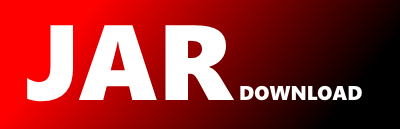
com.pulumi.azurenative.hdinsight.outputs.SecurityProfileResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hdinsight.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SecurityProfileResponse {
/**
* @return The resource ID of the user's Azure Active Directory Domain Service.
*
*/
private @Nullable String aaddsResourceId;
/**
* @return Optional. The Distinguished Names for cluster user groups
*
*/
private @Nullable List clusterUsersGroupDNs;
/**
* @return The directory type.
*
*/
private @Nullable String directoryType;
/**
* @return The organization's active directory domain.
*
*/
private @Nullable String domain;
/**
* @return The domain admin password.
*
*/
private @Nullable String domainUserPassword;
/**
* @return The domain user account that will have admin privileges on the cluster.
*
*/
private @Nullable String domainUsername;
/**
* @return The LDAPS protocol URLs to communicate with the Active Directory.
*
*/
private @Nullable List ldapsUrls;
/**
* @return User assigned identity that has permissions to read and create cluster-related artifacts in the user's AADDS.
*
*/
private @Nullable String msiResourceId;
/**
* @return The organizational unit within the Active Directory to place the cluster and service accounts.
*
*/
private @Nullable String organizationalUnitDN;
private SecurityProfileResponse() {}
/**
* @return The resource ID of the user's Azure Active Directory Domain Service.
*
*/
public Optional aaddsResourceId() {
return Optional.ofNullable(this.aaddsResourceId);
}
/**
* @return Optional. The Distinguished Names for cluster user groups
*
*/
public List clusterUsersGroupDNs() {
return this.clusterUsersGroupDNs == null ? List.of() : this.clusterUsersGroupDNs;
}
/**
* @return The directory type.
*
*/
public Optional directoryType() {
return Optional.ofNullable(this.directoryType);
}
/**
* @return The organization's active directory domain.
*
*/
public Optional domain() {
return Optional.ofNullable(this.domain);
}
/**
* @return The domain admin password.
*
*/
public Optional domainUserPassword() {
return Optional.ofNullable(this.domainUserPassword);
}
/**
* @return The domain user account that will have admin privileges on the cluster.
*
*/
public Optional domainUsername() {
return Optional.ofNullable(this.domainUsername);
}
/**
* @return The LDAPS protocol URLs to communicate with the Active Directory.
*
*/
public List ldapsUrls() {
return this.ldapsUrls == null ? List.of() : this.ldapsUrls;
}
/**
* @return User assigned identity that has permissions to read and create cluster-related artifacts in the user's AADDS.
*
*/
public Optional msiResourceId() {
return Optional.ofNullable(this.msiResourceId);
}
/**
* @return The organizational unit within the Active Directory to place the cluster and service accounts.
*
*/
public Optional organizationalUnitDN() {
return Optional.ofNullable(this.organizationalUnitDN);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SecurityProfileResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String aaddsResourceId;
private @Nullable List clusterUsersGroupDNs;
private @Nullable String directoryType;
private @Nullable String domain;
private @Nullable String domainUserPassword;
private @Nullable String domainUsername;
private @Nullable List ldapsUrls;
private @Nullable String msiResourceId;
private @Nullable String organizationalUnitDN;
public Builder() {}
public Builder(SecurityProfileResponse defaults) {
Objects.requireNonNull(defaults);
this.aaddsResourceId = defaults.aaddsResourceId;
this.clusterUsersGroupDNs = defaults.clusterUsersGroupDNs;
this.directoryType = defaults.directoryType;
this.domain = defaults.domain;
this.domainUserPassword = defaults.domainUserPassword;
this.domainUsername = defaults.domainUsername;
this.ldapsUrls = defaults.ldapsUrls;
this.msiResourceId = defaults.msiResourceId;
this.organizationalUnitDN = defaults.organizationalUnitDN;
}
@CustomType.Setter
public Builder aaddsResourceId(@Nullable String aaddsResourceId) {
this.aaddsResourceId = aaddsResourceId;
return this;
}
@CustomType.Setter
public Builder clusterUsersGroupDNs(@Nullable List clusterUsersGroupDNs) {
this.clusterUsersGroupDNs = clusterUsersGroupDNs;
return this;
}
public Builder clusterUsersGroupDNs(String... clusterUsersGroupDNs) {
return clusterUsersGroupDNs(List.of(clusterUsersGroupDNs));
}
@CustomType.Setter
public Builder directoryType(@Nullable String directoryType) {
this.directoryType = directoryType;
return this;
}
@CustomType.Setter
public Builder domain(@Nullable String domain) {
this.domain = domain;
return this;
}
@CustomType.Setter
public Builder domainUserPassword(@Nullable String domainUserPassword) {
this.domainUserPassword = domainUserPassword;
return this;
}
@CustomType.Setter
public Builder domainUsername(@Nullable String domainUsername) {
this.domainUsername = domainUsername;
return this;
}
@CustomType.Setter
public Builder ldapsUrls(@Nullable List ldapsUrls) {
this.ldapsUrls = ldapsUrls;
return this;
}
public Builder ldapsUrls(String... ldapsUrls) {
return ldapsUrls(List.of(ldapsUrls));
}
@CustomType.Setter
public Builder msiResourceId(@Nullable String msiResourceId) {
this.msiResourceId = msiResourceId;
return this;
}
@CustomType.Setter
public Builder organizationalUnitDN(@Nullable String organizationalUnitDN) {
this.organizationalUnitDN = organizationalUnitDN;
return this;
}
public SecurityProfileResponse build() {
final var _resultValue = new SecurityProfileResponse();
_resultValue.aaddsResourceId = aaddsResourceId;
_resultValue.clusterUsersGroupDNs = clusterUsersGroupDNs;
_resultValue.directoryType = directoryType;
_resultValue.domain = domain;
_resultValue.domainUserPassword = domainUserPassword;
_resultValue.domainUsername = domainUsername;
_resultValue.ldapsUrls = ldapsUrls;
_resultValue.msiResourceId = msiResourceId;
_resultValue.organizationalUnitDN = organizationalUnitDN;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy