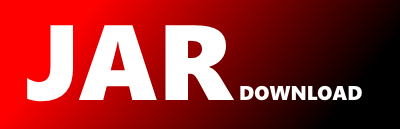
com.pulumi.azurenative.compute.inputs.VirtualMachinePublicIPAddressConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.inputs;
import com.pulumi.azurenative.compute.enums.DeleteOptions;
import com.pulumi.azurenative.compute.enums.IPVersions;
import com.pulumi.azurenative.compute.enums.PublicIPAllocationMethod;
import com.pulumi.azurenative.compute.inputs.PublicIPAddressSkuArgs;
import com.pulumi.azurenative.compute.inputs.SubResourceArgs;
import com.pulumi.azurenative.compute.inputs.VirtualMachineIpTagArgs;
import com.pulumi.azurenative.compute.inputs.VirtualMachinePublicIPAddressDnsSettingsConfigurationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes a virtual machines IP Configuration's PublicIPAddress configuration
*
*/
public final class VirtualMachinePublicIPAddressConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final VirtualMachinePublicIPAddressConfigurationArgs Empty = new VirtualMachinePublicIPAddressConfigurationArgs();
/**
* Specify what happens to the public IP address when the VM is deleted
*
*/
@Import(name="deleteOption")
private @Nullable Output> deleteOption;
/**
* @return Specify what happens to the public IP address when the VM is deleted
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy