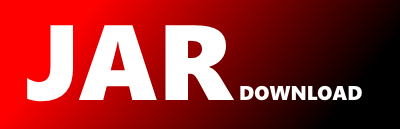
com.pulumi.azurenative.chaos.ChaosFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.chaos;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.chaos.inputs.GetCapabilityArgs;
import com.pulumi.azurenative.chaos.inputs.GetCapabilityPlainArgs;
import com.pulumi.azurenative.chaos.inputs.GetExperimentArgs;
import com.pulumi.azurenative.chaos.inputs.GetExperimentExecutionDetailsArgs;
import com.pulumi.azurenative.chaos.inputs.GetExperimentExecutionDetailsPlainArgs;
import com.pulumi.azurenative.chaos.inputs.GetExperimentPlainArgs;
import com.pulumi.azurenative.chaos.inputs.GetPrivateAccessArgs;
import com.pulumi.azurenative.chaos.inputs.GetPrivateAccessPlainArgs;
import com.pulumi.azurenative.chaos.inputs.GetTargetArgs;
import com.pulumi.azurenative.chaos.inputs.GetTargetPlainArgs;
import com.pulumi.azurenative.chaos.outputs.GetCapabilityResult;
import com.pulumi.azurenative.chaos.outputs.GetExperimentExecutionDetailsResult;
import com.pulumi.azurenative.chaos.outputs.GetExperimentResult;
import com.pulumi.azurenative.chaos.outputs.GetPrivateAccessResult;
import com.pulumi.azurenative.chaos.outputs.GetTargetResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class ChaosFunctions {
/**
* Get a Capability resource that extends a Target resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static Output getCapability(GetCapabilityArgs args) {
return getCapability(args, InvokeOptions.Empty);
}
/**
* Get a Capability resource that extends a Target resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static CompletableFuture getCapabilityPlain(GetCapabilityPlainArgs args) {
return getCapabilityPlain(args, InvokeOptions.Empty);
}
/**
* Get a Capability resource that extends a Target resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static Output getCapability(GetCapabilityArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:chaos:getCapability", TypeShape.of(GetCapabilityResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Capability resource that extends a Target resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static CompletableFuture getCapabilityPlain(GetCapabilityPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:chaos:getCapability", TypeShape.of(GetCapabilityResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Experiment resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static Output getExperiment(GetExperimentArgs args) {
return getExperiment(args, InvokeOptions.Empty);
}
/**
* Get a Experiment resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static CompletableFuture getExperimentPlain(GetExperimentPlainArgs args) {
return getExperimentPlain(args, InvokeOptions.Empty);
}
/**
* Get a Experiment resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static Output getExperiment(GetExperimentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:chaos:getExperiment", TypeShape.of(GetExperimentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Experiment resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static CompletableFuture getExperimentPlain(GetExperimentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:chaos:getExperiment", TypeShape.of(GetExperimentResult.class), args, Utilities.withVersion(options));
}
/**
* Execution details of an experiment resource.
* Azure REST API version: 2023-11-01.
*
* Other available API versions: 2024-01-01, 2024-03-22-preview.
*
*/
public static Output getExperimentExecutionDetails(GetExperimentExecutionDetailsArgs args) {
return getExperimentExecutionDetails(args, InvokeOptions.Empty);
}
/**
* Execution details of an experiment resource.
* Azure REST API version: 2023-11-01.
*
* Other available API versions: 2024-01-01, 2024-03-22-preview.
*
*/
public static CompletableFuture getExperimentExecutionDetailsPlain(GetExperimentExecutionDetailsPlainArgs args) {
return getExperimentExecutionDetailsPlain(args, InvokeOptions.Empty);
}
/**
* Execution details of an experiment resource.
* Azure REST API version: 2023-11-01.
*
* Other available API versions: 2024-01-01, 2024-03-22-preview.
*
*/
public static Output getExperimentExecutionDetails(GetExperimentExecutionDetailsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:chaos:getExperimentExecutionDetails", TypeShape.of(GetExperimentExecutionDetailsResult.class), args, Utilities.withVersion(options));
}
/**
* Execution details of an experiment resource.
* Azure REST API version: 2023-11-01.
*
* Other available API versions: 2024-01-01, 2024-03-22-preview.
*
*/
public static CompletableFuture getExperimentExecutionDetailsPlain(GetExperimentExecutionDetailsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:chaos:getExperimentExecutionDetails", TypeShape.of(GetExperimentExecutionDetailsResult.class), args, Utilities.withVersion(options));
}
/**
* Get a private access resource
* Azure REST API version: 2023-10-27-preview.
*
* Other available API versions: 2024-03-22-preview.
*
*/
public static Output getPrivateAccess(GetPrivateAccessArgs args) {
return getPrivateAccess(args, InvokeOptions.Empty);
}
/**
* Get a private access resource
* Azure REST API version: 2023-10-27-preview.
*
* Other available API versions: 2024-03-22-preview.
*
*/
public static CompletableFuture getPrivateAccessPlain(GetPrivateAccessPlainArgs args) {
return getPrivateAccessPlain(args, InvokeOptions.Empty);
}
/**
* Get a private access resource
* Azure REST API version: 2023-10-27-preview.
*
* Other available API versions: 2024-03-22-preview.
*
*/
public static Output getPrivateAccess(GetPrivateAccessArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:chaos:getPrivateAccess", TypeShape.of(GetPrivateAccessResult.class), args, Utilities.withVersion(options));
}
/**
* Get a private access resource
* Azure REST API version: 2023-10-27-preview.
*
* Other available API versions: 2024-03-22-preview.
*
*/
public static CompletableFuture getPrivateAccessPlain(GetPrivateAccessPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:chaos:getPrivateAccess", TypeShape.of(GetPrivateAccessResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Target resource that extends a tracked regional resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static Output getTarget(GetTargetArgs args) {
return getTarget(args, InvokeOptions.Empty);
}
/**
* Get a Target resource that extends a tracked regional resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static CompletableFuture getTargetPlain(GetTargetPlainArgs args) {
return getTargetPlain(args, InvokeOptions.Empty);
}
/**
* Get a Target resource that extends a tracked regional resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static Output getTarget(GetTargetArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:chaos:getTarget", TypeShape.of(GetTargetResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Target resource that extends a tracked regional resource.
* Azure REST API version: 2023-04-15-preview.
*
* Other available API versions: 2023-09-01-preview, 2023-10-27-preview, 2023-11-01, 2024-01-01, 2024-03-22-preview.
*
*/
public static CompletableFuture getTargetPlain(GetTargetPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:chaos:getTarget", TypeShape.of(GetTargetResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy