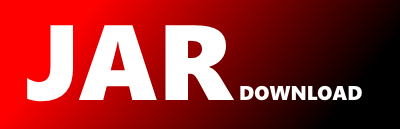
com.pulumi.azurenative.communication.CommunicationFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.communication;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.communication.inputs.GetCommunicationServiceArgs;
import com.pulumi.azurenative.communication.inputs.GetCommunicationServicePlainArgs;
import com.pulumi.azurenative.communication.inputs.GetDomainArgs;
import com.pulumi.azurenative.communication.inputs.GetDomainPlainArgs;
import com.pulumi.azurenative.communication.inputs.GetEmailServiceArgs;
import com.pulumi.azurenative.communication.inputs.GetEmailServicePlainArgs;
import com.pulumi.azurenative.communication.inputs.GetSenderUsernameArgs;
import com.pulumi.azurenative.communication.inputs.GetSenderUsernamePlainArgs;
import com.pulumi.azurenative.communication.inputs.GetSuppressionListAddressArgs;
import com.pulumi.azurenative.communication.inputs.GetSuppressionListAddressPlainArgs;
import com.pulumi.azurenative.communication.inputs.GetSuppressionListArgs;
import com.pulumi.azurenative.communication.inputs.GetSuppressionListPlainArgs;
import com.pulumi.azurenative.communication.inputs.ListCommunicationServiceKeysArgs;
import com.pulumi.azurenative.communication.inputs.ListCommunicationServiceKeysPlainArgs;
import com.pulumi.azurenative.communication.outputs.GetCommunicationServiceResult;
import com.pulumi.azurenative.communication.outputs.GetDomainResult;
import com.pulumi.azurenative.communication.outputs.GetEmailServiceResult;
import com.pulumi.azurenative.communication.outputs.GetSenderUsernameResult;
import com.pulumi.azurenative.communication.outputs.GetSuppressionListAddressResult;
import com.pulumi.azurenative.communication.outputs.GetSuppressionListResult;
import com.pulumi.azurenative.communication.outputs.ListCommunicationServiceKeysResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class CommunicationFunctions {
/**
* Get the CommunicationService and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static Output getCommunicationService(GetCommunicationServiceArgs args) {
return getCommunicationService(args, InvokeOptions.Empty);
}
/**
* Get the CommunicationService and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static CompletableFuture getCommunicationServicePlain(GetCommunicationServicePlainArgs args) {
return getCommunicationServicePlain(args, InvokeOptions.Empty);
}
/**
* Get the CommunicationService and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static Output getCommunicationService(GetCommunicationServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:communication:getCommunicationService", TypeShape.of(GetCommunicationServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get the CommunicationService and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static CompletableFuture getCommunicationServicePlain(GetCommunicationServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:communication:getCommunicationService", TypeShape.of(GetCommunicationServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get the Domains resource and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2022-07-01-preview, 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static Output getDomain(GetDomainArgs args) {
return getDomain(args, InvokeOptions.Empty);
}
/**
* Get the Domains resource and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2022-07-01-preview, 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static CompletableFuture getDomainPlain(GetDomainPlainArgs args) {
return getDomainPlain(args, InvokeOptions.Empty);
}
/**
* Get the Domains resource and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2022-07-01-preview, 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static Output getDomain(GetDomainArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:communication:getDomain", TypeShape.of(GetDomainResult.class), args, Utilities.withVersion(options));
}
/**
* Get the Domains resource and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2022-07-01-preview, 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static CompletableFuture getDomainPlain(GetDomainPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:communication:getDomain", TypeShape.of(GetDomainResult.class), args, Utilities.withVersion(options));
}
/**
* Get the EmailService and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static Output getEmailService(GetEmailServiceArgs args) {
return getEmailService(args, InvokeOptions.Empty);
}
/**
* Get the EmailService and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static CompletableFuture getEmailServicePlain(GetEmailServicePlainArgs args) {
return getEmailServicePlain(args, InvokeOptions.Empty);
}
/**
* Get the EmailService and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static Output getEmailService(GetEmailServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:communication:getEmailService", TypeShape.of(GetEmailServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get the EmailService and its properties.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static CompletableFuture getEmailServicePlain(GetEmailServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:communication:getEmailService", TypeShape.of(GetEmailServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a valid sender username for a domains resource.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static Output getSenderUsername(GetSenderUsernameArgs args) {
return getSenderUsername(args, InvokeOptions.Empty);
}
/**
* Get a valid sender username for a domains resource.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static CompletableFuture getSenderUsernamePlain(GetSenderUsernamePlainArgs args) {
return getSenderUsernamePlain(args, InvokeOptions.Empty);
}
/**
* Get a valid sender username for a domains resource.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static Output getSenderUsername(GetSenderUsernameArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:communication:getSenderUsername", TypeShape.of(GetSenderUsernameResult.class), args, Utilities.withVersion(options));
}
/**
* Get a valid sender username for a domains resource.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static CompletableFuture getSenderUsernamePlain(GetSenderUsernamePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:communication:getSenderUsername", TypeShape.of(GetSenderUsernameResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SuppressionList resource.
* Azure REST API version: 2023-06-01-preview.
*
*/
public static Output getSuppressionList(GetSuppressionListArgs args) {
return getSuppressionList(args, InvokeOptions.Empty);
}
/**
* Get a SuppressionList resource.
* Azure REST API version: 2023-06-01-preview.
*
*/
public static CompletableFuture getSuppressionListPlain(GetSuppressionListPlainArgs args) {
return getSuppressionListPlain(args, InvokeOptions.Empty);
}
/**
* Get a SuppressionList resource.
* Azure REST API version: 2023-06-01-preview.
*
*/
public static Output getSuppressionList(GetSuppressionListArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:communication:getSuppressionList", TypeShape.of(GetSuppressionListResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SuppressionList resource.
* Azure REST API version: 2023-06-01-preview.
*
*/
public static CompletableFuture getSuppressionListPlain(GetSuppressionListPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:communication:getSuppressionList", TypeShape.of(GetSuppressionListResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SuppressionListAddress.
* Azure REST API version: 2023-06-01-preview.
*
*/
public static Output getSuppressionListAddress(GetSuppressionListAddressArgs args) {
return getSuppressionListAddress(args, InvokeOptions.Empty);
}
/**
* Get a SuppressionListAddress.
* Azure REST API version: 2023-06-01-preview.
*
*/
public static CompletableFuture getSuppressionListAddressPlain(GetSuppressionListAddressPlainArgs args) {
return getSuppressionListAddressPlain(args, InvokeOptions.Empty);
}
/**
* Get a SuppressionListAddress.
* Azure REST API version: 2023-06-01-preview.
*
*/
public static Output getSuppressionListAddress(GetSuppressionListAddressArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:communication:getSuppressionListAddress", TypeShape.of(GetSuppressionListAddressResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SuppressionListAddress.
* Azure REST API version: 2023-06-01-preview.
*
*/
public static CompletableFuture getSuppressionListAddressPlain(GetSuppressionListAddressPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:communication:getSuppressionListAddress", TypeShape.of(GetSuppressionListAddressResult.class), args, Utilities.withVersion(options));
}
/**
* Get the access keys of the CommunicationService resource.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static Output listCommunicationServiceKeys(ListCommunicationServiceKeysArgs args) {
return listCommunicationServiceKeys(args, InvokeOptions.Empty);
}
/**
* Get the access keys of the CommunicationService resource.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static CompletableFuture listCommunicationServiceKeysPlain(ListCommunicationServiceKeysPlainArgs args) {
return listCommunicationServiceKeysPlain(args, InvokeOptions.Empty);
}
/**
* Get the access keys of the CommunicationService resource.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static Output listCommunicationServiceKeys(ListCommunicationServiceKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:communication:listCommunicationServiceKeys", TypeShape.of(ListCommunicationServiceKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Get the access keys of the CommunicationService resource.
* Azure REST API version: 2023-03-31.
*
* Other available API versions: 2023-04-01, 2023-04-01-preview, 2023-06-01-preview.
*
*/
public static CompletableFuture listCommunicationServiceKeysPlain(ListCommunicationServiceKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:communication:listCommunicationServiceKeys", TypeShape.of(ListCommunicationServiceKeysResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy