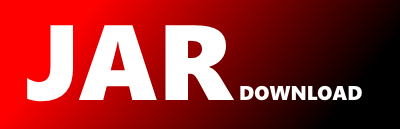
com.pulumi.azurenative.containerservice.outputs.GetLoadBalancerResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerservice.outputs;
import com.pulumi.azurenative.containerservice.outputs.LabelSelectorResponse;
import com.pulumi.azurenative.containerservice.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetLoadBalancerResult {
/**
* @return Whether to automatically place services on the load balancer. If not supplied, the default value is true. If set to false manually, both of the external and the internal load balancer will not be selected for services unless they explicitly target it.
*
*/
private @Nullable Boolean allowServicePlacement;
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
private String id;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Nodes that match this selector will be possible members of this load balancer.
*
*/
private @Nullable LabelSelectorResponse nodeSelector;
/**
* @return Required field. A string value that must specify the ID of an existing agent pool. All nodes in the given pool will always be added to this load balancer. This agent pool must have at least one node and minCount>=1 for autoscaling operations. An agent pool can only be the primary pool for a single load balancer.
*
*/
private String primaryAgentPoolName;
/**
* @return The current provisioning state.
*
*/
private String provisioningState;
/**
* @return Only services that must match this selector can be placed on this load balancer.
*
*/
private @Nullable LabelSelectorResponse serviceLabelSelector;
/**
* @return Services created in namespaces that match the selector can be placed on this load balancer.
*
*/
private @Nullable LabelSelectorResponse serviceNamespaceSelector;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetLoadBalancerResult() {}
/**
* @return Whether to automatically place services on the load balancer. If not supplied, the default value is true. If set to false manually, both of the external and the internal load balancer will not be selected for services unless they explicitly target it.
*
*/
public Optional allowServicePlacement() {
return Optional.ofNullable(this.allowServicePlacement);
}
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Nodes that match this selector will be possible members of this load balancer.
*
*/
public Optional nodeSelector() {
return Optional.ofNullable(this.nodeSelector);
}
/**
* @return Required field. A string value that must specify the ID of an existing agent pool. All nodes in the given pool will always be added to this load balancer. This agent pool must have at least one node and minCount>=1 for autoscaling operations. An agent pool can only be the primary pool for a single load balancer.
*
*/
public String primaryAgentPoolName() {
return this.primaryAgentPoolName;
}
/**
* @return The current provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Only services that must match this selector can be placed on this load balancer.
*
*/
public Optional serviceLabelSelector() {
return Optional.ofNullable(this.serviceLabelSelector);
}
/**
* @return Services created in namespaces that match the selector can be placed on this load balancer.
*
*/
public Optional serviceNamespaceSelector() {
return Optional.ofNullable(this.serviceNamespaceSelector);
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetLoadBalancerResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowServicePlacement;
private String id;
private String name;
private @Nullable LabelSelectorResponse nodeSelector;
private String primaryAgentPoolName;
private String provisioningState;
private @Nullable LabelSelectorResponse serviceLabelSelector;
private @Nullable LabelSelectorResponse serviceNamespaceSelector;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetLoadBalancerResult defaults) {
Objects.requireNonNull(defaults);
this.allowServicePlacement = defaults.allowServicePlacement;
this.id = defaults.id;
this.name = defaults.name;
this.nodeSelector = defaults.nodeSelector;
this.primaryAgentPoolName = defaults.primaryAgentPoolName;
this.provisioningState = defaults.provisioningState;
this.serviceLabelSelector = defaults.serviceLabelSelector;
this.serviceNamespaceSelector = defaults.serviceNamespaceSelector;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder allowServicePlacement(@Nullable Boolean allowServicePlacement) {
this.allowServicePlacement = allowServicePlacement;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder nodeSelector(@Nullable LabelSelectorResponse nodeSelector) {
this.nodeSelector = nodeSelector;
return this;
}
@CustomType.Setter
public Builder primaryAgentPoolName(String primaryAgentPoolName) {
if (primaryAgentPoolName == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "primaryAgentPoolName");
}
this.primaryAgentPoolName = primaryAgentPoolName;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder serviceLabelSelector(@Nullable LabelSelectorResponse serviceLabelSelector) {
this.serviceLabelSelector = serviceLabelSelector;
return this;
}
@CustomType.Setter
public Builder serviceNamespaceSelector(@Nullable LabelSelectorResponse serviceNamespaceSelector) {
this.serviceNamespaceSelector = serviceNamespaceSelector;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerResult", "type");
}
this.type = type;
return this;
}
public GetLoadBalancerResult build() {
final var _resultValue = new GetLoadBalancerResult();
_resultValue.allowServicePlacement = allowServicePlacement;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.nodeSelector = nodeSelector;
_resultValue.primaryAgentPoolName = primaryAgentPoolName;
_resultValue.provisioningState = provisioningState;
_resultValue.serviceLabelSelector = serviceLabelSelector;
_resultValue.serviceNamespaceSelector = serviceNamespaceSelector;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy