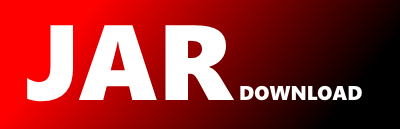
com.pulumi.azurenative.hybridcompute.outputs.GetMachineRunCommandResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hybridcompute.outputs;
import com.pulumi.azurenative.hybridcompute.outputs.MachineRunCommandInstanceViewResponse;
import com.pulumi.azurenative.hybridcompute.outputs.MachineRunCommandScriptSourceResponse;
import com.pulumi.azurenative.hybridcompute.outputs.RunCommandInputParameterResponse;
import com.pulumi.azurenative.hybridcompute.outputs.RunCommandManagedIdentityResponse;
import com.pulumi.azurenative.hybridcompute.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetMachineRunCommandResult {
/**
* @return Optional. If set to true, provisioning will complete as soon as script starts and will not wait for script to complete.
*
*/
private @Nullable Boolean asyncExecution;
/**
* @return User-assigned managed identity that has access to errorBlobUri storage blob. Use an empty object in case of system-assigned identity. Make sure managed identity has been given access to blob's container with 'Storage Blob Data Contributor' role assignment. In case of user-assigned identity, make sure you add it under VM's identity. For more info on managed identity and Run Command, refer https://aka.ms/ManagedIdentity and https://aka.ms/RunCommandManaged
*
*/
private @Nullable RunCommandManagedIdentityResponse errorBlobManagedIdentity;
/**
* @return Specifies the Azure storage blob where script error stream will be uploaded. Use a SAS URI with read, append, create, write access OR use managed identity to provide the VM access to the blob. Refer errorBlobManagedIdentity parameter.
*
*/
private @Nullable String errorBlobUri;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The machine run command instance view.
*
*/
private MachineRunCommandInstanceViewResponse instanceView;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return User-assigned managed identity that has access to outputBlobUri storage blob. Use an empty object in case of system-assigned identity. Make sure managed identity has been given access to blob's container with 'Storage Blob Data Contributor' role assignment. In case of user-assigned identity, make sure you add it under VM's identity. For more info on managed identity and Run Command, refer https://aka.ms/ManagedIdentity and https://aka.ms/RunCommandManaged
*
*/
private @Nullable RunCommandManagedIdentityResponse outputBlobManagedIdentity;
/**
* @return Specifies the Azure storage blob where script output stream will be uploaded. Use a SAS URI with read, append, create, write access OR use managed identity to provide the VM access to the blob. Refer outputBlobManagedIdentity parameter.
*
*/
private @Nullable String outputBlobUri;
/**
* @return The parameters used by the script.
*
*/
private @Nullable List parameters;
/**
* @return The parameters used by the script.
*
*/
private @Nullable List protectedParameters;
/**
* @return The provisioning state, which only appears in the response.
*
*/
private String provisioningState;
/**
* @return Specifies the user account password on the machine when executing the run command.
*
*/
private @Nullable String runAsPassword;
/**
* @return Specifies the user account on the machine when executing the run command.
*
*/
private @Nullable String runAsUser;
/**
* @return The source of the run command script.
*
*/
private @Nullable MachineRunCommandScriptSourceResponse source;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The timeout in seconds to execute the run command.
*
*/
private @Nullable Integer timeoutInSeconds;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetMachineRunCommandResult() {}
/**
* @return Optional. If set to true, provisioning will complete as soon as script starts and will not wait for script to complete.
*
*/
public Optional asyncExecution() {
return Optional.ofNullable(this.asyncExecution);
}
/**
* @return User-assigned managed identity that has access to errorBlobUri storage blob. Use an empty object in case of system-assigned identity. Make sure managed identity has been given access to blob's container with 'Storage Blob Data Contributor' role assignment. In case of user-assigned identity, make sure you add it under VM's identity. For more info on managed identity and Run Command, refer https://aka.ms/ManagedIdentity and https://aka.ms/RunCommandManaged
*
*/
public Optional errorBlobManagedIdentity() {
return Optional.ofNullable(this.errorBlobManagedIdentity);
}
/**
* @return Specifies the Azure storage blob where script error stream will be uploaded. Use a SAS URI with read, append, create, write access OR use managed identity to provide the VM access to the blob. Refer errorBlobManagedIdentity parameter.
*
*/
public Optional errorBlobUri() {
return Optional.ofNullable(this.errorBlobUri);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The machine run command instance view.
*
*/
public MachineRunCommandInstanceViewResponse instanceView() {
return this.instanceView;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return User-assigned managed identity that has access to outputBlobUri storage blob. Use an empty object in case of system-assigned identity. Make sure managed identity has been given access to blob's container with 'Storage Blob Data Contributor' role assignment. In case of user-assigned identity, make sure you add it under VM's identity. For more info on managed identity and Run Command, refer https://aka.ms/ManagedIdentity and https://aka.ms/RunCommandManaged
*
*/
public Optional outputBlobManagedIdentity() {
return Optional.ofNullable(this.outputBlobManagedIdentity);
}
/**
* @return Specifies the Azure storage blob where script output stream will be uploaded. Use a SAS URI with read, append, create, write access OR use managed identity to provide the VM access to the blob. Refer outputBlobManagedIdentity parameter.
*
*/
public Optional outputBlobUri() {
return Optional.ofNullable(this.outputBlobUri);
}
/**
* @return The parameters used by the script.
*
*/
public List parameters() {
return this.parameters == null ? List.of() : this.parameters;
}
/**
* @return The parameters used by the script.
*
*/
public List protectedParameters() {
return this.protectedParameters == null ? List.of() : this.protectedParameters;
}
/**
* @return The provisioning state, which only appears in the response.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Specifies the user account password on the machine when executing the run command.
*
*/
public Optional runAsPassword() {
return Optional.ofNullable(this.runAsPassword);
}
/**
* @return Specifies the user account on the machine when executing the run command.
*
*/
public Optional runAsUser() {
return Optional.ofNullable(this.runAsUser);
}
/**
* @return The source of the run command script.
*
*/
public Optional source() {
return Optional.ofNullable(this.source);
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The timeout in seconds to execute the run command.
*
*/
public Optional timeoutInSeconds() {
return Optional.ofNullable(this.timeoutInSeconds);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetMachineRunCommandResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean asyncExecution;
private @Nullable RunCommandManagedIdentityResponse errorBlobManagedIdentity;
private @Nullable String errorBlobUri;
private String id;
private MachineRunCommandInstanceViewResponse instanceView;
private String location;
private String name;
private @Nullable RunCommandManagedIdentityResponse outputBlobManagedIdentity;
private @Nullable String outputBlobUri;
private @Nullable List parameters;
private @Nullable List protectedParameters;
private String provisioningState;
private @Nullable String runAsPassword;
private @Nullable String runAsUser;
private @Nullable MachineRunCommandScriptSourceResponse source;
private SystemDataResponse systemData;
private @Nullable Map tags;
private @Nullable Integer timeoutInSeconds;
private String type;
public Builder() {}
public Builder(GetMachineRunCommandResult defaults) {
Objects.requireNonNull(defaults);
this.asyncExecution = defaults.asyncExecution;
this.errorBlobManagedIdentity = defaults.errorBlobManagedIdentity;
this.errorBlobUri = defaults.errorBlobUri;
this.id = defaults.id;
this.instanceView = defaults.instanceView;
this.location = defaults.location;
this.name = defaults.name;
this.outputBlobManagedIdentity = defaults.outputBlobManagedIdentity;
this.outputBlobUri = defaults.outputBlobUri;
this.parameters = defaults.parameters;
this.protectedParameters = defaults.protectedParameters;
this.provisioningState = defaults.provisioningState;
this.runAsPassword = defaults.runAsPassword;
this.runAsUser = defaults.runAsUser;
this.source = defaults.source;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.timeoutInSeconds = defaults.timeoutInSeconds;
this.type = defaults.type;
}
@CustomType.Setter
public Builder asyncExecution(@Nullable Boolean asyncExecution) {
this.asyncExecution = asyncExecution;
return this;
}
@CustomType.Setter
public Builder errorBlobManagedIdentity(@Nullable RunCommandManagedIdentityResponse errorBlobManagedIdentity) {
this.errorBlobManagedIdentity = errorBlobManagedIdentity;
return this;
}
@CustomType.Setter
public Builder errorBlobUri(@Nullable String errorBlobUri) {
this.errorBlobUri = errorBlobUri;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetMachineRunCommandResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder instanceView(MachineRunCommandInstanceViewResponse instanceView) {
if (instanceView == null) {
throw new MissingRequiredPropertyException("GetMachineRunCommandResult", "instanceView");
}
this.instanceView = instanceView;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetMachineRunCommandResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetMachineRunCommandResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder outputBlobManagedIdentity(@Nullable RunCommandManagedIdentityResponse outputBlobManagedIdentity) {
this.outputBlobManagedIdentity = outputBlobManagedIdentity;
return this;
}
@CustomType.Setter
public Builder outputBlobUri(@Nullable String outputBlobUri) {
this.outputBlobUri = outputBlobUri;
return this;
}
@CustomType.Setter
public Builder parameters(@Nullable List parameters) {
this.parameters = parameters;
return this;
}
public Builder parameters(RunCommandInputParameterResponse... parameters) {
return parameters(List.of(parameters));
}
@CustomType.Setter
public Builder protectedParameters(@Nullable List protectedParameters) {
this.protectedParameters = protectedParameters;
return this;
}
public Builder protectedParameters(RunCommandInputParameterResponse... protectedParameters) {
return protectedParameters(List.of(protectedParameters));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetMachineRunCommandResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder runAsPassword(@Nullable String runAsPassword) {
this.runAsPassword = runAsPassword;
return this;
}
@CustomType.Setter
public Builder runAsUser(@Nullable String runAsUser) {
this.runAsUser = runAsUser;
return this;
}
@CustomType.Setter
public Builder source(@Nullable MachineRunCommandScriptSourceResponse source) {
this.source = source;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetMachineRunCommandResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder timeoutInSeconds(@Nullable Integer timeoutInSeconds) {
this.timeoutInSeconds = timeoutInSeconds;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetMachineRunCommandResult", "type");
}
this.type = type;
return this;
}
public GetMachineRunCommandResult build() {
final var _resultValue = new GetMachineRunCommandResult();
_resultValue.asyncExecution = asyncExecution;
_resultValue.errorBlobManagedIdentity = errorBlobManagedIdentity;
_resultValue.errorBlobUri = errorBlobUri;
_resultValue.id = id;
_resultValue.instanceView = instanceView;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.outputBlobManagedIdentity = outputBlobManagedIdentity;
_resultValue.outputBlobUri = outputBlobUri;
_resultValue.parameters = parameters;
_resultValue.protectedParameters = protectedParameters;
_resultValue.provisioningState = provisioningState;
_resultValue.runAsPassword = runAsPassword;
_resultValue.runAsUser = runAsUser;
_resultValue.source = source;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.timeoutInSeconds = timeoutInSeconds;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy