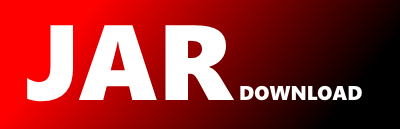
com.pulumi.azurenative.datafactory.inputs.WarehouseSourceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.inputs;
import com.pulumi.azurenative.datafactory.inputs.SqlPartitionSettingsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A copy activity Microsoft Fabric Warehouse source.
*
*/
public final class WarehouseSourceArgs extends com.pulumi.resources.ResourceArgs {
public static final WarehouseSourceArgs Empty = new WarehouseSourceArgs();
/**
* Specifies the additional columns to be added to source data. Type: array of objects(AdditionalColumns) (or Expression with resultType array of objects).
*
*/
@Import(name="additionalColumns")
private @Nullable Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy