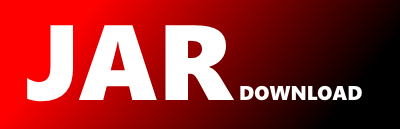
com.pulumi.azurenative.edgeorder.outputs.ChildConfigurationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.edgeorder.outputs;
import com.pulumi.azurenative.edgeorder.outputs.AvailabilityInformationResponse;
import com.pulumi.azurenative.edgeorder.outputs.CostInformationResponse;
import com.pulumi.azurenative.edgeorder.outputs.DescriptionResponse;
import com.pulumi.azurenative.edgeorder.outputs.DimensionsResponse;
import com.pulumi.azurenative.edgeorder.outputs.FilterablePropertyResponse;
import com.pulumi.azurenative.edgeorder.outputs.GroupedChildConfigurationsResponse;
import com.pulumi.azurenative.edgeorder.outputs.HierarchyInformationResponse;
import com.pulumi.azurenative.edgeorder.outputs.ImageInformationResponse;
import com.pulumi.azurenative.edgeorder.outputs.SpecificationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class ChildConfigurationResponse {
/**
* @return Availability information of the product system.
*
*/
private AvailabilityInformationResponse availabilityInformation;
/**
* @return Child configuration type.
*
*/
private String childConfigurationType;
/**
* @return Different types of child configurations which exist for this configuration, these can be used to populate the child configuration filter.
*
*/
private List childConfigurationTypes;
/**
* @return Cost information for the product system.
*
*/
private CostInformationResponse costInformation;
/**
* @return Description related to the product system.
*
*/
private DescriptionResponse description;
/**
* @return Dimensions of the configuration.
*
*/
private DimensionsResponse dimensions;
/**
* @return Display Name for the product system.
*
*/
private String displayName;
/**
* @return List of filters supported for a product.
*
*/
private List filterableProperties;
/**
* @return The entity responsible for fulfillment of the item at the given hierarchy level.
*
*/
private String fulfilledBy;
/**
* @return Child configurations present for the configuration after applying child configuration filter, grouped by the category name of the child configuration.
*
*/
private List groupedChildConfigurations;
/**
* @return Hierarchy information of a product.
*
*/
private HierarchyInformationResponse hierarchyInformation;
/**
* @return Image information for the product system.
*
*/
private List imageInformation;
/**
* @return Flag to indicate if the child configuration is part of the base configuration, which means the customer need not pass this configuration in OptInAdditionalConfigurations while placing an order, it will be shipped by default.
*
*/
private Boolean isPartOfBaseConfiguration;
/**
* @return Maximum quantity a customer can order while choosing this configuration.
*
*/
private Integer maximumQuantity;
/**
* @return Minimum quantity a customer can order while choosing this configuration.
*
*/
private Integer minimumQuantity;
/**
* @return Specifications of the configuration.
*
*/
private List specifications;
private ChildConfigurationResponse() {}
/**
* @return Availability information of the product system.
*
*/
public AvailabilityInformationResponse availabilityInformation() {
return this.availabilityInformation;
}
/**
* @return Child configuration type.
*
*/
public String childConfigurationType() {
return this.childConfigurationType;
}
/**
* @return Different types of child configurations which exist for this configuration, these can be used to populate the child configuration filter.
*
*/
public List childConfigurationTypes() {
return this.childConfigurationTypes;
}
/**
* @return Cost information for the product system.
*
*/
public CostInformationResponse costInformation() {
return this.costInformation;
}
/**
* @return Description related to the product system.
*
*/
public DescriptionResponse description() {
return this.description;
}
/**
* @return Dimensions of the configuration.
*
*/
public DimensionsResponse dimensions() {
return this.dimensions;
}
/**
* @return Display Name for the product system.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return List of filters supported for a product.
*
*/
public List filterableProperties() {
return this.filterableProperties;
}
/**
* @return The entity responsible for fulfillment of the item at the given hierarchy level.
*
*/
public String fulfilledBy() {
return this.fulfilledBy;
}
/**
* @return Child configurations present for the configuration after applying child configuration filter, grouped by the category name of the child configuration.
*
*/
public List groupedChildConfigurations() {
return this.groupedChildConfigurations;
}
/**
* @return Hierarchy information of a product.
*
*/
public HierarchyInformationResponse hierarchyInformation() {
return this.hierarchyInformation;
}
/**
* @return Image information for the product system.
*
*/
public List imageInformation() {
return this.imageInformation;
}
/**
* @return Flag to indicate if the child configuration is part of the base configuration, which means the customer need not pass this configuration in OptInAdditionalConfigurations while placing an order, it will be shipped by default.
*
*/
public Boolean isPartOfBaseConfiguration() {
return this.isPartOfBaseConfiguration;
}
/**
* @return Maximum quantity a customer can order while choosing this configuration.
*
*/
public Integer maximumQuantity() {
return this.maximumQuantity;
}
/**
* @return Minimum quantity a customer can order while choosing this configuration.
*
*/
public Integer minimumQuantity() {
return this.minimumQuantity;
}
/**
* @return Specifications of the configuration.
*
*/
public List specifications() {
return this.specifications;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ChildConfigurationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private AvailabilityInformationResponse availabilityInformation;
private String childConfigurationType;
private List childConfigurationTypes;
private CostInformationResponse costInformation;
private DescriptionResponse description;
private DimensionsResponse dimensions;
private String displayName;
private List filterableProperties;
private String fulfilledBy;
private List groupedChildConfigurations;
private HierarchyInformationResponse hierarchyInformation;
private List imageInformation;
private Boolean isPartOfBaseConfiguration;
private Integer maximumQuantity;
private Integer minimumQuantity;
private List specifications;
public Builder() {}
public Builder(ChildConfigurationResponse defaults) {
Objects.requireNonNull(defaults);
this.availabilityInformation = defaults.availabilityInformation;
this.childConfigurationType = defaults.childConfigurationType;
this.childConfigurationTypes = defaults.childConfigurationTypes;
this.costInformation = defaults.costInformation;
this.description = defaults.description;
this.dimensions = defaults.dimensions;
this.displayName = defaults.displayName;
this.filterableProperties = defaults.filterableProperties;
this.fulfilledBy = defaults.fulfilledBy;
this.groupedChildConfigurations = defaults.groupedChildConfigurations;
this.hierarchyInformation = defaults.hierarchyInformation;
this.imageInformation = defaults.imageInformation;
this.isPartOfBaseConfiguration = defaults.isPartOfBaseConfiguration;
this.maximumQuantity = defaults.maximumQuantity;
this.minimumQuantity = defaults.minimumQuantity;
this.specifications = defaults.specifications;
}
@CustomType.Setter
public Builder availabilityInformation(AvailabilityInformationResponse availabilityInformation) {
if (availabilityInformation == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "availabilityInformation");
}
this.availabilityInformation = availabilityInformation;
return this;
}
@CustomType.Setter
public Builder childConfigurationType(String childConfigurationType) {
if (childConfigurationType == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "childConfigurationType");
}
this.childConfigurationType = childConfigurationType;
return this;
}
@CustomType.Setter
public Builder childConfigurationTypes(List childConfigurationTypes) {
if (childConfigurationTypes == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "childConfigurationTypes");
}
this.childConfigurationTypes = childConfigurationTypes;
return this;
}
public Builder childConfigurationTypes(String... childConfigurationTypes) {
return childConfigurationTypes(List.of(childConfigurationTypes));
}
@CustomType.Setter
public Builder costInformation(CostInformationResponse costInformation) {
if (costInformation == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "costInformation");
}
this.costInformation = costInformation;
return this;
}
@CustomType.Setter
public Builder description(DescriptionResponse description) {
if (description == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder dimensions(DimensionsResponse dimensions) {
if (dimensions == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "dimensions");
}
this.dimensions = dimensions;
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
if (displayName == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "displayName");
}
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder filterableProperties(List filterableProperties) {
if (filterableProperties == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "filterableProperties");
}
this.filterableProperties = filterableProperties;
return this;
}
public Builder filterableProperties(FilterablePropertyResponse... filterableProperties) {
return filterableProperties(List.of(filterableProperties));
}
@CustomType.Setter
public Builder fulfilledBy(String fulfilledBy) {
if (fulfilledBy == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "fulfilledBy");
}
this.fulfilledBy = fulfilledBy;
return this;
}
@CustomType.Setter
public Builder groupedChildConfigurations(List groupedChildConfigurations) {
if (groupedChildConfigurations == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "groupedChildConfigurations");
}
this.groupedChildConfigurations = groupedChildConfigurations;
return this;
}
public Builder groupedChildConfigurations(GroupedChildConfigurationsResponse... groupedChildConfigurations) {
return groupedChildConfigurations(List.of(groupedChildConfigurations));
}
@CustomType.Setter
public Builder hierarchyInformation(HierarchyInformationResponse hierarchyInformation) {
if (hierarchyInformation == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "hierarchyInformation");
}
this.hierarchyInformation = hierarchyInformation;
return this;
}
@CustomType.Setter
public Builder imageInformation(List imageInformation) {
if (imageInformation == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "imageInformation");
}
this.imageInformation = imageInformation;
return this;
}
public Builder imageInformation(ImageInformationResponse... imageInformation) {
return imageInformation(List.of(imageInformation));
}
@CustomType.Setter
public Builder isPartOfBaseConfiguration(Boolean isPartOfBaseConfiguration) {
if (isPartOfBaseConfiguration == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "isPartOfBaseConfiguration");
}
this.isPartOfBaseConfiguration = isPartOfBaseConfiguration;
return this;
}
@CustomType.Setter
public Builder maximumQuantity(Integer maximumQuantity) {
if (maximumQuantity == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "maximumQuantity");
}
this.maximumQuantity = maximumQuantity;
return this;
}
@CustomType.Setter
public Builder minimumQuantity(Integer minimumQuantity) {
if (minimumQuantity == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "minimumQuantity");
}
this.minimumQuantity = minimumQuantity;
return this;
}
@CustomType.Setter
public Builder specifications(List specifications) {
if (specifications == null) {
throw new MissingRequiredPropertyException("ChildConfigurationResponse", "specifications");
}
this.specifications = specifications;
return this;
}
public Builder specifications(SpecificationResponse... specifications) {
return specifications(List.of(specifications));
}
public ChildConfigurationResponse build() {
final var _resultValue = new ChildConfigurationResponse();
_resultValue.availabilityInformation = availabilityInformation;
_resultValue.childConfigurationType = childConfigurationType;
_resultValue.childConfigurationTypes = childConfigurationTypes;
_resultValue.costInformation = costInformation;
_resultValue.description = description;
_resultValue.dimensions = dimensions;
_resultValue.displayName = displayName;
_resultValue.filterableProperties = filterableProperties;
_resultValue.fulfilledBy = fulfilledBy;
_resultValue.groupedChildConfigurations = groupedChildConfigurations;
_resultValue.hierarchyInformation = hierarchyInformation;
_resultValue.imageInformation = imageInformation;
_resultValue.isPartOfBaseConfiguration = isPartOfBaseConfiguration;
_resultValue.maximumQuantity = maximumQuantity;
_resultValue.minimumQuantity = minimumQuantity;
_resultValue.specifications = specifications;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy