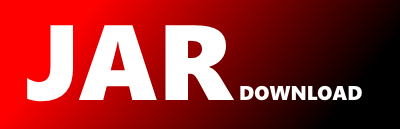
com.pulumi.azurenative.compute.outputs.VirtualMachineScaleSetOSProfileResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.LinuxConfigurationResponse;
import com.pulumi.azurenative.compute.outputs.VaultSecretGroupResponse;
import com.pulumi.azurenative.compute.outputs.WindowsConfigurationResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VirtualMachineScaleSetOSProfileResponse {
/**
* @return Specifies the password of the administrator account. <br><br> **Minimum-length (Windows):** 8 characters <br><br> **Minimum-length (Linux):** 6 characters <br><br> **Max-length (Windows):** 123 characters <br><br> **Max-length (Linux):** 72 characters <br><br> **Complexity requirements:** 3 out of 4 conditions below need to be fulfilled <br> Has lower characters <br>Has upper characters <br> Has a digit <br> Has a special character (Regex match [\W_]) <br><br> **Disallowed values:** "abc{@literal @}123", "P{@literal @}$$w0rd", "P{@literal @}ssw0rd", "P{@literal @}ssword123", "Pa$$word", "pass{@literal @}word1", "Password!", "Password1", "Password22", "iloveyou!" <br><br> For resetting the password, see [How to reset the Remote Desktop service or its login password in a Windows VM](https://docs.microsoft.com/troubleshoot/azure/virtual-machines/reset-rdp) <br><br> For resetting root password, see [Manage users, SSH, and check or repair disks on Azure Linux VMs using the VMAccess Extension](https://docs.microsoft.com/troubleshoot/azure/virtual-machines/troubleshoot-ssh-connection)
*
*/
private @Nullable String adminPassword;
/**
* @return Specifies the name of the administrator account. <br><br> **Windows-only restriction:** Cannot end in "." <br><br> **Disallowed values:** "administrator", "admin", "user", "user1", "test", "user2", "test1", "user3", "admin1", "1", "123", "a", "actuser", "adm", "admin2", "aspnet", "backup", "console", "david", "guest", "john", "owner", "root", "server", "sql", "support", "support_388945a0", "sys", "test2", "test3", "user4", "user5". <br><br> **Minimum-length (Linux):** 1 character <br><br> **Max-length (Linux):** 64 characters <br><br> **Max-length (Windows):** 20 characters
*
*/
private @Nullable String adminUsername;
/**
* @return Specifies whether extension operations should be allowed on the virtual machine scale set. This may only be set to False when no extensions are present on the virtual machine scale set.
*
*/
private @Nullable Boolean allowExtensionOperations;
/**
* @return Specifies the computer name prefix for all of the virtual machines in the scale set. Computer name prefixes must be 1 to 15 characters long.
*
*/
private @Nullable String computerNamePrefix;
/**
* @return Specifies a base-64 encoded string of custom data. The base-64 encoded string is decoded to a binary array that is saved as a file on the Virtual Machine. The maximum length of the binary array is 65535 bytes. For using cloud-init for your VM, see [Using cloud-init to customize a Linux VM during creation](https://docs.microsoft.com/azure/virtual-machines/linux/using-cloud-init)
*
*/
private @Nullable String customData;
/**
* @return Specifies the Linux operating system settings on the virtual machine. For a list of supported Linux distributions, see [Linux on Azure-Endorsed Distributions](https://docs.microsoft.com/azure/virtual-machines/linux/endorsed-distros).
*
*/
private @Nullable LinuxConfigurationResponse linuxConfiguration;
/**
* @return Optional property which must either be set to True or omitted.
*
*/
private @Nullable Boolean requireGuestProvisionSignal;
/**
* @return Specifies set of certificates that should be installed onto the virtual machines in the scale set. To install certificates on a virtual machine it is recommended to use the [Azure Key Vault virtual machine extension for Linux](https://docs.microsoft.com/azure/virtual-machines/extensions/key-vault-linux) or the [Azure Key Vault virtual machine extension for Windows](https://docs.microsoft.com/azure/virtual-machines/extensions/key-vault-windows).
*
*/
private @Nullable List secrets;
/**
* @return Specifies Windows operating system settings on the virtual machine.
*
*/
private @Nullable WindowsConfigurationResponse windowsConfiguration;
private VirtualMachineScaleSetOSProfileResponse() {}
/**
* @return Specifies the password of the administrator account. <br><br> **Minimum-length (Windows):** 8 characters <br><br> **Minimum-length (Linux):** 6 characters <br><br> **Max-length (Windows):** 123 characters <br><br> **Max-length (Linux):** 72 characters <br><br> **Complexity requirements:** 3 out of 4 conditions below need to be fulfilled <br> Has lower characters <br>Has upper characters <br> Has a digit <br> Has a special character (Regex match [\W_]) <br><br> **Disallowed values:** "abc{@literal @}123", "P{@literal @}$$w0rd", "P{@literal @}ssw0rd", "P{@literal @}ssword123", "Pa$$word", "pass{@literal @}word1", "Password!", "Password1", "Password22", "iloveyou!" <br><br> For resetting the password, see [How to reset the Remote Desktop service or its login password in a Windows VM](https://docs.microsoft.com/troubleshoot/azure/virtual-machines/reset-rdp) <br><br> For resetting root password, see [Manage users, SSH, and check or repair disks on Azure Linux VMs using the VMAccess Extension](https://docs.microsoft.com/troubleshoot/azure/virtual-machines/troubleshoot-ssh-connection)
*
*/
public Optional adminPassword() {
return Optional.ofNullable(this.adminPassword);
}
/**
* @return Specifies the name of the administrator account. <br><br> **Windows-only restriction:** Cannot end in "." <br><br> **Disallowed values:** "administrator", "admin", "user", "user1", "test", "user2", "test1", "user3", "admin1", "1", "123", "a", "actuser", "adm", "admin2", "aspnet", "backup", "console", "david", "guest", "john", "owner", "root", "server", "sql", "support", "support_388945a0", "sys", "test2", "test3", "user4", "user5". <br><br> **Minimum-length (Linux):** 1 character <br><br> **Max-length (Linux):** 64 characters <br><br> **Max-length (Windows):** 20 characters
*
*/
public Optional adminUsername() {
return Optional.ofNullable(this.adminUsername);
}
/**
* @return Specifies whether extension operations should be allowed on the virtual machine scale set. This may only be set to False when no extensions are present on the virtual machine scale set.
*
*/
public Optional allowExtensionOperations() {
return Optional.ofNullable(this.allowExtensionOperations);
}
/**
* @return Specifies the computer name prefix for all of the virtual machines in the scale set. Computer name prefixes must be 1 to 15 characters long.
*
*/
public Optional computerNamePrefix() {
return Optional.ofNullable(this.computerNamePrefix);
}
/**
* @return Specifies a base-64 encoded string of custom data. The base-64 encoded string is decoded to a binary array that is saved as a file on the Virtual Machine. The maximum length of the binary array is 65535 bytes. For using cloud-init for your VM, see [Using cloud-init to customize a Linux VM during creation](https://docs.microsoft.com/azure/virtual-machines/linux/using-cloud-init)
*
*/
public Optional customData() {
return Optional.ofNullable(this.customData);
}
/**
* @return Specifies the Linux operating system settings on the virtual machine. For a list of supported Linux distributions, see [Linux on Azure-Endorsed Distributions](https://docs.microsoft.com/azure/virtual-machines/linux/endorsed-distros).
*
*/
public Optional linuxConfiguration() {
return Optional.ofNullable(this.linuxConfiguration);
}
/**
* @return Optional property which must either be set to True or omitted.
*
*/
public Optional requireGuestProvisionSignal() {
return Optional.ofNullable(this.requireGuestProvisionSignal);
}
/**
* @return Specifies set of certificates that should be installed onto the virtual machines in the scale set. To install certificates on a virtual machine it is recommended to use the [Azure Key Vault virtual machine extension for Linux](https://docs.microsoft.com/azure/virtual-machines/extensions/key-vault-linux) or the [Azure Key Vault virtual machine extension for Windows](https://docs.microsoft.com/azure/virtual-machines/extensions/key-vault-windows).
*
*/
public List secrets() {
return this.secrets == null ? List.of() : this.secrets;
}
/**
* @return Specifies Windows operating system settings on the virtual machine.
*
*/
public Optional windowsConfiguration() {
return Optional.ofNullable(this.windowsConfiguration);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VirtualMachineScaleSetOSProfileResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String adminPassword;
private @Nullable String adminUsername;
private @Nullable Boolean allowExtensionOperations;
private @Nullable String computerNamePrefix;
private @Nullable String customData;
private @Nullable LinuxConfigurationResponse linuxConfiguration;
private @Nullable Boolean requireGuestProvisionSignal;
private @Nullable List secrets;
private @Nullable WindowsConfigurationResponse windowsConfiguration;
public Builder() {}
public Builder(VirtualMachineScaleSetOSProfileResponse defaults) {
Objects.requireNonNull(defaults);
this.adminPassword = defaults.adminPassword;
this.adminUsername = defaults.adminUsername;
this.allowExtensionOperations = defaults.allowExtensionOperations;
this.computerNamePrefix = defaults.computerNamePrefix;
this.customData = defaults.customData;
this.linuxConfiguration = defaults.linuxConfiguration;
this.requireGuestProvisionSignal = defaults.requireGuestProvisionSignal;
this.secrets = defaults.secrets;
this.windowsConfiguration = defaults.windowsConfiguration;
}
@CustomType.Setter
public Builder adminPassword(@Nullable String adminPassword) {
this.adminPassword = adminPassword;
return this;
}
@CustomType.Setter
public Builder adminUsername(@Nullable String adminUsername) {
this.adminUsername = adminUsername;
return this;
}
@CustomType.Setter
public Builder allowExtensionOperations(@Nullable Boolean allowExtensionOperations) {
this.allowExtensionOperations = allowExtensionOperations;
return this;
}
@CustomType.Setter
public Builder computerNamePrefix(@Nullable String computerNamePrefix) {
this.computerNamePrefix = computerNamePrefix;
return this;
}
@CustomType.Setter
public Builder customData(@Nullable String customData) {
this.customData = customData;
return this;
}
@CustomType.Setter
public Builder linuxConfiguration(@Nullable LinuxConfigurationResponse linuxConfiguration) {
this.linuxConfiguration = linuxConfiguration;
return this;
}
@CustomType.Setter
public Builder requireGuestProvisionSignal(@Nullable Boolean requireGuestProvisionSignal) {
this.requireGuestProvisionSignal = requireGuestProvisionSignal;
return this;
}
@CustomType.Setter
public Builder secrets(@Nullable List secrets) {
this.secrets = secrets;
return this;
}
public Builder secrets(VaultSecretGroupResponse... secrets) {
return secrets(List.of(secrets));
}
@CustomType.Setter
public Builder windowsConfiguration(@Nullable WindowsConfigurationResponse windowsConfiguration) {
this.windowsConfiguration = windowsConfiguration;
return this;
}
public VirtualMachineScaleSetOSProfileResponse build() {
final var _resultValue = new VirtualMachineScaleSetOSProfileResponse();
_resultValue.adminPassword = adminPassword;
_resultValue.adminUsername = adminUsername;
_resultValue.allowExtensionOperations = allowExtensionOperations;
_resultValue.computerNamePrefix = computerNamePrefix;
_resultValue.customData = customData;
_resultValue.linuxConfiguration = linuxConfiguration;
_resultValue.requireGuestProvisionSignal = requireGuestProvisionSignal;
_resultValue.secrets = secrets;
_resultValue.windowsConfiguration = windowsConfiguration;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy