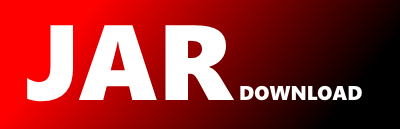
com.pulumi.azurenative.containerservice.outputs.ManagedClusterAgentPoolProfileResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerservice.outputs;
import com.pulumi.azurenative.containerservice.outputs.AgentPoolUpgradeSettingsResponse;
import com.pulumi.azurenative.containerservice.outputs.CreationDataResponse;
import com.pulumi.azurenative.containerservice.outputs.KubeletConfigResponse;
import com.pulumi.azurenative.containerservice.outputs.LinuxOSConfigResponse;
import com.pulumi.azurenative.containerservice.outputs.PowerStateResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ManagedClusterAgentPoolProfileResponse {
/**
* @return The list of Availability zones to use for nodes. This can only be specified if the AgentPoolType property is 'VirtualMachineScaleSets'.
*
*/
private @Nullable List availabilityZones;
/**
* @return Number of agents (VMs) to host docker containers. Allowed values must be in the range of 0 to 1000 (inclusive) for user pools and in the range of 1 to 1000 (inclusive) for system pools. The default value is 1.
*
*/
private @Nullable Integer count;
/**
* @return CreationData to be used to specify the source Snapshot ID if the node pool will be created/upgraded using a snapshot.
*
*/
private @Nullable CreationDataResponse creationData;
/**
* @return If orchestratorVersion is a fully specified version <major.minor.patch>, this field will be exactly equal to it. If orchestratorVersion is <major.minor>, this field will contain the full <major.minor.patch> version being used.
*
*/
private String currentOrchestratorVersion;
/**
* @return Whether to enable auto-scaler
*
*/
private @Nullable Boolean enableAutoScaling;
/**
* @return This is only supported on certain VM sizes and in certain Azure regions. For more information, see: https://docs.microsoft.com/azure/aks/enable-host-encryption
*
*/
private @Nullable Boolean enableEncryptionAtHost;
/**
* @return See [Add a FIPS-enabled node pool](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#add-a-fips-enabled-node-pool-preview) for more details.
*
*/
private @Nullable Boolean enableFIPS;
/**
* @return Some scenarios may require nodes in a node pool to receive their own dedicated public IP addresses. A common scenario is for gaming workloads, where a console needs to make a direct connection to a cloud virtual machine to minimize hops. For more information see [assigning a public IP per node](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#assign-a-public-ip-per-node-for-your-node-pools). The default is false.
*
*/
private @Nullable Boolean enableNodePublicIP;
/**
* @return Whether to enable UltraSSD
*
*/
private @Nullable Boolean enableUltraSSD;
/**
* @return GPUInstanceProfile to be used to specify GPU MIG instance profile for supported GPU VM SKU.
*
*/
private @Nullable String gpuInstanceProfile;
/**
* @return This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/hostGroups/{hostGroupName}. For more information see [Azure dedicated hosts](https://docs.microsoft.com/azure/virtual-machines/dedicated-hosts).
*
*/
private @Nullable String hostGroupID;
/**
* @return The Kubelet configuration on the agent pool nodes.
*
*/
private @Nullable KubeletConfigResponse kubeletConfig;
/**
* @return Determines the placement of emptyDir volumes, container runtime data root, and Kubelet ephemeral storage.
*
*/
private @Nullable String kubeletDiskType;
/**
* @return The OS configuration of Linux agent nodes.
*
*/
private @Nullable LinuxOSConfigResponse linuxOSConfig;
/**
* @return The maximum number of nodes for auto-scaling
*
*/
private @Nullable Integer maxCount;
/**
* @return The maximum number of pods that can run on a node.
*
*/
private @Nullable Integer maxPods;
/**
* @return The minimum number of nodes for auto-scaling
*
*/
private @Nullable Integer minCount;
/**
* @return A cluster must have at least one 'System' Agent Pool at all times. For additional information on agent pool restrictions and best practices, see: https://docs.microsoft.com/azure/aks/use-system-pools
*
*/
private @Nullable String mode;
/**
* @return Windows agent pool names must be 6 characters or less.
*
*/
private String name;
/**
* @return The version of node image
*
*/
private String nodeImageVersion;
/**
* @return The node labels to be persisted across all nodes in agent pool.
*
*/
private @Nullable Map nodeLabels;
/**
* @return This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/publicIPPrefixes/{publicIPPrefixName}
*
*/
private @Nullable String nodePublicIPPrefixID;
/**
* @return The taints added to new nodes during node pool create and scale. For example, key=value:NoSchedule.
*
*/
private @Nullable List nodeTaints;
/**
* @return Both patch version <major.minor.patch> (e.g. 1.20.13) and <major.minor> (e.g. 1.20) are supported. When <major.minor> is specified, the latest supported GA patch version is chosen automatically. Updating the cluster with the same <major.minor> once it has been created (e.g. 1.14.x -> 1.14) will not trigger an upgrade, even if a newer patch version is available. As a best practice, you should upgrade all node pools in an AKS cluster to the same Kubernetes version. The node pool version must have the same major version as the control plane. The node pool minor version must be within two minor versions of the control plane version. The node pool version cannot be greater than the control plane version. For more information see [upgrading a node pool](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#upgrade-a-node-pool).
*
*/
private @Nullable String orchestratorVersion;
/**
* @return OS Disk Size in GB to be used to specify the disk size for every machine in the master/agent pool. If you specify 0, it will apply the default osDisk size according to the vmSize specified.
*
*/
private @Nullable Integer osDiskSizeGB;
/**
* @return The default is 'Ephemeral' if the VM supports it and has a cache disk larger than the requested OSDiskSizeGB. Otherwise, defaults to 'Managed'. May not be changed after creation. For more information see [Ephemeral OS](https://docs.microsoft.com/azure/aks/cluster-configuration#ephemeral-os).
*
*/
private @Nullable String osDiskType;
/**
* @return Specifies the OS SKU used by the agent pool. The default is Ubuntu if OSType is Linux. The default is Windows2019 when Kubernetes <= 1.24 or Windows2022 when Kubernetes >= 1.25 if OSType is Windows.
*
*/
private @Nullable String osSKU;
/**
* @return The operating system type. The default is Linux.
*
*/
private @Nullable String osType;
/**
* @return If omitted, pod IPs are statically assigned on the node subnet (see vnetSubnetID for more details). This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{virtualNetworkName}/subnets/{subnetName}
*
*/
private @Nullable String podSubnetID;
/**
* @return When an Agent Pool is first created it is initially Running. The Agent Pool can be stopped by setting this field to Stopped. A stopped Agent Pool stops all of its VMs and does not accrue billing charges. An Agent Pool can only be stopped if it is Running and provisioning state is Succeeded
*
*/
private @Nullable PowerStateResponse powerState;
/**
* @return The current deployment or provisioning state.
*
*/
private String provisioningState;
/**
* @return The ID for Proximity Placement Group.
*
*/
private @Nullable String proximityPlacementGroupID;
/**
* @return This also effects the cluster autoscaler behavior. If not specified, it defaults to Delete.
*
*/
private @Nullable String scaleDownMode;
/**
* @return This cannot be specified unless the scaleSetPriority is 'Spot'. If not specified, the default is 'Delete'.
*
*/
private @Nullable String scaleSetEvictionPolicy;
/**
* @return The Virtual Machine Scale Set priority. If not specified, the default is 'Regular'.
*
*/
private @Nullable String scaleSetPriority;
/**
* @return Possible values are any decimal value greater than zero or -1 which indicates the willingness to pay any on-demand price. For more details on spot pricing, see [spot VMs pricing](https://docs.microsoft.com/azure/virtual-machines/spot-vms#pricing)
*
*/
private @Nullable Double spotMaxPrice;
/**
* @return The tags to be persisted on the agent pool virtual machine scale set.
*
*/
private @Nullable Map tags;
/**
* @return The type of Agent Pool.
*
*/
private @Nullable String type;
/**
* @return Settings for upgrading the agentpool
*
*/
private @Nullable AgentPoolUpgradeSettingsResponse upgradeSettings;
/**
* @return VM size availability varies by region. If a node contains insufficient compute resources (memory, cpu, etc) pods might fail to run correctly. For more details on restricted VM sizes, see: https://docs.microsoft.com/azure/aks/quotas-skus-regions
*
*/
private @Nullable String vmSize;
/**
* @return If this is not specified, a VNET and subnet will be generated and used. If no podSubnetID is specified, this applies to nodes and pods, otherwise it applies to just nodes. This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{virtualNetworkName}/subnets/{subnetName}
*
*/
private @Nullable String vnetSubnetID;
/**
* @return Determines the type of workload a node can run.
*
*/
private @Nullable String workloadRuntime;
private ManagedClusterAgentPoolProfileResponse() {}
/**
* @return The list of Availability zones to use for nodes. This can only be specified if the AgentPoolType property is 'VirtualMachineScaleSets'.
*
*/
public List availabilityZones() {
return this.availabilityZones == null ? List.of() : this.availabilityZones;
}
/**
* @return Number of agents (VMs) to host docker containers. Allowed values must be in the range of 0 to 1000 (inclusive) for user pools and in the range of 1 to 1000 (inclusive) for system pools. The default value is 1.
*
*/
public Optional count() {
return Optional.ofNullable(this.count);
}
/**
* @return CreationData to be used to specify the source Snapshot ID if the node pool will be created/upgraded using a snapshot.
*
*/
public Optional creationData() {
return Optional.ofNullable(this.creationData);
}
/**
* @return If orchestratorVersion is a fully specified version <major.minor.patch>, this field will be exactly equal to it. If orchestratorVersion is <major.minor>, this field will contain the full <major.minor.patch> version being used.
*
*/
public String currentOrchestratorVersion() {
return this.currentOrchestratorVersion;
}
/**
* @return Whether to enable auto-scaler
*
*/
public Optional enableAutoScaling() {
return Optional.ofNullable(this.enableAutoScaling);
}
/**
* @return This is only supported on certain VM sizes and in certain Azure regions. For more information, see: https://docs.microsoft.com/azure/aks/enable-host-encryption
*
*/
public Optional enableEncryptionAtHost() {
return Optional.ofNullable(this.enableEncryptionAtHost);
}
/**
* @return See [Add a FIPS-enabled node pool](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#add-a-fips-enabled-node-pool-preview) for more details.
*
*/
public Optional enableFIPS() {
return Optional.ofNullable(this.enableFIPS);
}
/**
* @return Some scenarios may require nodes in a node pool to receive their own dedicated public IP addresses. A common scenario is for gaming workloads, where a console needs to make a direct connection to a cloud virtual machine to minimize hops. For more information see [assigning a public IP per node](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#assign-a-public-ip-per-node-for-your-node-pools). The default is false.
*
*/
public Optional enableNodePublicIP() {
return Optional.ofNullable(this.enableNodePublicIP);
}
/**
* @return Whether to enable UltraSSD
*
*/
public Optional enableUltraSSD() {
return Optional.ofNullable(this.enableUltraSSD);
}
/**
* @return GPUInstanceProfile to be used to specify GPU MIG instance profile for supported GPU VM SKU.
*
*/
public Optional gpuInstanceProfile() {
return Optional.ofNullable(this.gpuInstanceProfile);
}
/**
* @return This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/hostGroups/{hostGroupName}. For more information see [Azure dedicated hosts](https://docs.microsoft.com/azure/virtual-machines/dedicated-hosts).
*
*/
public Optional hostGroupID() {
return Optional.ofNullable(this.hostGroupID);
}
/**
* @return The Kubelet configuration on the agent pool nodes.
*
*/
public Optional kubeletConfig() {
return Optional.ofNullable(this.kubeletConfig);
}
/**
* @return Determines the placement of emptyDir volumes, container runtime data root, and Kubelet ephemeral storage.
*
*/
public Optional kubeletDiskType() {
return Optional.ofNullable(this.kubeletDiskType);
}
/**
* @return The OS configuration of Linux agent nodes.
*
*/
public Optional linuxOSConfig() {
return Optional.ofNullable(this.linuxOSConfig);
}
/**
* @return The maximum number of nodes for auto-scaling
*
*/
public Optional maxCount() {
return Optional.ofNullable(this.maxCount);
}
/**
* @return The maximum number of pods that can run on a node.
*
*/
public Optional maxPods() {
return Optional.ofNullable(this.maxPods);
}
/**
* @return The minimum number of nodes for auto-scaling
*
*/
public Optional minCount() {
return Optional.ofNullable(this.minCount);
}
/**
* @return A cluster must have at least one 'System' Agent Pool at all times. For additional information on agent pool restrictions and best practices, see: https://docs.microsoft.com/azure/aks/use-system-pools
*
*/
public Optional mode() {
return Optional.ofNullable(this.mode);
}
/**
* @return Windows agent pool names must be 6 characters or less.
*
*/
public String name() {
return this.name;
}
/**
* @return The version of node image
*
*/
public String nodeImageVersion() {
return this.nodeImageVersion;
}
/**
* @return The node labels to be persisted across all nodes in agent pool.
*
*/
public Map nodeLabels() {
return this.nodeLabels == null ? Map.of() : this.nodeLabels;
}
/**
* @return This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/publicIPPrefixes/{publicIPPrefixName}
*
*/
public Optional nodePublicIPPrefixID() {
return Optional.ofNullable(this.nodePublicIPPrefixID);
}
/**
* @return The taints added to new nodes during node pool create and scale. For example, key=value:NoSchedule.
*
*/
public List nodeTaints() {
return this.nodeTaints == null ? List.of() : this.nodeTaints;
}
/**
* @return Both patch version <major.minor.patch> (e.g. 1.20.13) and <major.minor> (e.g. 1.20) are supported. When <major.minor> is specified, the latest supported GA patch version is chosen automatically. Updating the cluster with the same <major.minor> once it has been created (e.g. 1.14.x -> 1.14) will not trigger an upgrade, even if a newer patch version is available. As a best practice, you should upgrade all node pools in an AKS cluster to the same Kubernetes version. The node pool version must have the same major version as the control plane. The node pool minor version must be within two minor versions of the control plane version. The node pool version cannot be greater than the control plane version. For more information see [upgrading a node pool](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#upgrade-a-node-pool).
*
*/
public Optional orchestratorVersion() {
return Optional.ofNullable(this.orchestratorVersion);
}
/**
* @return OS Disk Size in GB to be used to specify the disk size for every machine in the master/agent pool. If you specify 0, it will apply the default osDisk size according to the vmSize specified.
*
*/
public Optional osDiskSizeGB() {
return Optional.ofNullable(this.osDiskSizeGB);
}
/**
* @return The default is 'Ephemeral' if the VM supports it and has a cache disk larger than the requested OSDiskSizeGB. Otherwise, defaults to 'Managed'. May not be changed after creation. For more information see [Ephemeral OS](https://docs.microsoft.com/azure/aks/cluster-configuration#ephemeral-os).
*
*/
public Optional osDiskType() {
return Optional.ofNullable(this.osDiskType);
}
/**
* @return Specifies the OS SKU used by the agent pool. The default is Ubuntu if OSType is Linux. The default is Windows2019 when Kubernetes <= 1.24 or Windows2022 when Kubernetes >= 1.25 if OSType is Windows.
*
*/
public Optional osSKU() {
return Optional.ofNullable(this.osSKU);
}
/**
* @return The operating system type. The default is Linux.
*
*/
public Optional osType() {
return Optional.ofNullable(this.osType);
}
/**
* @return If omitted, pod IPs are statically assigned on the node subnet (see vnetSubnetID for more details). This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{virtualNetworkName}/subnets/{subnetName}
*
*/
public Optional podSubnetID() {
return Optional.ofNullable(this.podSubnetID);
}
/**
* @return When an Agent Pool is first created it is initially Running. The Agent Pool can be stopped by setting this field to Stopped. A stopped Agent Pool stops all of its VMs and does not accrue billing charges. An Agent Pool can only be stopped if it is Running and provisioning state is Succeeded
*
*/
public Optional powerState() {
return Optional.ofNullable(this.powerState);
}
/**
* @return The current deployment or provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The ID for Proximity Placement Group.
*
*/
public Optional proximityPlacementGroupID() {
return Optional.ofNullable(this.proximityPlacementGroupID);
}
/**
* @return This also effects the cluster autoscaler behavior. If not specified, it defaults to Delete.
*
*/
public Optional scaleDownMode() {
return Optional.ofNullable(this.scaleDownMode);
}
/**
* @return This cannot be specified unless the scaleSetPriority is 'Spot'. If not specified, the default is 'Delete'.
*
*/
public Optional scaleSetEvictionPolicy() {
return Optional.ofNullable(this.scaleSetEvictionPolicy);
}
/**
* @return The Virtual Machine Scale Set priority. If not specified, the default is 'Regular'.
*
*/
public Optional scaleSetPriority() {
return Optional.ofNullable(this.scaleSetPriority);
}
/**
* @return Possible values are any decimal value greater than zero or -1 which indicates the willingness to pay any on-demand price. For more details on spot pricing, see [spot VMs pricing](https://docs.microsoft.com/azure/virtual-machines/spot-vms#pricing)
*
*/
public Optional spotMaxPrice() {
return Optional.ofNullable(this.spotMaxPrice);
}
/**
* @return The tags to be persisted on the agent pool virtual machine scale set.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of Agent Pool.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
/**
* @return Settings for upgrading the agentpool
*
*/
public Optional upgradeSettings() {
return Optional.ofNullable(this.upgradeSettings);
}
/**
* @return VM size availability varies by region. If a node contains insufficient compute resources (memory, cpu, etc) pods might fail to run correctly. For more details on restricted VM sizes, see: https://docs.microsoft.com/azure/aks/quotas-skus-regions
*
*/
public Optional vmSize() {
return Optional.ofNullable(this.vmSize);
}
/**
* @return If this is not specified, a VNET and subnet will be generated and used. If no podSubnetID is specified, this applies to nodes and pods, otherwise it applies to just nodes. This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{virtualNetworkName}/subnets/{subnetName}
*
*/
public Optional vnetSubnetID() {
return Optional.ofNullable(this.vnetSubnetID);
}
/**
* @return Determines the type of workload a node can run.
*
*/
public Optional workloadRuntime() {
return Optional.ofNullable(this.workloadRuntime);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ManagedClusterAgentPoolProfileResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List availabilityZones;
private @Nullable Integer count;
private @Nullable CreationDataResponse creationData;
private String currentOrchestratorVersion;
private @Nullable Boolean enableAutoScaling;
private @Nullable Boolean enableEncryptionAtHost;
private @Nullable Boolean enableFIPS;
private @Nullable Boolean enableNodePublicIP;
private @Nullable Boolean enableUltraSSD;
private @Nullable String gpuInstanceProfile;
private @Nullable String hostGroupID;
private @Nullable KubeletConfigResponse kubeletConfig;
private @Nullable String kubeletDiskType;
private @Nullable LinuxOSConfigResponse linuxOSConfig;
private @Nullable Integer maxCount;
private @Nullable Integer maxPods;
private @Nullable Integer minCount;
private @Nullable String mode;
private String name;
private String nodeImageVersion;
private @Nullable Map nodeLabels;
private @Nullable String nodePublicIPPrefixID;
private @Nullable List nodeTaints;
private @Nullable String orchestratorVersion;
private @Nullable Integer osDiskSizeGB;
private @Nullable String osDiskType;
private @Nullable String osSKU;
private @Nullable String osType;
private @Nullable String podSubnetID;
private @Nullable PowerStateResponse powerState;
private String provisioningState;
private @Nullable String proximityPlacementGroupID;
private @Nullable String scaleDownMode;
private @Nullable String scaleSetEvictionPolicy;
private @Nullable String scaleSetPriority;
private @Nullable Double spotMaxPrice;
private @Nullable Map tags;
private @Nullable String type;
private @Nullable AgentPoolUpgradeSettingsResponse upgradeSettings;
private @Nullable String vmSize;
private @Nullable String vnetSubnetID;
private @Nullable String workloadRuntime;
public Builder() {}
public Builder(ManagedClusterAgentPoolProfileResponse defaults) {
Objects.requireNonNull(defaults);
this.availabilityZones = defaults.availabilityZones;
this.count = defaults.count;
this.creationData = defaults.creationData;
this.currentOrchestratorVersion = defaults.currentOrchestratorVersion;
this.enableAutoScaling = defaults.enableAutoScaling;
this.enableEncryptionAtHost = defaults.enableEncryptionAtHost;
this.enableFIPS = defaults.enableFIPS;
this.enableNodePublicIP = defaults.enableNodePublicIP;
this.enableUltraSSD = defaults.enableUltraSSD;
this.gpuInstanceProfile = defaults.gpuInstanceProfile;
this.hostGroupID = defaults.hostGroupID;
this.kubeletConfig = defaults.kubeletConfig;
this.kubeletDiskType = defaults.kubeletDiskType;
this.linuxOSConfig = defaults.linuxOSConfig;
this.maxCount = defaults.maxCount;
this.maxPods = defaults.maxPods;
this.minCount = defaults.minCount;
this.mode = defaults.mode;
this.name = defaults.name;
this.nodeImageVersion = defaults.nodeImageVersion;
this.nodeLabels = defaults.nodeLabels;
this.nodePublicIPPrefixID = defaults.nodePublicIPPrefixID;
this.nodeTaints = defaults.nodeTaints;
this.orchestratorVersion = defaults.orchestratorVersion;
this.osDiskSizeGB = defaults.osDiskSizeGB;
this.osDiskType = defaults.osDiskType;
this.osSKU = defaults.osSKU;
this.osType = defaults.osType;
this.podSubnetID = defaults.podSubnetID;
this.powerState = defaults.powerState;
this.provisioningState = defaults.provisioningState;
this.proximityPlacementGroupID = defaults.proximityPlacementGroupID;
this.scaleDownMode = defaults.scaleDownMode;
this.scaleSetEvictionPolicy = defaults.scaleSetEvictionPolicy;
this.scaleSetPriority = defaults.scaleSetPriority;
this.spotMaxPrice = defaults.spotMaxPrice;
this.tags = defaults.tags;
this.type = defaults.type;
this.upgradeSettings = defaults.upgradeSettings;
this.vmSize = defaults.vmSize;
this.vnetSubnetID = defaults.vnetSubnetID;
this.workloadRuntime = defaults.workloadRuntime;
}
@CustomType.Setter
public Builder availabilityZones(@Nullable List availabilityZones) {
this.availabilityZones = availabilityZones;
return this;
}
public Builder availabilityZones(String... availabilityZones) {
return availabilityZones(List.of(availabilityZones));
}
@CustomType.Setter
public Builder count(@Nullable Integer count) {
this.count = count;
return this;
}
@CustomType.Setter
public Builder creationData(@Nullable CreationDataResponse creationData) {
this.creationData = creationData;
return this;
}
@CustomType.Setter
public Builder currentOrchestratorVersion(String currentOrchestratorVersion) {
if (currentOrchestratorVersion == null) {
throw new MissingRequiredPropertyException("ManagedClusterAgentPoolProfileResponse", "currentOrchestratorVersion");
}
this.currentOrchestratorVersion = currentOrchestratorVersion;
return this;
}
@CustomType.Setter
public Builder enableAutoScaling(@Nullable Boolean enableAutoScaling) {
this.enableAutoScaling = enableAutoScaling;
return this;
}
@CustomType.Setter
public Builder enableEncryptionAtHost(@Nullable Boolean enableEncryptionAtHost) {
this.enableEncryptionAtHost = enableEncryptionAtHost;
return this;
}
@CustomType.Setter
public Builder enableFIPS(@Nullable Boolean enableFIPS) {
this.enableFIPS = enableFIPS;
return this;
}
@CustomType.Setter
public Builder enableNodePublicIP(@Nullable Boolean enableNodePublicIP) {
this.enableNodePublicIP = enableNodePublicIP;
return this;
}
@CustomType.Setter
public Builder enableUltraSSD(@Nullable Boolean enableUltraSSD) {
this.enableUltraSSD = enableUltraSSD;
return this;
}
@CustomType.Setter
public Builder gpuInstanceProfile(@Nullable String gpuInstanceProfile) {
this.gpuInstanceProfile = gpuInstanceProfile;
return this;
}
@CustomType.Setter
public Builder hostGroupID(@Nullable String hostGroupID) {
this.hostGroupID = hostGroupID;
return this;
}
@CustomType.Setter
public Builder kubeletConfig(@Nullable KubeletConfigResponse kubeletConfig) {
this.kubeletConfig = kubeletConfig;
return this;
}
@CustomType.Setter
public Builder kubeletDiskType(@Nullable String kubeletDiskType) {
this.kubeletDiskType = kubeletDiskType;
return this;
}
@CustomType.Setter
public Builder linuxOSConfig(@Nullable LinuxOSConfigResponse linuxOSConfig) {
this.linuxOSConfig = linuxOSConfig;
return this;
}
@CustomType.Setter
public Builder maxCount(@Nullable Integer maxCount) {
this.maxCount = maxCount;
return this;
}
@CustomType.Setter
public Builder maxPods(@Nullable Integer maxPods) {
this.maxPods = maxPods;
return this;
}
@CustomType.Setter
public Builder minCount(@Nullable Integer minCount) {
this.minCount = minCount;
return this;
}
@CustomType.Setter
public Builder mode(@Nullable String mode) {
this.mode = mode;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("ManagedClusterAgentPoolProfileResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder nodeImageVersion(String nodeImageVersion) {
if (nodeImageVersion == null) {
throw new MissingRequiredPropertyException("ManagedClusterAgentPoolProfileResponse", "nodeImageVersion");
}
this.nodeImageVersion = nodeImageVersion;
return this;
}
@CustomType.Setter
public Builder nodeLabels(@Nullable Map nodeLabels) {
this.nodeLabels = nodeLabels;
return this;
}
@CustomType.Setter
public Builder nodePublicIPPrefixID(@Nullable String nodePublicIPPrefixID) {
this.nodePublicIPPrefixID = nodePublicIPPrefixID;
return this;
}
@CustomType.Setter
public Builder nodeTaints(@Nullable List nodeTaints) {
this.nodeTaints = nodeTaints;
return this;
}
public Builder nodeTaints(String... nodeTaints) {
return nodeTaints(List.of(nodeTaints));
}
@CustomType.Setter
public Builder orchestratorVersion(@Nullable String orchestratorVersion) {
this.orchestratorVersion = orchestratorVersion;
return this;
}
@CustomType.Setter
public Builder osDiskSizeGB(@Nullable Integer osDiskSizeGB) {
this.osDiskSizeGB = osDiskSizeGB;
return this;
}
@CustomType.Setter
public Builder osDiskType(@Nullable String osDiskType) {
this.osDiskType = osDiskType;
return this;
}
@CustomType.Setter
public Builder osSKU(@Nullable String osSKU) {
this.osSKU = osSKU;
return this;
}
@CustomType.Setter
public Builder osType(@Nullable String osType) {
this.osType = osType;
return this;
}
@CustomType.Setter
public Builder podSubnetID(@Nullable String podSubnetID) {
this.podSubnetID = podSubnetID;
return this;
}
@CustomType.Setter
public Builder powerState(@Nullable PowerStateResponse powerState) {
this.powerState = powerState;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("ManagedClusterAgentPoolProfileResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder proximityPlacementGroupID(@Nullable String proximityPlacementGroupID) {
this.proximityPlacementGroupID = proximityPlacementGroupID;
return this;
}
@CustomType.Setter
public Builder scaleDownMode(@Nullable String scaleDownMode) {
this.scaleDownMode = scaleDownMode;
return this;
}
@CustomType.Setter
public Builder scaleSetEvictionPolicy(@Nullable String scaleSetEvictionPolicy) {
this.scaleSetEvictionPolicy = scaleSetEvictionPolicy;
return this;
}
@CustomType.Setter
public Builder scaleSetPriority(@Nullable String scaleSetPriority) {
this.scaleSetPriority = scaleSetPriority;
return this;
}
@CustomType.Setter
public Builder spotMaxPrice(@Nullable Double spotMaxPrice) {
this.spotMaxPrice = spotMaxPrice;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
@CustomType.Setter
public Builder upgradeSettings(@Nullable AgentPoolUpgradeSettingsResponse upgradeSettings) {
this.upgradeSettings = upgradeSettings;
return this;
}
@CustomType.Setter
public Builder vmSize(@Nullable String vmSize) {
this.vmSize = vmSize;
return this;
}
@CustomType.Setter
public Builder vnetSubnetID(@Nullable String vnetSubnetID) {
this.vnetSubnetID = vnetSubnetID;
return this;
}
@CustomType.Setter
public Builder workloadRuntime(@Nullable String workloadRuntime) {
this.workloadRuntime = workloadRuntime;
return this;
}
public ManagedClusterAgentPoolProfileResponse build() {
final var _resultValue = new ManagedClusterAgentPoolProfileResponse();
_resultValue.availabilityZones = availabilityZones;
_resultValue.count = count;
_resultValue.creationData = creationData;
_resultValue.currentOrchestratorVersion = currentOrchestratorVersion;
_resultValue.enableAutoScaling = enableAutoScaling;
_resultValue.enableEncryptionAtHost = enableEncryptionAtHost;
_resultValue.enableFIPS = enableFIPS;
_resultValue.enableNodePublicIP = enableNodePublicIP;
_resultValue.enableUltraSSD = enableUltraSSD;
_resultValue.gpuInstanceProfile = gpuInstanceProfile;
_resultValue.hostGroupID = hostGroupID;
_resultValue.kubeletConfig = kubeletConfig;
_resultValue.kubeletDiskType = kubeletDiskType;
_resultValue.linuxOSConfig = linuxOSConfig;
_resultValue.maxCount = maxCount;
_resultValue.maxPods = maxPods;
_resultValue.minCount = minCount;
_resultValue.mode = mode;
_resultValue.name = name;
_resultValue.nodeImageVersion = nodeImageVersion;
_resultValue.nodeLabels = nodeLabels;
_resultValue.nodePublicIPPrefixID = nodePublicIPPrefixID;
_resultValue.nodeTaints = nodeTaints;
_resultValue.orchestratorVersion = orchestratorVersion;
_resultValue.osDiskSizeGB = osDiskSizeGB;
_resultValue.osDiskType = osDiskType;
_resultValue.osSKU = osSKU;
_resultValue.osType = osType;
_resultValue.podSubnetID = podSubnetID;
_resultValue.powerState = powerState;
_resultValue.provisioningState = provisioningState;
_resultValue.proximityPlacementGroupID = proximityPlacementGroupID;
_resultValue.scaleDownMode = scaleDownMode;
_resultValue.scaleSetEvictionPolicy = scaleSetEvictionPolicy;
_resultValue.scaleSetPriority = scaleSetPriority;
_resultValue.spotMaxPrice = spotMaxPrice;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.upgradeSettings = upgradeSettings;
_resultValue.vmSize = vmSize;
_resultValue.vnetSubnetID = vnetSubnetID;
_resultValue.workloadRuntime = workloadRuntime;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy