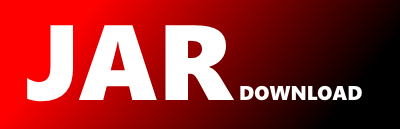
com.pulumi.azurenative.eventhub.EventhubFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.eventhub;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.eventhub.inputs.GetApplicationGroupArgs;
import com.pulumi.azurenative.eventhub.inputs.GetApplicationGroupPlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetClusterArgs;
import com.pulumi.azurenative.eventhub.inputs.GetClusterPlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetConsumerGroupArgs;
import com.pulumi.azurenative.eventhub.inputs.GetConsumerGroupPlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetDisasterRecoveryConfigArgs;
import com.pulumi.azurenative.eventhub.inputs.GetDisasterRecoveryConfigPlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetEventHubArgs;
import com.pulumi.azurenative.eventhub.inputs.GetEventHubAuthorizationRuleArgs;
import com.pulumi.azurenative.eventhub.inputs.GetEventHubAuthorizationRulePlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetEventHubPlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetNamespaceArgs;
import com.pulumi.azurenative.eventhub.inputs.GetNamespaceAuthorizationRuleArgs;
import com.pulumi.azurenative.eventhub.inputs.GetNamespaceAuthorizationRulePlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetNamespaceIpFilterRuleArgs;
import com.pulumi.azurenative.eventhub.inputs.GetNamespaceIpFilterRulePlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetNamespaceNetworkRuleSetArgs;
import com.pulumi.azurenative.eventhub.inputs.GetNamespaceNetworkRuleSetPlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetNamespacePlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetNamespaceVirtualNetworkRuleArgs;
import com.pulumi.azurenative.eventhub.inputs.GetNamespaceVirtualNetworkRulePlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.eventhub.inputs.GetPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.eventhub.inputs.GetSchemaRegistryArgs;
import com.pulumi.azurenative.eventhub.inputs.GetSchemaRegistryPlainArgs;
import com.pulumi.azurenative.eventhub.inputs.ListDisasterRecoveryConfigKeysArgs;
import com.pulumi.azurenative.eventhub.inputs.ListDisasterRecoveryConfigKeysPlainArgs;
import com.pulumi.azurenative.eventhub.inputs.ListEventHubKeysArgs;
import com.pulumi.azurenative.eventhub.inputs.ListEventHubKeysPlainArgs;
import com.pulumi.azurenative.eventhub.inputs.ListNamespaceKeysArgs;
import com.pulumi.azurenative.eventhub.inputs.ListNamespaceKeysPlainArgs;
import com.pulumi.azurenative.eventhub.outputs.GetApplicationGroupResult;
import com.pulumi.azurenative.eventhub.outputs.GetClusterResult;
import com.pulumi.azurenative.eventhub.outputs.GetConsumerGroupResult;
import com.pulumi.azurenative.eventhub.outputs.GetDisasterRecoveryConfigResult;
import com.pulumi.azurenative.eventhub.outputs.GetEventHubAuthorizationRuleResult;
import com.pulumi.azurenative.eventhub.outputs.GetEventHubResult;
import com.pulumi.azurenative.eventhub.outputs.GetNamespaceAuthorizationRuleResult;
import com.pulumi.azurenative.eventhub.outputs.GetNamespaceIpFilterRuleResult;
import com.pulumi.azurenative.eventhub.outputs.GetNamespaceNetworkRuleSetResult;
import com.pulumi.azurenative.eventhub.outputs.GetNamespaceResult;
import com.pulumi.azurenative.eventhub.outputs.GetNamespaceVirtualNetworkRuleResult;
import com.pulumi.azurenative.eventhub.outputs.GetPrivateEndpointConnectionResult;
import com.pulumi.azurenative.eventhub.outputs.GetSchemaRegistryResult;
import com.pulumi.azurenative.eventhub.outputs.ListDisasterRecoveryConfigKeysResult;
import com.pulumi.azurenative.eventhub.outputs.ListEventHubKeysResult;
import com.pulumi.azurenative.eventhub.outputs.ListNamespaceKeysResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class EventhubFunctions {
/**
* Gets an ApplicationGroup for a Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getApplicationGroup(GetApplicationGroupArgs args) {
return getApplicationGroup(args, InvokeOptions.Empty);
}
/**
* Gets an ApplicationGroup for a Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getApplicationGroupPlain(GetApplicationGroupPlainArgs args) {
return getApplicationGroupPlain(args, InvokeOptions.Empty);
}
/**
* Gets an ApplicationGroup for a Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getApplicationGroup(GetApplicationGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getApplicationGroup", TypeShape.of(GetApplicationGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an ApplicationGroup for a Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getApplicationGroupPlain(GetApplicationGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getApplicationGroup", TypeShape.of(GetApplicationGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the resource description of the specified Event Hubs Cluster.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getCluster(GetClusterArgs args) {
return getCluster(args, InvokeOptions.Empty);
}
/**
* Gets the resource description of the specified Event Hubs Cluster.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getClusterPlain(GetClusterPlainArgs args) {
return getClusterPlain(args, InvokeOptions.Empty);
}
/**
* Gets the resource description of the specified Event Hubs Cluster.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getCluster(GetClusterArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getCluster", TypeShape.of(GetClusterResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the resource description of the specified Event Hubs Cluster.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getClusterPlain(GetClusterPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getCluster", TypeShape.of(GetClusterResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a description for the specified consumer group.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getConsumerGroup(GetConsumerGroupArgs args) {
return getConsumerGroup(args, InvokeOptions.Empty);
}
/**
* Gets a description for the specified consumer group.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getConsumerGroupPlain(GetConsumerGroupPlainArgs args) {
return getConsumerGroupPlain(args, InvokeOptions.Empty);
}
/**
* Gets a description for the specified consumer group.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getConsumerGroup(GetConsumerGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getConsumerGroup", TypeShape.of(GetConsumerGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a description for the specified consumer group.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getConsumerGroupPlain(GetConsumerGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getConsumerGroup", TypeShape.of(GetConsumerGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves Alias(Disaster Recovery configuration) for primary or secondary namespace
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getDisasterRecoveryConfig(GetDisasterRecoveryConfigArgs args) {
return getDisasterRecoveryConfig(args, InvokeOptions.Empty);
}
/**
* Retrieves Alias(Disaster Recovery configuration) for primary or secondary namespace
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getDisasterRecoveryConfigPlain(GetDisasterRecoveryConfigPlainArgs args) {
return getDisasterRecoveryConfigPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves Alias(Disaster Recovery configuration) for primary or secondary namespace
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getDisasterRecoveryConfig(GetDisasterRecoveryConfigArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getDisasterRecoveryConfig", TypeShape.of(GetDisasterRecoveryConfigResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves Alias(Disaster Recovery configuration) for primary or secondary namespace
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getDisasterRecoveryConfigPlain(GetDisasterRecoveryConfigPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getDisasterRecoveryConfig", TypeShape.of(GetDisasterRecoveryConfigResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an Event Hubs description for the specified Event Hub.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getEventHub(GetEventHubArgs args) {
return getEventHub(args, InvokeOptions.Empty);
}
/**
* Gets an Event Hubs description for the specified Event Hub.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getEventHubPlain(GetEventHubPlainArgs args) {
return getEventHubPlain(args, InvokeOptions.Empty);
}
/**
* Gets an Event Hubs description for the specified Event Hub.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getEventHub(GetEventHubArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getEventHub", TypeShape.of(GetEventHubResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an Event Hubs description for the specified Event Hub.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getEventHubPlain(GetEventHubPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getEventHub", TypeShape.of(GetEventHubResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an AuthorizationRule for an Event Hub by rule name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getEventHubAuthorizationRule(GetEventHubAuthorizationRuleArgs args) {
return getEventHubAuthorizationRule(args, InvokeOptions.Empty);
}
/**
* Gets an AuthorizationRule for an Event Hub by rule name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getEventHubAuthorizationRulePlain(GetEventHubAuthorizationRulePlainArgs args) {
return getEventHubAuthorizationRulePlain(args, InvokeOptions.Empty);
}
/**
* Gets an AuthorizationRule for an Event Hub by rule name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getEventHubAuthorizationRule(GetEventHubAuthorizationRuleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getEventHubAuthorizationRule", TypeShape.of(GetEventHubAuthorizationRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an AuthorizationRule for an Event Hub by rule name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getEventHubAuthorizationRulePlain(GetEventHubAuthorizationRulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getEventHubAuthorizationRule", TypeShape.of(GetEventHubAuthorizationRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the description of the specified namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getNamespace(GetNamespaceArgs args) {
return getNamespace(args, InvokeOptions.Empty);
}
/**
* Gets the description of the specified namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getNamespacePlain(GetNamespacePlainArgs args) {
return getNamespacePlain(args, InvokeOptions.Empty);
}
/**
* Gets the description of the specified namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getNamespace(GetNamespaceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getNamespace", TypeShape.of(GetNamespaceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the description of the specified namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getNamespacePlain(GetNamespacePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getNamespace", TypeShape.of(GetNamespaceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an AuthorizationRule for a Namespace by rule name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getNamespaceAuthorizationRule(GetNamespaceAuthorizationRuleArgs args) {
return getNamespaceAuthorizationRule(args, InvokeOptions.Empty);
}
/**
* Gets an AuthorizationRule for a Namespace by rule name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getNamespaceAuthorizationRulePlain(GetNamespaceAuthorizationRulePlainArgs args) {
return getNamespaceAuthorizationRulePlain(args, InvokeOptions.Empty);
}
/**
* Gets an AuthorizationRule for a Namespace by rule name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getNamespaceAuthorizationRule(GetNamespaceAuthorizationRuleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getNamespaceAuthorizationRule", TypeShape.of(GetNamespaceAuthorizationRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an AuthorizationRule for a Namespace by rule name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getNamespaceAuthorizationRulePlain(GetNamespaceAuthorizationRulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getNamespaceAuthorizationRule", TypeShape.of(GetNamespaceAuthorizationRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an IpFilterRule for a Namespace by rule name.
* Azure REST API version: 2018-01-01-preview.
*
*/
public static Output getNamespaceIpFilterRule(GetNamespaceIpFilterRuleArgs args) {
return getNamespaceIpFilterRule(args, InvokeOptions.Empty);
}
/**
* Gets an IpFilterRule for a Namespace by rule name.
* Azure REST API version: 2018-01-01-preview.
*
*/
public static CompletableFuture getNamespaceIpFilterRulePlain(GetNamespaceIpFilterRulePlainArgs args) {
return getNamespaceIpFilterRulePlain(args, InvokeOptions.Empty);
}
/**
* Gets an IpFilterRule for a Namespace by rule name.
* Azure REST API version: 2018-01-01-preview.
*
*/
public static Output getNamespaceIpFilterRule(GetNamespaceIpFilterRuleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getNamespaceIpFilterRule", TypeShape.of(GetNamespaceIpFilterRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an IpFilterRule for a Namespace by rule name.
* Azure REST API version: 2018-01-01-preview.
*
*/
public static CompletableFuture getNamespaceIpFilterRulePlain(GetNamespaceIpFilterRulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getNamespaceIpFilterRule", TypeShape.of(GetNamespaceIpFilterRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets NetworkRuleSet for a Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getNamespaceNetworkRuleSet(GetNamespaceNetworkRuleSetArgs args) {
return getNamespaceNetworkRuleSet(args, InvokeOptions.Empty);
}
/**
* Gets NetworkRuleSet for a Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getNamespaceNetworkRuleSetPlain(GetNamespaceNetworkRuleSetPlainArgs args) {
return getNamespaceNetworkRuleSetPlain(args, InvokeOptions.Empty);
}
/**
* Gets NetworkRuleSet for a Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getNamespaceNetworkRuleSet(GetNamespaceNetworkRuleSetArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getNamespaceNetworkRuleSet", TypeShape.of(GetNamespaceNetworkRuleSetResult.class), args, Utilities.withVersion(options));
}
/**
* Gets NetworkRuleSet for a Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getNamespaceNetworkRuleSetPlain(GetNamespaceNetworkRuleSetPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getNamespaceNetworkRuleSet", TypeShape.of(GetNamespaceNetworkRuleSetResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an VirtualNetworkRule for a Namespace by rule name.
* Azure REST API version: 2018-01-01-preview.
*
*/
public static Output getNamespaceVirtualNetworkRule(GetNamespaceVirtualNetworkRuleArgs args) {
return getNamespaceVirtualNetworkRule(args, InvokeOptions.Empty);
}
/**
* Gets an VirtualNetworkRule for a Namespace by rule name.
* Azure REST API version: 2018-01-01-preview.
*
*/
public static CompletableFuture getNamespaceVirtualNetworkRulePlain(GetNamespaceVirtualNetworkRulePlainArgs args) {
return getNamespaceVirtualNetworkRulePlain(args, InvokeOptions.Empty);
}
/**
* Gets an VirtualNetworkRule for a Namespace by rule name.
* Azure REST API version: 2018-01-01-preview.
*
*/
public static Output getNamespaceVirtualNetworkRule(GetNamespaceVirtualNetworkRuleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getNamespaceVirtualNetworkRule", TypeShape.of(GetNamespaceVirtualNetworkRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an VirtualNetworkRule for a Namespace by rule name.
* Azure REST API version: 2018-01-01-preview.
*
*/
public static CompletableFuture getNamespaceVirtualNetworkRulePlain(GetNamespaceVirtualNetworkRulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getNamespaceVirtualNetworkRule", TypeShape.of(GetNamespaceVirtualNetworkRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a description for the specified Private Endpoint Connection name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args) {
return getPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Gets a description for the specified Private Endpoint Connection name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args) {
return getPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets a description for the specified Private Endpoint Connection name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a description for the specified Private Endpoint Connection name.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of an EventHub schema group.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getSchemaRegistry(GetSchemaRegistryArgs args) {
return getSchemaRegistry(args, InvokeOptions.Empty);
}
/**
* Gets the details of an EventHub schema group.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getSchemaRegistryPlain(GetSchemaRegistryPlainArgs args) {
return getSchemaRegistryPlain(args, InvokeOptions.Empty);
}
/**
* Gets the details of an EventHub schema group.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output getSchemaRegistry(GetSchemaRegistryArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:getSchemaRegistry", TypeShape.of(GetSchemaRegistryResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of an EventHub schema group.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getSchemaRegistryPlain(GetSchemaRegistryPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:getSchemaRegistry", TypeShape.of(GetSchemaRegistryResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the primary and secondary connection strings for the Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output listDisasterRecoveryConfigKeys(ListDisasterRecoveryConfigKeysArgs args) {
return listDisasterRecoveryConfigKeys(args, InvokeOptions.Empty);
}
/**
* Gets the primary and secondary connection strings for the Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture listDisasterRecoveryConfigKeysPlain(ListDisasterRecoveryConfigKeysPlainArgs args) {
return listDisasterRecoveryConfigKeysPlain(args, InvokeOptions.Empty);
}
/**
* Gets the primary and secondary connection strings for the Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output listDisasterRecoveryConfigKeys(ListDisasterRecoveryConfigKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:listDisasterRecoveryConfigKeys", TypeShape.of(ListDisasterRecoveryConfigKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the primary and secondary connection strings for the Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture listDisasterRecoveryConfigKeysPlain(ListDisasterRecoveryConfigKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:listDisasterRecoveryConfigKeys", TypeShape.of(ListDisasterRecoveryConfigKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the ACS and SAS connection strings for the Event Hub.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output listEventHubKeys(ListEventHubKeysArgs args) {
return listEventHubKeys(args, InvokeOptions.Empty);
}
/**
* Gets the ACS and SAS connection strings for the Event Hub.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture listEventHubKeysPlain(ListEventHubKeysPlainArgs args) {
return listEventHubKeysPlain(args, InvokeOptions.Empty);
}
/**
* Gets the ACS and SAS connection strings for the Event Hub.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output listEventHubKeys(ListEventHubKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:listEventHubKeys", TypeShape.of(ListEventHubKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the ACS and SAS connection strings for the Event Hub.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture listEventHubKeysPlain(ListEventHubKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:listEventHubKeys", TypeShape.of(ListEventHubKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the primary and secondary connection strings for the Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output listNamespaceKeys(ListNamespaceKeysArgs args) {
return listNamespaceKeys(args, InvokeOptions.Empty);
}
/**
* Gets the primary and secondary connection strings for the Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture listNamespaceKeysPlain(ListNamespaceKeysPlainArgs args) {
return listNamespaceKeysPlain(args, InvokeOptions.Empty);
}
/**
* Gets the primary and secondary connection strings for the Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static Output listNamespaceKeys(ListNamespaceKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:eventhub:listNamespaceKeys", TypeShape.of(ListNamespaceKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the primary and secondary connection strings for the Namespace.
* Azure REST API version: 2022-10-01-preview.
*
* Other available API versions: 2015-08-01, 2023-01-01-preview, 2024-01-01, 2024-05-01-preview.
*
*/
public static CompletableFuture listNamespaceKeysPlain(ListNamespaceKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:eventhub:listNamespaceKeys", TypeShape.of(ListNamespaceKeysResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy