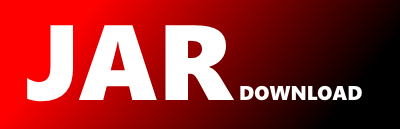
com.pulumi.azurenative.devtestlab.inputs.NetworkInterfacePropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devtestlab.inputs;
import com.pulumi.azurenative.devtestlab.inputs.SharedPublicIpAddressConfigurationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Properties of a network interface.
*
*/
public final class NetworkInterfacePropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final NetworkInterfacePropertiesArgs Empty = new NetworkInterfacePropertiesArgs();
/**
* The DNS name.
*
*/
@Import(name="dnsName")
private @Nullable Output dnsName;
/**
* @return The DNS name.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy