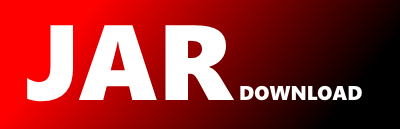
com.pulumi.azurenative.devhub.outputs.GetIacProfileResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devhub.outputs;
import com.pulumi.azurenative.devhub.outputs.IacTemplatePropertiesResponse;
import com.pulumi.azurenative.devhub.outputs.StagePropertiesResponse;
import com.pulumi.azurenative.devhub.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetIacProfileResult {
/**
* @return Determines the authorization status of requests.
*
*/
private String authStatus;
/**
* @return Repository Branch Name
*
*/
private @Nullable String branchName;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The status of the Pull Request submitted against the users repository.
*
*/
private String prStatus;
/**
* @return The number associated with the submitted pull request.
*
*/
private Integer pullNumber;
/**
* @return Repository Main Branch
*
*/
private @Nullable String repositoryMainBranch;
/**
* @return Repository Name
*
*/
private @Nullable String repositoryName;
/**
* @return Repository Owner
*
*/
private @Nullable String repositoryOwner;
private @Nullable List stages;
/**
* @return Terraform Storage Account Name
*
*/
private @Nullable String storageAccountName;
/**
* @return Terraform Storage Account Resource Group
*
*/
private @Nullable String storageAccountResourceGroup;
/**
* @return Terraform Storage Account Subscription
*
*/
private @Nullable String storageAccountSubscription;
/**
* @return Terraform Container Name
*
*/
private @Nullable String storageContainerName;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
private @Nullable List templates;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetIacProfileResult() {}
/**
* @return Determines the authorization status of requests.
*
*/
public String authStatus() {
return this.authStatus;
}
/**
* @return Repository Branch Name
*
*/
public Optional branchName() {
return Optional.ofNullable(this.branchName);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The status of the Pull Request submitted against the users repository.
*
*/
public String prStatus() {
return this.prStatus;
}
/**
* @return The number associated with the submitted pull request.
*
*/
public Integer pullNumber() {
return this.pullNumber;
}
/**
* @return Repository Main Branch
*
*/
public Optional repositoryMainBranch() {
return Optional.ofNullable(this.repositoryMainBranch);
}
/**
* @return Repository Name
*
*/
public Optional repositoryName() {
return Optional.ofNullable(this.repositoryName);
}
/**
* @return Repository Owner
*
*/
public Optional repositoryOwner() {
return Optional.ofNullable(this.repositoryOwner);
}
public List stages() {
return this.stages == null ? List.of() : this.stages;
}
/**
* @return Terraform Storage Account Name
*
*/
public Optional storageAccountName() {
return Optional.ofNullable(this.storageAccountName);
}
/**
* @return Terraform Storage Account Resource Group
*
*/
public Optional storageAccountResourceGroup() {
return Optional.ofNullable(this.storageAccountResourceGroup);
}
/**
* @return Terraform Storage Account Subscription
*
*/
public Optional storageAccountSubscription() {
return Optional.ofNullable(this.storageAccountSubscription);
}
/**
* @return Terraform Container Name
*
*/
public Optional storageContainerName() {
return Optional.ofNullable(this.storageContainerName);
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
public List templates() {
return this.templates == null ? List.of() : this.templates;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetIacProfileResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String authStatus;
private @Nullable String branchName;
private String etag;
private String id;
private String location;
private String name;
private String prStatus;
private Integer pullNumber;
private @Nullable String repositoryMainBranch;
private @Nullable String repositoryName;
private @Nullable String repositoryOwner;
private @Nullable List stages;
private @Nullable String storageAccountName;
private @Nullable String storageAccountResourceGroup;
private @Nullable String storageAccountSubscription;
private @Nullable String storageContainerName;
private SystemDataResponse systemData;
private @Nullable Map tags;
private @Nullable List templates;
private String type;
public Builder() {}
public Builder(GetIacProfileResult defaults) {
Objects.requireNonNull(defaults);
this.authStatus = defaults.authStatus;
this.branchName = defaults.branchName;
this.etag = defaults.etag;
this.id = defaults.id;
this.location = defaults.location;
this.name = defaults.name;
this.prStatus = defaults.prStatus;
this.pullNumber = defaults.pullNumber;
this.repositoryMainBranch = defaults.repositoryMainBranch;
this.repositoryName = defaults.repositoryName;
this.repositoryOwner = defaults.repositoryOwner;
this.stages = defaults.stages;
this.storageAccountName = defaults.storageAccountName;
this.storageAccountResourceGroup = defaults.storageAccountResourceGroup;
this.storageAccountSubscription = defaults.storageAccountSubscription;
this.storageContainerName = defaults.storageContainerName;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.templates = defaults.templates;
this.type = defaults.type;
}
@CustomType.Setter
public Builder authStatus(String authStatus) {
if (authStatus == null) {
throw new MissingRequiredPropertyException("GetIacProfileResult", "authStatus");
}
this.authStatus = authStatus;
return this;
}
@CustomType.Setter
public Builder branchName(@Nullable String branchName) {
this.branchName = branchName;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetIacProfileResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetIacProfileResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetIacProfileResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetIacProfileResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder prStatus(String prStatus) {
if (prStatus == null) {
throw new MissingRequiredPropertyException("GetIacProfileResult", "prStatus");
}
this.prStatus = prStatus;
return this;
}
@CustomType.Setter
public Builder pullNumber(Integer pullNumber) {
if (pullNumber == null) {
throw new MissingRequiredPropertyException("GetIacProfileResult", "pullNumber");
}
this.pullNumber = pullNumber;
return this;
}
@CustomType.Setter
public Builder repositoryMainBranch(@Nullable String repositoryMainBranch) {
this.repositoryMainBranch = repositoryMainBranch;
return this;
}
@CustomType.Setter
public Builder repositoryName(@Nullable String repositoryName) {
this.repositoryName = repositoryName;
return this;
}
@CustomType.Setter
public Builder repositoryOwner(@Nullable String repositoryOwner) {
this.repositoryOwner = repositoryOwner;
return this;
}
@CustomType.Setter
public Builder stages(@Nullable List stages) {
this.stages = stages;
return this;
}
public Builder stages(StagePropertiesResponse... stages) {
return stages(List.of(stages));
}
@CustomType.Setter
public Builder storageAccountName(@Nullable String storageAccountName) {
this.storageAccountName = storageAccountName;
return this;
}
@CustomType.Setter
public Builder storageAccountResourceGroup(@Nullable String storageAccountResourceGroup) {
this.storageAccountResourceGroup = storageAccountResourceGroup;
return this;
}
@CustomType.Setter
public Builder storageAccountSubscription(@Nullable String storageAccountSubscription) {
this.storageAccountSubscription = storageAccountSubscription;
return this;
}
@CustomType.Setter
public Builder storageContainerName(@Nullable String storageContainerName) {
this.storageContainerName = storageContainerName;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetIacProfileResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder templates(@Nullable List templates) {
this.templates = templates;
return this;
}
public Builder templates(IacTemplatePropertiesResponse... templates) {
return templates(List.of(templates));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetIacProfileResult", "type");
}
this.type = type;
return this;
}
public GetIacProfileResult build() {
final var _resultValue = new GetIacProfileResult();
_resultValue.authStatus = authStatus;
_resultValue.branchName = branchName;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.prStatus = prStatus;
_resultValue.pullNumber = pullNumber;
_resultValue.repositoryMainBranch = repositoryMainBranch;
_resultValue.repositoryName = repositoryName;
_resultValue.repositoryOwner = repositoryOwner;
_resultValue.stages = stages;
_resultValue.storageAccountName = storageAccountName;
_resultValue.storageAccountResourceGroup = storageAccountResourceGroup;
_resultValue.storageAccountSubscription = storageAccountSubscription;
_resultValue.storageContainerName = storageContainerName;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.templates = templates;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy