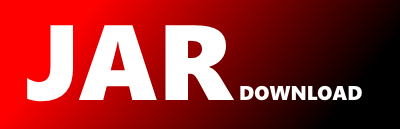
com.pulumi.azurenative.databox.outputs.DataBoxDiskGranularCopyProgressResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databox.outputs;
import com.pulumi.azurenative.databox.outputs.CloudErrorResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class DataBoxDiskGranularCopyProgressResponse {
/**
* @return Id of the account where the data needs to be uploaded.
*
*/
private String accountId;
/**
* @return Available actions on the job.
*
*/
private List actions;
/**
* @return To indicate bytes transferred.
*
*/
private Double bytesProcessed;
/**
* @return The Status of the copy
*
*/
private String copyStatus;
/**
* @return Data Account Type.
*
*/
private String dataAccountType;
/**
* @return To indicate directories errored out in the job.
*
*/
private Double directoriesErroredOut;
/**
* @return Error, if any, in the stage
*
*/
private CloudErrorResponse error;
/**
* @return Number of files which could not be copied
*
*/
private Double filesErroredOut;
/**
* @return Number of files processed
*
*/
private Double filesProcessed;
/**
* @return To indicate directories renamed
*
*/
private Double invalidDirectoriesProcessed;
/**
* @return Total amount of data not adhering to azure naming conventions which were processed by automatic renaming
*
*/
private Double invalidFileBytesUploaded;
/**
* @return Number of files not adhering to azure naming conventions which were processed by automatic renaming
*
*/
private Double invalidFilesProcessed;
/**
* @return To indicate if enumeration of data is in progress.
* Until this is true, the TotalBytesToProcess may not be valid.
*
*/
private Boolean isEnumerationInProgress;
/**
* @return Number of folders not adhering to azure naming conventions which were processed by automatic renaming
*
*/
private Double renamedContainerCount;
/**
* @return Disk Serial Number.
*
*/
private String serialNumber;
/**
* @return Name of the storage account. This will be empty for data account types other than storage account.
*
*/
private String storageAccountName;
/**
* @return Total amount of data to be processed by the job.
*
*/
private Double totalBytesToProcess;
/**
* @return Total files to process
*
*/
private Double totalFilesToProcess;
/**
* @return Transfer type of data
*
*/
private String transferType;
private DataBoxDiskGranularCopyProgressResponse() {}
/**
* @return Id of the account where the data needs to be uploaded.
*
*/
public String accountId() {
return this.accountId;
}
/**
* @return Available actions on the job.
*
*/
public List actions() {
return this.actions;
}
/**
* @return To indicate bytes transferred.
*
*/
public Double bytesProcessed() {
return this.bytesProcessed;
}
/**
* @return The Status of the copy
*
*/
public String copyStatus() {
return this.copyStatus;
}
/**
* @return Data Account Type.
*
*/
public String dataAccountType() {
return this.dataAccountType;
}
/**
* @return To indicate directories errored out in the job.
*
*/
public Double directoriesErroredOut() {
return this.directoriesErroredOut;
}
/**
* @return Error, if any, in the stage
*
*/
public CloudErrorResponse error() {
return this.error;
}
/**
* @return Number of files which could not be copied
*
*/
public Double filesErroredOut() {
return this.filesErroredOut;
}
/**
* @return Number of files processed
*
*/
public Double filesProcessed() {
return this.filesProcessed;
}
/**
* @return To indicate directories renamed
*
*/
public Double invalidDirectoriesProcessed() {
return this.invalidDirectoriesProcessed;
}
/**
* @return Total amount of data not adhering to azure naming conventions which were processed by automatic renaming
*
*/
public Double invalidFileBytesUploaded() {
return this.invalidFileBytesUploaded;
}
/**
* @return Number of files not adhering to azure naming conventions which were processed by automatic renaming
*
*/
public Double invalidFilesProcessed() {
return this.invalidFilesProcessed;
}
/**
* @return To indicate if enumeration of data is in progress.
* Until this is true, the TotalBytesToProcess may not be valid.
*
*/
public Boolean isEnumerationInProgress() {
return this.isEnumerationInProgress;
}
/**
* @return Number of folders not adhering to azure naming conventions which were processed by automatic renaming
*
*/
public Double renamedContainerCount() {
return this.renamedContainerCount;
}
/**
* @return Disk Serial Number.
*
*/
public String serialNumber() {
return this.serialNumber;
}
/**
* @return Name of the storage account. This will be empty for data account types other than storage account.
*
*/
public String storageAccountName() {
return this.storageAccountName;
}
/**
* @return Total amount of data to be processed by the job.
*
*/
public Double totalBytesToProcess() {
return this.totalBytesToProcess;
}
/**
* @return Total files to process
*
*/
public Double totalFilesToProcess() {
return this.totalFilesToProcess;
}
/**
* @return Transfer type of data
*
*/
public String transferType() {
return this.transferType;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DataBoxDiskGranularCopyProgressResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String accountId;
private List actions;
private Double bytesProcessed;
private String copyStatus;
private String dataAccountType;
private Double directoriesErroredOut;
private CloudErrorResponse error;
private Double filesErroredOut;
private Double filesProcessed;
private Double invalidDirectoriesProcessed;
private Double invalidFileBytesUploaded;
private Double invalidFilesProcessed;
private Boolean isEnumerationInProgress;
private Double renamedContainerCount;
private String serialNumber;
private String storageAccountName;
private Double totalBytesToProcess;
private Double totalFilesToProcess;
private String transferType;
public Builder() {}
public Builder(DataBoxDiskGranularCopyProgressResponse defaults) {
Objects.requireNonNull(defaults);
this.accountId = defaults.accountId;
this.actions = defaults.actions;
this.bytesProcessed = defaults.bytesProcessed;
this.copyStatus = defaults.copyStatus;
this.dataAccountType = defaults.dataAccountType;
this.directoriesErroredOut = defaults.directoriesErroredOut;
this.error = defaults.error;
this.filesErroredOut = defaults.filesErroredOut;
this.filesProcessed = defaults.filesProcessed;
this.invalidDirectoriesProcessed = defaults.invalidDirectoriesProcessed;
this.invalidFileBytesUploaded = defaults.invalidFileBytesUploaded;
this.invalidFilesProcessed = defaults.invalidFilesProcessed;
this.isEnumerationInProgress = defaults.isEnumerationInProgress;
this.renamedContainerCount = defaults.renamedContainerCount;
this.serialNumber = defaults.serialNumber;
this.storageAccountName = defaults.storageAccountName;
this.totalBytesToProcess = defaults.totalBytesToProcess;
this.totalFilesToProcess = defaults.totalFilesToProcess;
this.transferType = defaults.transferType;
}
@CustomType.Setter
public Builder accountId(String accountId) {
if (accountId == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "accountId");
}
this.accountId = accountId;
return this;
}
@CustomType.Setter
public Builder actions(List actions) {
if (actions == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "actions");
}
this.actions = actions;
return this;
}
public Builder actions(String... actions) {
return actions(List.of(actions));
}
@CustomType.Setter
public Builder bytesProcessed(Double bytesProcessed) {
if (bytesProcessed == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "bytesProcessed");
}
this.bytesProcessed = bytesProcessed;
return this;
}
@CustomType.Setter
public Builder copyStatus(String copyStatus) {
if (copyStatus == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "copyStatus");
}
this.copyStatus = copyStatus;
return this;
}
@CustomType.Setter
public Builder dataAccountType(String dataAccountType) {
if (dataAccountType == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "dataAccountType");
}
this.dataAccountType = dataAccountType;
return this;
}
@CustomType.Setter
public Builder directoriesErroredOut(Double directoriesErroredOut) {
if (directoriesErroredOut == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "directoriesErroredOut");
}
this.directoriesErroredOut = directoriesErroredOut;
return this;
}
@CustomType.Setter
public Builder error(CloudErrorResponse error) {
if (error == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "error");
}
this.error = error;
return this;
}
@CustomType.Setter
public Builder filesErroredOut(Double filesErroredOut) {
if (filesErroredOut == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "filesErroredOut");
}
this.filesErroredOut = filesErroredOut;
return this;
}
@CustomType.Setter
public Builder filesProcessed(Double filesProcessed) {
if (filesProcessed == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "filesProcessed");
}
this.filesProcessed = filesProcessed;
return this;
}
@CustomType.Setter
public Builder invalidDirectoriesProcessed(Double invalidDirectoriesProcessed) {
if (invalidDirectoriesProcessed == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "invalidDirectoriesProcessed");
}
this.invalidDirectoriesProcessed = invalidDirectoriesProcessed;
return this;
}
@CustomType.Setter
public Builder invalidFileBytesUploaded(Double invalidFileBytesUploaded) {
if (invalidFileBytesUploaded == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "invalidFileBytesUploaded");
}
this.invalidFileBytesUploaded = invalidFileBytesUploaded;
return this;
}
@CustomType.Setter
public Builder invalidFilesProcessed(Double invalidFilesProcessed) {
if (invalidFilesProcessed == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "invalidFilesProcessed");
}
this.invalidFilesProcessed = invalidFilesProcessed;
return this;
}
@CustomType.Setter
public Builder isEnumerationInProgress(Boolean isEnumerationInProgress) {
if (isEnumerationInProgress == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "isEnumerationInProgress");
}
this.isEnumerationInProgress = isEnumerationInProgress;
return this;
}
@CustomType.Setter
public Builder renamedContainerCount(Double renamedContainerCount) {
if (renamedContainerCount == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "renamedContainerCount");
}
this.renamedContainerCount = renamedContainerCount;
return this;
}
@CustomType.Setter
public Builder serialNumber(String serialNumber) {
if (serialNumber == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "serialNumber");
}
this.serialNumber = serialNumber;
return this;
}
@CustomType.Setter
public Builder storageAccountName(String storageAccountName) {
if (storageAccountName == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "storageAccountName");
}
this.storageAccountName = storageAccountName;
return this;
}
@CustomType.Setter
public Builder totalBytesToProcess(Double totalBytesToProcess) {
if (totalBytesToProcess == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "totalBytesToProcess");
}
this.totalBytesToProcess = totalBytesToProcess;
return this;
}
@CustomType.Setter
public Builder totalFilesToProcess(Double totalFilesToProcess) {
if (totalFilesToProcess == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "totalFilesToProcess");
}
this.totalFilesToProcess = totalFilesToProcess;
return this;
}
@CustomType.Setter
public Builder transferType(String transferType) {
if (transferType == null) {
throw new MissingRequiredPropertyException("DataBoxDiskGranularCopyProgressResponse", "transferType");
}
this.transferType = transferType;
return this;
}
public DataBoxDiskGranularCopyProgressResponse build() {
final var _resultValue = new DataBoxDiskGranularCopyProgressResponse();
_resultValue.accountId = accountId;
_resultValue.actions = actions;
_resultValue.bytesProcessed = bytesProcessed;
_resultValue.copyStatus = copyStatus;
_resultValue.dataAccountType = dataAccountType;
_resultValue.directoriesErroredOut = directoriesErroredOut;
_resultValue.error = error;
_resultValue.filesErroredOut = filesErroredOut;
_resultValue.filesProcessed = filesProcessed;
_resultValue.invalidDirectoriesProcessed = invalidDirectoriesProcessed;
_resultValue.invalidFileBytesUploaded = invalidFileBytesUploaded;
_resultValue.invalidFilesProcessed = invalidFilesProcessed;
_resultValue.isEnumerationInProgress = isEnumerationInProgress;
_resultValue.renamedContainerCount = renamedContainerCount;
_resultValue.serialNumber = serialNumber;
_resultValue.storageAccountName = storageAccountName;
_resultValue.totalBytesToProcess = totalBytesToProcess;
_resultValue.totalFilesToProcess = totalFilesToProcess;
_resultValue.transferType = transferType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy