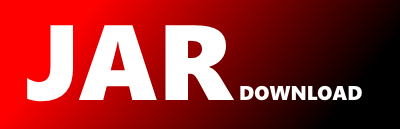
com.pulumi.azurenative.devhub.Workflow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devhub;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.devhub.WorkflowArgs;
import com.pulumi.azurenative.devhub.outputs.ACRResponse;
import com.pulumi.azurenative.devhub.outputs.DeploymentPropertiesResponse;
import com.pulumi.azurenative.devhub.outputs.GitHubWorkflowProfileResponseOidcCredentials;
import com.pulumi.azurenative.devhub.outputs.SystemDataResponse;
import com.pulumi.azurenative.devhub.outputs.WorkflowRunResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Resource representation of a workflow
* Azure REST API version: 2022-10-11-preview. Prior API version in Azure Native 1.x: 2022-04-01-preview.
*
* Other available API versions: 2023-08-01, 2024-05-01-preview, 2024-08-01-preview.
*
* ## Example Usage
* ### Create Workflow
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.devhub.Workflow;
* import com.pulumi.azurenative.devhub.WorkflowArgs;
* import com.pulumi.azurenative.devhub.inputs.ACRArgs;
* import com.pulumi.azurenative.devhub.inputs.DeploymentPropertiesArgs;
* import com.pulumi.azurenative.devhub.inputs.GitHubWorkflowProfileOidcCredentialsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var workflow = new Workflow("workflow", WorkflowArgs.builder()
* .acr(ACRArgs.builder()
* .acrRegistryName("registry1")
* .acrRepositoryName("repo1")
* .acrResourceGroup("resourceGroup1")
* .acrSubscriptionId("subscriptionId1")
* .build())
* .aksResourceId("/subscriptions/subscriptionId1/resourcegroups/resourceGroup1/providers/Microsoft.ContainerService/managedClusters/cluster1")
* .branchName("branch1")
* .deploymentProperties(DeploymentPropertiesArgs.builder()
* .kubeManifestLocations("/src/manifests/")
* .manifestType("kube")
* .overrides(Map.of("key1", "value1"))
* .build())
* .dockerBuildContext("repo1/src/")
* .dockerfile("repo1/images/Dockerfile")
* .location("location1")
* .namespace("namespace1")
* .oidcCredentials(GitHubWorkflowProfileOidcCredentialsArgs.builder()
* .azureClientId("12345678-3456-7890-5678-012345678901")
* .azureTenantId("66666666-3456-7890-5678-012345678901")
* .build())
* .repositoryName("repo1")
* .repositoryOwner("owner1")
* .resourceGroupName("resourceGroup1")
* .tags(Map.of("appname", "testApp"))
* .workflowName("workflow1")
* .build());
*
* }
* }
*
* }
*
* ### Create Workflow With Artifact Generation
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.devhub.Workflow;
* import com.pulumi.azurenative.devhub.WorkflowArgs;
* import com.pulumi.azurenative.devhub.inputs.ACRArgs;
* import com.pulumi.azurenative.devhub.inputs.DeploymentPropertiesArgs;
* import com.pulumi.azurenative.devhub.inputs.GitHubWorkflowProfileOidcCredentialsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var workflow = new Workflow("workflow", WorkflowArgs.builder()
* .acr(ACRArgs.builder()
* .acrRegistryName("registry1")
* .acrRepositoryName("repo1")
* .acrResourceGroup("resourceGroup1")
* .acrSubscriptionId("subscriptionId1")
* .build())
* .aksResourceId("/subscriptions/subscriptionId1/resourcegroups/resourceGroup1/providers/Microsoft.ContainerService/managedClusters/cluster1")
* .appName("my-app")
* .branchName("branch1")
* .deploymentProperties(DeploymentPropertiesArgs.builder()
* .kubeManifestLocations("/src/manifests/")
* .manifestType("kube")
* .overrides(Map.of("key1", "value1"))
* .build())
* .dockerBuildContext("repo1/src/")
* .dockerfile("repo1/images/Dockerfile")
* .dockerfileGenerationMode("enabled")
* .dockerfileOutputDirectory("./")
* .generationLanguage("javascript")
* .imageName("myimage")
* .imageTag("latest")
* .languageVersion("14")
* .location("location1")
* .manifestGenerationMode("enabled")
* .manifestOutputDirectory("./")
* .manifestType("kube")
* .oidcCredentials(GitHubWorkflowProfileOidcCredentialsArgs.builder()
* .azureClientId("12345678-3456-7890-5678-012345678901")
* .azureTenantId("66666666-3456-7890-5678-012345678901")
* .build())
* .port("80")
* .repositoryName("repo1")
* .repositoryOwner("owner1")
* .resourceGroupName("resourceGroup1")
* .tags(Map.of("appname", "testApp"))
* .workflowName("workflow1")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:devhub:Workflow workflow1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DevHub/workflows/{workflowName}
* ```
*
*/
@ResourceType(type="azure-native:devhub:Workflow")
public class Workflow extends com.pulumi.resources.CustomResource {
/**
* Information on the azure container registry
*
*/
@Export(name="acr", refs={ACRResponse.class}, tree="[0]")
private Output* @Nullable */ ACRResponse> acr;
/**
* @return Information on the azure container registry
*
*/
public Output> acr() {
return Codegen.optional(this.acr);
}
/**
* The Azure Kubernetes Cluster Resource the application will be deployed to.
*
*/
@Export(name="aksResourceId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> aksResourceId;
/**
* @return The Azure Kubernetes Cluster Resource the application will be deployed to.
*
*/
public Output> aksResourceId() {
return Codegen.optional(this.aksResourceId);
}
/**
* The name of the app.
*
*/
@Export(name="appName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> appName;
/**
* @return The name of the app.
*
*/
public Output> appName() {
return Codegen.optional(this.appName);
}
/**
* Determines the authorization status of requests.
*
*/
@Export(name="authStatus", refs={String.class}, tree="[0]")
private Output authStatus;
/**
* @return Determines the authorization status of requests.
*
*/
public Output authStatus() {
return this.authStatus;
}
/**
* Repository Branch Name
*
*/
@Export(name="branchName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> branchName;
/**
* @return Repository Branch Name
*
*/
public Output> branchName() {
return Codegen.optional(this.branchName);
}
/**
* The version of the language image used for building the code in the generated dockerfile.
*
*/
@Export(name="builderVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> builderVersion;
/**
* @return The version of the language image used for building the code in the generated dockerfile.
*
*/
public Output> builderVersion() {
return Codegen.optional(this.builderVersion);
}
@Export(name="deploymentProperties", refs={DeploymentPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ DeploymentPropertiesResponse> deploymentProperties;
public Output> deploymentProperties() {
return Codegen.optional(this.deploymentProperties);
}
/**
* Path to Dockerfile Build Context within the repository.
*
*/
@Export(name="dockerBuildContext", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dockerBuildContext;
/**
* @return Path to Dockerfile Build Context within the repository.
*
*/
public Output> dockerBuildContext() {
return Codegen.optional(this.dockerBuildContext);
}
/**
* Path to the Dockerfile within the repository.
*
*/
@Export(name="dockerfile", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dockerfile;
/**
* @return Path to the Dockerfile within the repository.
*
*/
public Output> dockerfile() {
return Codegen.optional(this.dockerfile);
}
/**
* The mode of generation to be used for generating Dockerfiles.
*
*/
@Export(name="dockerfileGenerationMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dockerfileGenerationMode;
/**
* @return The mode of generation to be used for generating Dockerfiles.
*
*/
public Output> dockerfileGenerationMode() {
return Codegen.optional(this.dockerfileGenerationMode);
}
/**
* The directory to output the generated Dockerfile to.
*
*/
@Export(name="dockerfileOutputDirectory", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dockerfileOutputDirectory;
/**
* @return The directory to output the generated Dockerfile to.
*
*/
public Output> dockerfileOutputDirectory() {
return Codegen.optional(this.dockerfileOutputDirectory);
}
/**
* The programming language used.
*
*/
@Export(name="generationLanguage", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> generationLanguage;
/**
* @return The programming language used.
*
*/
public Output> generationLanguage() {
return Codegen.optional(this.generationLanguage);
}
/**
* The name of the image to be generated.
*
*/
@Export(name="imageName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> imageName;
/**
* @return The name of the image to be generated.
*
*/
public Output> imageName() {
return Codegen.optional(this.imageName);
}
/**
* The tag to apply to the generated image.
*
*/
@Export(name="imageTag", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> imageTag;
/**
* @return The tag to apply to the generated image.
*
*/
public Output> imageTag() {
return Codegen.optional(this.imageTag);
}
/**
* The version of the language image used for execution in the generated dockerfile.
*
*/
@Export(name="languageVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> languageVersion;
/**
* @return The version of the language image used for execution in the generated dockerfile.
*
*/
public Output> languageVersion() {
return Codegen.optional(this.languageVersion);
}
@Export(name="lastWorkflowRun", refs={WorkflowRunResponse.class}, tree="[0]")
private Output* @Nullable */ WorkflowRunResponse> lastWorkflowRun;
public Output> lastWorkflowRun() {
return Codegen.optional(this.lastWorkflowRun);
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* The mode of generation to be used for generating Manifest.
*
*/
@Export(name="manifestGenerationMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> manifestGenerationMode;
/**
* @return The mode of generation to be used for generating Manifest.
*
*/
public Output> manifestGenerationMode() {
return Codegen.optional(this.manifestGenerationMode);
}
/**
* The directory to output the generated manifests to.
*
*/
@Export(name="manifestOutputDirectory", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> manifestOutputDirectory;
/**
* @return The directory to output the generated manifests to.
*
*/
public Output> manifestOutputDirectory() {
return Codegen.optional(this.manifestOutputDirectory);
}
/**
* Determines the type of manifests to be generated.
*
*/
@Export(name="manifestType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> manifestType;
/**
* @return Determines the type of manifests to be generated.
*
*/
public Output> manifestType() {
return Codegen.optional(this.manifestType);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Kubernetes namespace the application is deployed to.
*
*/
@Export(name="namespace", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> namespace;
/**
* @return Kubernetes namespace the application is deployed to.
*
*/
public Output> namespace() {
return Codegen.optional(this.namespace);
}
/**
* The fields needed for OIDC with GitHub.
*
*/
@Export(name="oidcCredentials", refs={GitHubWorkflowProfileResponseOidcCredentials.class}, tree="[0]")
private Output* @Nullable */ GitHubWorkflowProfileResponseOidcCredentials> oidcCredentials;
/**
* @return The fields needed for OIDC with GitHub.
*
*/
public Output> oidcCredentials() {
return Codegen.optional(this.oidcCredentials);
}
/**
* The port the application is exposed on.
*
*/
@Export(name="port", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> port;
/**
* @return The port the application is exposed on.
*
*/
public Output> port() {
return Codegen.optional(this.port);
}
/**
* The status of the Pull Request submitted against the users repository.
*
*/
@Export(name="prStatus", refs={String.class}, tree="[0]")
private Output prStatus;
/**
* @return The status of the Pull Request submitted against the users repository.
*
*/
public Output prStatus() {
return this.prStatus;
}
/**
* The URL to the Pull Request submitted against the users repository.
*
*/
@Export(name="prURL", refs={String.class}, tree="[0]")
private Output prURL;
/**
* @return The URL to the Pull Request submitted against the users repository.
*
*/
public Output prURL() {
return this.prURL;
}
/**
* The number associated with the submitted pull request.
*
*/
@Export(name="pullNumber", refs={Integer.class}, tree="[0]")
private Output pullNumber;
/**
* @return The number associated with the submitted pull request.
*
*/
public Output pullNumber() {
return this.pullNumber;
}
/**
* Repository Name
*
*/
@Export(name="repositoryName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> repositoryName;
/**
* @return Repository Name
*
*/
public Output> repositoryName() {
return Codegen.optional(this.repositoryName);
}
/**
* Repository Owner
*
*/
@Export(name="repositoryOwner", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> repositoryOwner;
/**
* @return Repository Owner
*
*/
public Output> repositoryOwner() {
return Codegen.optional(this.repositoryOwner);
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Workflow(java.lang.String name) {
this(name, WorkflowArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Workflow(java.lang.String name, WorkflowArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Workflow(java.lang.String name, WorkflowArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:devhub:Workflow", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Workflow(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:devhub:Workflow", name, null, makeResourceOptions(options, id), false);
}
private static WorkflowArgs makeArgs(WorkflowArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? WorkflowArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:devhub/v20220401preview:Workflow").build()),
Output.of(Alias.builder().type("azure-native:devhub/v20221011preview:Workflow").build()),
Output.of(Alias.builder().type("azure-native:devhub/v20230801:Workflow").build()),
Output.of(Alias.builder().type("azure-native:devhub/v20240501preview:Workflow").build()),
Output.of(Alias.builder().type("azure-native:devhub/v20240801preview:Workflow").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Workflow get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Workflow(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy