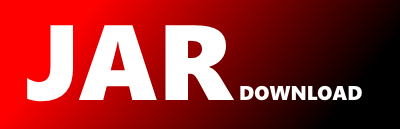
com.pulumi.azurenative.costmanagement.Budget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.costmanagement;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.costmanagement.BudgetArgs;
import com.pulumi.azurenative.costmanagement.outputs.BudgetFilterResponse;
import com.pulumi.azurenative.costmanagement.outputs.BudgetTimePeriodResponse;
import com.pulumi.azurenative.costmanagement.outputs.CurrentSpendResponse;
import com.pulumi.azurenative.costmanagement.outputs.ForecastSpendResponse;
import com.pulumi.azurenative.costmanagement.outputs.NotificationResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A budget resource.
* Azure REST API version: 2023-04-01-preview.
*
* Other available API versions: 2019-04-01-preview, 2023-08-01, 2023-09-01, 2023-11-01.
*
*/
@ResourceType(type="azure-native:costmanagement:Budget")
public class Budget extends com.pulumi.resources.CustomResource {
/**
* The total amount of cost to track with the budget.
*
* Supported for CategoryType(s): Cost.
*
* Required for CategoryType(s): Cost.
*
*/
@Export(name="amount", refs={Double.class}, tree="[0]")
private Output* @Nullable */ Double> amount;
/**
* @return The total amount of cost to track with the budget.
*
* Supported for CategoryType(s): Cost.
*
* Required for CategoryType(s): Cost.
*
*/
public Output> amount() {
return Codegen.optional(this.amount);
}
/**
* The category of the budget.
* - 'Cost' defines a Budget.
* - 'ReservationUtilization' defines a Reservation Utilization Alert Rule.
*
*/
@Export(name="category", refs={String.class}, tree="[0]")
private Output category;
/**
* @return The category of the budget.
* - 'Cost' defines a Budget.
* - 'ReservationUtilization' defines a Reservation Utilization Alert Rule.
*
*/
public Output category() {
return this.category;
}
/**
* The current amount of cost which is being tracked for a budget.
*
* Supported for CategoryType(s): Cost.
*
*/
@Export(name="currentSpend", refs={CurrentSpendResponse.class}, tree="[0]")
private Output currentSpend;
/**
* @return The current amount of cost which is being tracked for a budget.
*
* Supported for CategoryType(s): Cost.
*
*/
public Output currentSpend() {
return this.currentSpend;
}
/**
* eTag of the resource. To handle concurrent update scenario, this field will be used to determine whether the user is updating the latest version or not.
*
*/
@Export(name="eTag", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> eTag;
/**
* @return eTag of the resource. To handle concurrent update scenario, this field will be used to determine whether the user is updating the latest version or not.
*
*/
public Output> eTag() {
return Codegen.optional(this.eTag);
}
/**
* May be used to filter budgets by user-specified dimensions and/or tags.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
*/
@Export(name="filter", refs={BudgetFilterResponse.class}, tree="[0]")
private Output* @Nullable */ BudgetFilterResponse> filter;
/**
* @return May be used to filter budgets by user-specified dimensions and/or tags.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
*/
public Output> filter() {
return Codegen.optional(this.filter);
}
/**
* The forecasted cost which is being tracked for a budget.
*
* Supported for CategoryType(s): Cost.
*
*/
@Export(name="forecastSpend", refs={ForecastSpendResponse.class}, tree="[0]")
private Output forecastSpend;
/**
* @return The forecasted cost which is being tracked for a budget.
*
* Supported for CategoryType(s): Cost.
*
*/
public Output forecastSpend() {
return this.forecastSpend;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Dictionary of notifications associated with the budget.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
* - Constraints for **CategoryType: Cost** - Budget can have up to 5 notifications with thresholdType: Actual and 5 notifications with thresholdType: Forecasted.
* - Constraints for **CategoryType: ReservationUtilization** - Only one notification allowed. thresholdType is not applicable.
*
*/
@Export(name="notifications", refs={Map.class,String.class,NotificationResponse.class}, tree="[0,1,2]")
private Output* @Nullable */ Map> notifications;
/**
* @return Dictionary of notifications associated with the budget.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
* - Constraints for **CategoryType: Cost** - Budget can have up to 5 notifications with thresholdType: Actual and 5 notifications with thresholdType: Forecasted.
* - Constraints for **CategoryType: ReservationUtilization** - Only one notification allowed. thresholdType is not applicable.
*
*/
public Output>> notifications() {
return Codegen.optional(this.notifications);
}
/**
* The time covered by a budget. Tracking of the amount will be reset based on the time grain.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
* Supported timeGrainTypes for **CategoryType: Cost**
*
* - Monthly
* - Quarterly
* - Annually
* - BillingMonth*
* - BillingQuarter*
* - BillingAnnual*
*
* *only supported for Web Direct customers.
*
* Supported timeGrainTypes for **CategoryType: ReservationUtilization**
* - Last7Days
* - Last30Days
*
* Required for CategoryType(s): Cost, ReservationUtilization.
*
*/
@Export(name="timeGrain", refs={String.class}, tree="[0]")
private Output timeGrain;
/**
* @return The time covered by a budget. Tracking of the amount will be reset based on the time grain.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
* Supported timeGrainTypes for **CategoryType: Cost**
*
* - Monthly
* - Quarterly
* - Annually
* - BillingMonth*
* - BillingQuarter*
* - BillingAnnual*
*
* *only supported for Web Direct customers.
*
* Supported timeGrainTypes for **CategoryType: ReservationUtilization**
* - Last7Days
* - Last30Days
*
* Required for CategoryType(s): Cost, ReservationUtilization.
*
*/
public Output timeGrain() {
return this.timeGrain;
}
/**
* The time period that defines the active period of the budget. The budget will evaluate data on or after the startDate and will expire on the endDate.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
* Required for CategoryType(s): Cost, ReservationUtilization.
*
*/
@Export(name="timePeriod", refs={BudgetTimePeriodResponse.class}, tree="[0]")
private Output timePeriod;
/**
* @return The time period that defines the active period of the budget. The budget will evaluate data on or after the startDate and will expire on the endDate.
*
* Supported for CategoryType(s): Cost, ReservationUtilization.
*
* Required for CategoryType(s): Cost, ReservationUtilization.
*
*/
public Output timePeriod() {
return this.timePeriod;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Budget(java.lang.String name) {
this(name, BudgetArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Budget(java.lang.String name, BudgetArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Budget(java.lang.String name, BudgetArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:costmanagement:Budget", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Budget(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:costmanagement:Budget", name, null, makeResourceOptions(options, id), false);
}
private static BudgetArgs makeArgs(BudgetArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? BudgetArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:costmanagement/v20190401preview:Budget").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20230401preview:Budget").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20230801:Budget").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20230901:Budget").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20231101:Budget").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Budget get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Budget(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy