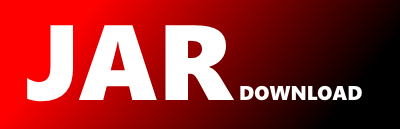
com.pulumi.azurenative.compute.outputs.VirtualMachineScaleSetExtensionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.KeyVaultSecretReferenceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VirtualMachineScaleSetExtensionResponse {
/**
* @return Indicates whether the extension should use a newer minor version if one is available at deployment time. Once deployed, however, the extension will not upgrade minor versions unless redeployed, even with this property set to true.
*
*/
private @Nullable Boolean autoUpgradeMinorVersion;
/**
* @return Indicates whether the extension should be automatically upgraded by the platform if there is a newer version of the extension available.
*
*/
private @Nullable Boolean enableAutomaticUpgrade;
/**
* @return If a value is provided and is different from the previous value, the extension handler will be forced to update even if the extension configuration has not changed.
*
*/
private @Nullable String forceUpdateTag;
/**
* @return Resource Id
*
*/
private String id;
/**
* @return The name of the extension.
*
*/
private @Nullable String name;
/**
* @return The extension can contain either protectedSettings or protectedSettingsFromKeyVault or no protected settings at all.
*
*/
private @Nullable Object protectedSettings;
/**
* @return The extensions protected settings that are passed by reference, and consumed from key vault
*
*/
private @Nullable KeyVaultSecretReferenceResponse protectedSettingsFromKeyVault;
/**
* @return Collection of extension names after which this extension needs to be provisioned.
*
*/
private @Nullable List provisionAfterExtensions;
/**
* @return The provisioning state, which only appears in the response.
*
*/
private String provisioningState;
/**
* @return The name of the extension handler publisher.
*
*/
private @Nullable String publisher;
/**
* @return Json formatted public settings for the extension.
*
*/
private @Nullable Object settings;
/**
* @return Indicates whether failures stemming from the extension will be suppressed (Operational failures such as not connecting to the VM will not be suppressed regardless of this value). The default is false.
*
*/
private @Nullable Boolean suppressFailures;
/**
* @return Resource type
*
*/
private String type;
/**
* @return Specifies the version of the script handler.
*
*/
private @Nullable String typeHandlerVersion;
private VirtualMachineScaleSetExtensionResponse() {}
/**
* @return Indicates whether the extension should use a newer minor version if one is available at deployment time. Once deployed, however, the extension will not upgrade minor versions unless redeployed, even with this property set to true.
*
*/
public Optional autoUpgradeMinorVersion() {
return Optional.ofNullable(this.autoUpgradeMinorVersion);
}
/**
* @return Indicates whether the extension should be automatically upgraded by the platform if there is a newer version of the extension available.
*
*/
public Optional enableAutomaticUpgrade() {
return Optional.ofNullable(this.enableAutomaticUpgrade);
}
/**
* @return If a value is provided and is different from the previous value, the extension handler will be forced to update even if the extension configuration has not changed.
*
*/
public Optional forceUpdateTag() {
return Optional.ofNullable(this.forceUpdateTag);
}
/**
* @return Resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the extension.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The extension can contain either protectedSettings or protectedSettingsFromKeyVault or no protected settings at all.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy