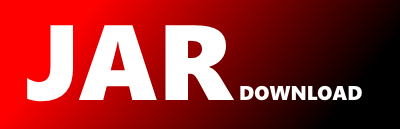
com.pulumi.azurenative.customerinsights.outputs.GetKpiResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.customerinsights.outputs;
import com.pulumi.azurenative.customerinsights.outputs.KpiAliasResponse;
import com.pulumi.azurenative.customerinsights.outputs.KpiExtractResponse;
import com.pulumi.azurenative.customerinsights.outputs.KpiGroupByMetadataResponse;
import com.pulumi.azurenative.customerinsights.outputs.KpiParticipantProfilesMetadataResponse;
import com.pulumi.azurenative.customerinsights.outputs.KpiThresholdsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetKpiResult {
/**
* @return The aliases.
*
*/
private @Nullable List aliases;
/**
* @return The calculation window.
*
*/
private String calculationWindow;
/**
* @return Name of calculation window field.
*
*/
private @Nullable String calculationWindowFieldName;
/**
* @return Localized description for the KPI.
*
*/
private @Nullable Map description;
/**
* @return Localized display name for the KPI.
*
*/
private @Nullable Map displayName;
/**
* @return The mapping entity type.
*
*/
private String entityType;
/**
* @return The mapping entity name.
*
*/
private String entityTypeName;
/**
* @return The computation expression for the KPI.
*
*/
private String expression;
/**
* @return The KPI extracts.
*
*/
private @Nullable List extracts;
/**
* @return The filter expression for the KPI.
*
*/
private @Nullable String filter;
/**
* @return The computation function for the KPI.
*
*/
private String function;
/**
* @return the group by properties for the KPI.
*
*/
private @Nullable List groupBy;
/**
* @return The KPI GroupByMetadata.
*
*/
private List groupByMetadata;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return The KPI name.
*
*/
private String kpiName;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return The participant profiles.
*
*/
private List participantProfilesMetadata;
/**
* @return Provisioning state.
*
*/
private String provisioningState;
/**
* @return The hub name.
*
*/
private String tenantId;
/**
* @return The KPI thresholds.
*
*/
private @Nullable KpiThresholdsResponse thresHolds;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return The unit of measurement for the KPI.
*
*/
private @Nullable String unit;
private GetKpiResult() {}
/**
* @return The aliases.
*
*/
public List aliases() {
return this.aliases == null ? List.of() : this.aliases;
}
/**
* @return The calculation window.
*
*/
public String calculationWindow() {
return this.calculationWindow;
}
/**
* @return Name of calculation window field.
*
*/
public Optional calculationWindowFieldName() {
return Optional.ofNullable(this.calculationWindowFieldName);
}
/**
* @return Localized description for the KPI.
*
*/
public Map description() {
return this.description == null ? Map.of() : this.description;
}
/**
* @return Localized display name for the KPI.
*
*/
public Map displayName() {
return this.displayName == null ? Map.of() : this.displayName;
}
/**
* @return The mapping entity type.
*
*/
public String entityType() {
return this.entityType;
}
/**
* @return The mapping entity name.
*
*/
public String entityTypeName() {
return this.entityTypeName;
}
/**
* @return The computation expression for the KPI.
*
*/
public String expression() {
return this.expression;
}
/**
* @return The KPI extracts.
*
*/
public List extracts() {
return this.extracts == null ? List.of() : this.extracts;
}
/**
* @return The filter expression for the KPI.
*
*/
public Optional filter() {
return Optional.ofNullable(this.filter);
}
/**
* @return The computation function for the KPI.
*
*/
public String function() {
return this.function;
}
/**
* @return the group by properties for the KPI.
*
*/
public List groupBy() {
return this.groupBy == null ? List.of() : this.groupBy;
}
/**
* @return The KPI GroupByMetadata.
*
*/
public List groupByMetadata() {
return this.groupByMetadata;
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return The KPI name.
*
*/
public String kpiName() {
return this.kpiName;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The participant profiles.
*
*/
public List participantProfilesMetadata() {
return this.participantProfilesMetadata;
}
/**
* @return Provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The hub name.
*
*/
public String tenantId() {
return this.tenantId;
}
/**
* @return The KPI thresholds.
*
*/
public Optional thresHolds() {
return Optional.ofNullable(this.thresHolds);
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return The unit of measurement for the KPI.
*
*/
public Optional unit() {
return Optional.ofNullable(this.unit);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetKpiResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List aliases;
private String calculationWindow;
private @Nullable String calculationWindowFieldName;
private @Nullable Map description;
private @Nullable Map displayName;
private String entityType;
private String entityTypeName;
private String expression;
private @Nullable List extracts;
private @Nullable String filter;
private String function;
private @Nullable List groupBy;
private List groupByMetadata;
private String id;
private String kpiName;
private String name;
private List participantProfilesMetadata;
private String provisioningState;
private String tenantId;
private @Nullable KpiThresholdsResponse thresHolds;
private String type;
private @Nullable String unit;
public Builder() {}
public Builder(GetKpiResult defaults) {
Objects.requireNonNull(defaults);
this.aliases = defaults.aliases;
this.calculationWindow = defaults.calculationWindow;
this.calculationWindowFieldName = defaults.calculationWindowFieldName;
this.description = defaults.description;
this.displayName = defaults.displayName;
this.entityType = defaults.entityType;
this.entityTypeName = defaults.entityTypeName;
this.expression = defaults.expression;
this.extracts = defaults.extracts;
this.filter = defaults.filter;
this.function = defaults.function;
this.groupBy = defaults.groupBy;
this.groupByMetadata = defaults.groupByMetadata;
this.id = defaults.id;
this.kpiName = defaults.kpiName;
this.name = defaults.name;
this.participantProfilesMetadata = defaults.participantProfilesMetadata;
this.provisioningState = defaults.provisioningState;
this.tenantId = defaults.tenantId;
this.thresHolds = defaults.thresHolds;
this.type = defaults.type;
this.unit = defaults.unit;
}
@CustomType.Setter
public Builder aliases(@Nullable List aliases) {
this.aliases = aliases;
return this;
}
public Builder aliases(KpiAliasResponse... aliases) {
return aliases(List.of(aliases));
}
@CustomType.Setter
public Builder calculationWindow(String calculationWindow) {
if (calculationWindow == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "calculationWindow");
}
this.calculationWindow = calculationWindow;
return this;
}
@CustomType.Setter
public Builder calculationWindowFieldName(@Nullable String calculationWindowFieldName) {
this.calculationWindowFieldName = calculationWindowFieldName;
return this;
}
@CustomType.Setter
public Builder description(@Nullable Map description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder displayName(@Nullable Map displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder entityType(String entityType) {
if (entityType == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "entityType");
}
this.entityType = entityType;
return this;
}
@CustomType.Setter
public Builder entityTypeName(String entityTypeName) {
if (entityTypeName == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "entityTypeName");
}
this.entityTypeName = entityTypeName;
return this;
}
@CustomType.Setter
public Builder expression(String expression) {
if (expression == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "expression");
}
this.expression = expression;
return this;
}
@CustomType.Setter
public Builder extracts(@Nullable List extracts) {
this.extracts = extracts;
return this;
}
public Builder extracts(KpiExtractResponse... extracts) {
return extracts(List.of(extracts));
}
@CustomType.Setter
public Builder filter(@Nullable String filter) {
this.filter = filter;
return this;
}
@CustomType.Setter
public Builder function(String function) {
if (function == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "function");
}
this.function = function;
return this;
}
@CustomType.Setter
public Builder groupBy(@Nullable List groupBy) {
this.groupBy = groupBy;
return this;
}
public Builder groupBy(String... groupBy) {
return groupBy(List.of(groupBy));
}
@CustomType.Setter
public Builder groupByMetadata(List groupByMetadata) {
if (groupByMetadata == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "groupByMetadata");
}
this.groupByMetadata = groupByMetadata;
return this;
}
public Builder groupByMetadata(KpiGroupByMetadataResponse... groupByMetadata) {
return groupByMetadata(List.of(groupByMetadata));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kpiName(String kpiName) {
if (kpiName == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "kpiName");
}
this.kpiName = kpiName;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder participantProfilesMetadata(List participantProfilesMetadata) {
if (participantProfilesMetadata == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "participantProfilesMetadata");
}
this.participantProfilesMetadata = participantProfilesMetadata;
return this;
}
public Builder participantProfilesMetadata(KpiParticipantProfilesMetadataResponse... participantProfilesMetadata) {
return participantProfilesMetadata(List.of(participantProfilesMetadata));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder tenantId(String tenantId) {
if (tenantId == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "tenantId");
}
this.tenantId = tenantId;
return this;
}
@CustomType.Setter
public Builder thresHolds(@Nullable KpiThresholdsResponse thresHolds) {
this.thresHolds = thresHolds;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetKpiResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder unit(@Nullable String unit) {
this.unit = unit;
return this;
}
public GetKpiResult build() {
final var _resultValue = new GetKpiResult();
_resultValue.aliases = aliases;
_resultValue.calculationWindow = calculationWindow;
_resultValue.calculationWindowFieldName = calculationWindowFieldName;
_resultValue.description = description;
_resultValue.displayName = displayName;
_resultValue.entityType = entityType;
_resultValue.entityTypeName = entityTypeName;
_resultValue.expression = expression;
_resultValue.extracts = extracts;
_resultValue.filter = filter;
_resultValue.function = function;
_resultValue.groupBy = groupBy;
_resultValue.groupByMetadata = groupByMetadata;
_resultValue.id = id;
_resultValue.kpiName = kpiName;
_resultValue.name = name;
_resultValue.participantProfilesMetadata = participantProfilesMetadata;
_resultValue.provisioningState = provisioningState;
_resultValue.tenantId = tenantId;
_resultValue.thresHolds = thresHolds;
_resultValue.type = type;
_resultValue.unit = unit;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy