustomDomainResponse... customDomains) {
return customDomains(List.of(customDomains));
}
@CustomType.Setter
public Builder defaultOriginGroup(@Nullable ResourceReferenceResponse defaultOriginGroup) {
this.defaultOriginGroup = defaultOriginGroup;
return this;
}
@CustomType.Setter
public Builder deliveryPolicy(@Nullable EndpointPropertiesUpdateParametersResponseDeliveryPolicy deliveryPolicy) {
this.deliveryPolicy = deliveryPolicy;
return this;
}
@CustomType.Setter
public Builder geoFilters(@Nullable List geoFilters) {
this.geoFilters = geoFilters;
return this;
}
public Builder geoFilters(GeoFilterResponse... geoFilters) {
return geoFilters(List.of(geoFilters));
}
@CustomType.Setter
public Builder hostName(String hostName) {
if (hostName == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "hostName");
}
this.hostName = hostName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isCompressionEnabled(@Nullable Boolean isCompressionEnabled) {
this.isCompressionEnabled = isCompressionEnabled;
return this;
}
@CustomType.Setter
public Builder isHttpAllowed(@Nullable Boolean isHttpAllowed) {
this.isHttpAllowed = isHttpAllowed;
return this;
}
@CustomType.Setter
public Builder isHttpsAllowed(@Nullable Boolean isHttpsAllowed) {
this.isHttpsAllowed = isHttpsAllowed;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder optimizationType(@Nullable String optimizationType) {
this.optimizationType = optimizationType;
return this;
}
@CustomType.Setter
public Builder originGroups(@Nullable List originGroups) {
this.originGroups = originGroups;
return this;
}
public Builder originGroups(DeepCreatedOriginGroupResponse... originGroups) {
return originGroups(List.of(originGroups));
}
@CustomType.Setter
public Builder originHostHeader(@Nullable String originHostHeader) {
this.originHostHeader = originHostHeader;
return this;
}
@CustomType.Setter
public Builder originPath(@Nullable String originPath) {
this.originPath = originPath;
return this;
}
@CustomType.Setter
public Builder origins(List origins) {
if (origins == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "origins");
}
this.origins = origins;
return this;
}
public Builder origins(DeepCreatedOriginResponse... origins) {
return origins(List.of(origins));
}
@CustomType.Setter
public Builder probePath(@Nullable String probePath) {
this.probePath = probePath;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder queryStringCachingBehavior(@Nullable String queryStringCachingBehavior) {
this.queryStringCachingBehavior = queryStringCachingBehavior;
return this;
}
@CustomType.Setter
public Builder resourceState(String resourceState) {
if (resourceState == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "resourceState");
}
this.resourceState = resourceState;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder urlSigningKeys(@Nullable List urlSigningKeys) {
this.urlSigningKeys = urlSigningKeys;
return this;
}
public Builder urlSigningKeys(UrlSigningKeyResponse... urlSigningKeys) {
return urlSigningKeys(List.of(urlSigningKeys));
}
@CustomType.Setter
public Builder webApplicationFirewallPolicyLink(@Nullable EndpointPropertiesUpdateParametersResponseWebApplicationFirewallPolicyLink webApplicationFirewallPolicyLink) {
this.webApplicationFirewallPolicyLink = webApplicationFirewallPolicyLink;
return this;
}
public GetEndpointResult build() {
final var _resultValue = new GetEndpointResult();
_resultValue.contentTypesToCompress = contentTypesToCompress;
_resultValue.customDomains = customDomains;
_resultValue.defaultOriginGroup = defaultOriginGroup;
_resultValue.deliveryPolicy = deliveryPolicy;
_resultValue.geoFilters = geoFilters;
_resultValue.hostName = hostName;
_resultValue.id = id;
_resultValue.isCompressionEnabled = isCompressionEnabled;
_resultValue.isHttpAllowed = isHttpAllowed;
_resultValue.isHttpsAllowed = isHttpsAllowed;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.optimizationType = optimizationType;
_resultValue.originGroups = originGroups;
_resultValue.originHostHeader = originHostHeader;
_resultValue.originPath = originPath;
_resultValue.origins = origins;
_resultValue.probePath = probePath;
_resultValue.provisioningState = provisioningState;
_resultValue.queryStringCachingBehavior = queryStringCachingBehavior;
_resultValue.resourceState = resourceState;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.urlSigningKeys = urlSigningKeys;
_resultValue.webApplicationFirewallPolicyLink = webApplicationFirewallPolicyLink;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy