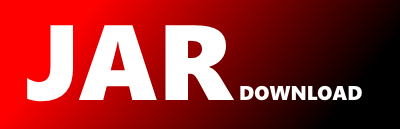
com.pulumi.azurenative.datafactory.inputs.WebBasicAuthenticationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.inputs;
import com.pulumi.azurenative.datafactory.inputs.AzureKeyVaultSecretReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.SecureStringArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
/**
* A WebLinkedService that uses basic authentication to communicate with an HTTP endpoint.
*
*/
public final class WebBasicAuthenticationArgs extends com.pulumi.resources.ResourceArgs {
public static final WebBasicAuthenticationArgs Empty = new WebBasicAuthenticationArgs();
/**
* Type of authentication used to connect to the web table source.
* Expected value is 'Basic'.
*
*/
@Import(name="authenticationType", required=true)
private Output authenticationType;
/**
* @return Type of authentication used to connect to the web table source.
* Expected value is 'Basic'.
*
*/
public Output authenticationType() {
return this.authenticationType;
}
/**
* The password for Basic authentication.
*
*/
@Import(name="password", required=true)
private Output> password;
/**
* @return The password for Basic authentication.
*
*/
public Output> password() {
return this.password;
}
/**
* The URL of the web service endpoint, e.g. https://www.microsoft.com . Type: string (or Expression with resultType string).
*
*/
@Import(name="url", required=true)
private Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy