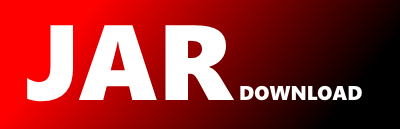
com.pulumi.azurenative.datafactory.inputs.CmdkeySetupArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.inputs;
import com.pulumi.azurenative.datafactory.inputs.AzureKeyVaultSecretReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.SecureStringArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
/**
* The custom setup of running cmdkey commands.
*
*/
public final class CmdkeySetupArgs extends com.pulumi.resources.ResourceArgs {
public static final CmdkeySetupArgs Empty = new CmdkeySetupArgs();
/**
* The password of data source access.
*
*/
@Import(name="password", required=true)
private Output> password;
/**
* @return The password of data source access.
*
*/
public Output> password() {
return this.password;
}
/**
* The server name of data source access. Type: string.
*
*/
@Import(name="targetName", required=true)
private Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy