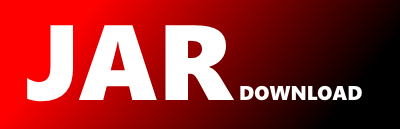
com.pulumi.azurenative.desktopvirtualization.outputs.AppAttachPackageInfoPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.desktopvirtualization.outputs;
import com.pulumi.azurenative.desktopvirtualization.outputs.MsixPackageApplicationsResponse;
import com.pulumi.azurenative.desktopvirtualization.outputs.MsixPackageDependenciesResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AppAttachPackageInfoPropertiesResponse {
/**
* @return Date certificate expires, found in the appxmanifest.xml.
*
*/
private @Nullable String certificateExpiry;
/**
* @return Certificate name found in the appxmanifest.xml.
*
*/
private @Nullable String certificateName;
/**
* @return User friendly Name to be displayed in the portal.
*
*/
private @Nullable String displayName;
/**
* @return VHD/CIM image path on Network Share.
*
*/
private @Nullable String imagePath;
/**
* @return Make this version of the package the active one across the hostpool.
*
*/
private @Nullable Boolean isActive;
/**
* @return Is package timestamped so it can ignore the certificate expiry date
*
*/
private @Nullable String isPackageTimestamped;
/**
* @return Specifies how to register Package in feed.
*
*/
private @Nullable Boolean isRegularRegistration;
/**
* @return Date Package was last updated, found in the appxmanifest.xml.
*
*/
private @Nullable String lastUpdated;
/**
* @return Alias of App Attach Package. Assigned at import time
*
*/
private @Nullable String packageAlias;
/**
* @return List of package applications.
*
*/
private @Nullable List packageApplications;
/**
* @return List of package dependencies.
*
*/
private @Nullable List packageDependencies;
/**
* @return Package Family Name from appxmanifest.xml. Contains Package Name and Publisher name.
*
*/
private @Nullable String packageFamilyName;
/**
* @return Package Full Name from appxmanifest.xml.
*
*/
private @Nullable String packageFullName;
/**
* @return Package Name from appxmanifest.xml.
*
*/
private @Nullable String packageName;
/**
* @return Relative Path to the package inside the image.
*
*/
private @Nullable String packageRelativePath;
/**
* @return Package Version found in the appxmanifest.xml.
*
*/
private @Nullable String version;
private AppAttachPackageInfoPropertiesResponse() {}
/**
* @return Date certificate expires, found in the appxmanifest.xml.
*
*/
public Optional certificateExpiry() {
return Optional.ofNullable(this.certificateExpiry);
}
/**
* @return Certificate name found in the appxmanifest.xml.
*
*/
public Optional certificateName() {
return Optional.ofNullable(this.certificateName);
}
/**
* @return User friendly Name to be displayed in the portal.
*
*/
public Optional displayName() {
return Optional.ofNullable(this.displayName);
}
/**
* @return VHD/CIM image path on Network Share.
*
*/
public Optional imagePath() {
return Optional.ofNullable(this.imagePath);
}
/**
* @return Make this version of the package the active one across the hostpool.
*
*/
public Optional isActive() {
return Optional.ofNullable(this.isActive);
}
/**
* @return Is package timestamped so it can ignore the certificate expiry date
*
*/
public Optional isPackageTimestamped() {
return Optional.ofNullable(this.isPackageTimestamped);
}
/**
* @return Specifies how to register Package in feed.
*
*/
public Optional isRegularRegistration() {
return Optional.ofNullable(this.isRegularRegistration);
}
/**
* @return Date Package was last updated, found in the appxmanifest.xml.
*
*/
public Optional lastUpdated() {
return Optional.ofNullable(this.lastUpdated);
}
/**
* @return Alias of App Attach Package. Assigned at import time
*
*/
public Optional packageAlias() {
return Optional.ofNullable(this.packageAlias);
}
/**
* @return List of package applications.
*
*/
public List packageApplications() {
return this.packageApplications == null ? List.of() : this.packageApplications;
}
/**
* @return List of package dependencies.
*
*/
public List packageDependencies() {
return this.packageDependencies == null ? List.of() : this.packageDependencies;
}
/**
* @return Package Family Name from appxmanifest.xml. Contains Package Name and Publisher name.
*
*/
public Optional packageFamilyName() {
return Optional.ofNullable(this.packageFamilyName);
}
/**
* @return Package Full Name from appxmanifest.xml.
*
*/
public Optional packageFullName() {
return Optional.ofNullable(this.packageFullName);
}
/**
* @return Package Name from appxmanifest.xml.
*
*/
public Optional packageName() {
return Optional.ofNullable(this.packageName);
}
/**
* @return Relative Path to the package inside the image.
*
*/
public Optional packageRelativePath() {
return Optional.ofNullable(this.packageRelativePath);
}
/**
* @return Package Version found in the appxmanifest.xml.
*
*/
public Optional version() {
return Optional.ofNullable(this.version);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AppAttachPackageInfoPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String certificateExpiry;
private @Nullable String certificateName;
private @Nullable String displayName;
private @Nullable String imagePath;
private @Nullable Boolean isActive;
private @Nullable String isPackageTimestamped;
private @Nullable Boolean isRegularRegistration;
private @Nullable String lastUpdated;
private @Nullable String packageAlias;
private @Nullable List packageApplications;
private @Nullable List packageDependencies;
private @Nullable String packageFamilyName;
private @Nullable String packageFullName;
private @Nullable String packageName;
private @Nullable String packageRelativePath;
private @Nullable String version;
public Builder() {}
public Builder(AppAttachPackageInfoPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.certificateExpiry = defaults.certificateExpiry;
this.certificateName = defaults.certificateName;
this.displayName = defaults.displayName;
this.imagePath = defaults.imagePath;
this.isActive = defaults.isActive;
this.isPackageTimestamped = defaults.isPackageTimestamped;
this.isRegularRegistration = defaults.isRegularRegistration;
this.lastUpdated = defaults.lastUpdated;
this.packageAlias = defaults.packageAlias;
this.packageApplications = defaults.packageApplications;
this.packageDependencies = defaults.packageDependencies;
this.packageFamilyName = defaults.packageFamilyName;
this.packageFullName = defaults.packageFullName;
this.packageName = defaults.packageName;
this.packageRelativePath = defaults.packageRelativePath;
this.version = defaults.version;
}
@CustomType.Setter
public Builder certificateExpiry(@Nullable String certificateExpiry) {
this.certificateExpiry = certificateExpiry;
return this;
}
@CustomType.Setter
public Builder certificateName(@Nullable String certificateName) {
this.certificateName = certificateName;
return this;
}
@CustomType.Setter
public Builder displayName(@Nullable String displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder imagePath(@Nullable String imagePath) {
this.imagePath = imagePath;
return this;
}
@CustomType.Setter
public Builder isActive(@Nullable Boolean isActive) {
this.isActive = isActive;
return this;
}
@CustomType.Setter
public Builder isPackageTimestamped(@Nullable String isPackageTimestamped) {
this.isPackageTimestamped = isPackageTimestamped;
return this;
}
@CustomType.Setter
public Builder isRegularRegistration(@Nullable Boolean isRegularRegistration) {
this.isRegularRegistration = isRegularRegistration;
return this;
}
@CustomType.Setter
public Builder lastUpdated(@Nullable String lastUpdated) {
this.lastUpdated = lastUpdated;
return this;
}
@CustomType.Setter
public Builder packageAlias(@Nullable String packageAlias) {
this.packageAlias = packageAlias;
return this;
}
@CustomType.Setter
public Builder packageApplications(@Nullable List packageApplications) {
this.packageApplications = packageApplications;
return this;
}
public Builder packageApplications(MsixPackageApplicationsResponse... packageApplications) {
return packageApplications(List.of(packageApplications));
}
@CustomType.Setter
public Builder packageDependencies(@Nullable List packageDependencies) {
this.packageDependencies = packageDependencies;
return this;
}
public Builder packageDependencies(MsixPackageDependenciesResponse... packageDependencies) {
return packageDependencies(List.of(packageDependencies));
}
@CustomType.Setter
public Builder packageFamilyName(@Nullable String packageFamilyName) {
this.packageFamilyName = packageFamilyName;
return this;
}
@CustomType.Setter
public Builder packageFullName(@Nullable String packageFullName) {
this.packageFullName = packageFullName;
return this;
}
@CustomType.Setter
public Builder packageName(@Nullable String packageName) {
this.packageName = packageName;
return this;
}
@CustomType.Setter
public Builder packageRelativePath(@Nullable String packageRelativePath) {
this.packageRelativePath = packageRelativePath;
return this;
}
@CustomType.Setter
public Builder version(@Nullable String version) {
this.version = version;
return this;
}
public AppAttachPackageInfoPropertiesResponse build() {
final var _resultValue = new AppAttachPackageInfoPropertiesResponse();
_resultValue.certificateExpiry = certificateExpiry;
_resultValue.certificateName = certificateName;
_resultValue.displayName = displayName;
_resultValue.imagePath = imagePath;
_resultValue.isActive = isActive;
_resultValue.isPackageTimestamped = isPackageTimestamped;
_resultValue.isRegularRegistration = isRegularRegistration;
_resultValue.lastUpdated = lastUpdated;
_resultValue.packageAlias = packageAlias;
_resultValue.packageApplications = packageApplications;
_resultValue.packageDependencies = packageDependencies;
_resultValue.packageFamilyName = packageFamilyName;
_resultValue.packageFullName = packageFullName;
_resultValue.packageName = packageName;
_resultValue.packageRelativePath = packageRelativePath;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy