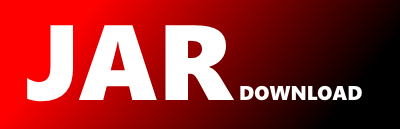
com.pulumi.azurenative.datafactory.inputs.HDInsightSparkActivityArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.inputs;
import com.pulumi.azurenative.datafactory.enums.ActivityOnInactiveMarkAs;
import com.pulumi.azurenative.datafactory.enums.ActivityState;
import com.pulumi.azurenative.datafactory.enums.HDInsightActivityDebugInfoOption;
import com.pulumi.azurenative.datafactory.inputs.ActivityDependencyArgs;
import com.pulumi.azurenative.datafactory.inputs.ActivityPolicyArgs;
import com.pulumi.azurenative.datafactory.inputs.LinkedServiceReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.UserPropertyArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* HDInsight Spark activity.
*
*/
public final class HDInsightSparkActivityArgs extends com.pulumi.resources.ResourceArgs {
public static final HDInsightSparkActivityArgs Empty = new HDInsightSparkActivityArgs();
/**
* The user-specified arguments to HDInsightSparkActivity.
*
*/
@Import(name="arguments")
private @Nullable Output> arguments;
/**
* @return The user-specified arguments to HDInsightSparkActivity.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy