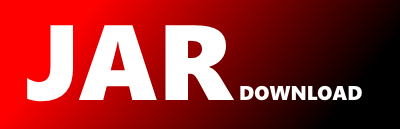
com.pulumi.azurenative.importexport.outputs.DriveStatusResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.importexport.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DriveStatusResponse {
/**
* @return The BitLocker key used to encrypt the drive.
*
*/
private @Nullable String bitLockerKey;
/**
* @return Bytes successfully transferred for the drive.
*
*/
private @Nullable Double bytesSucceeded;
/**
* @return Detailed status about the data transfer process. This field is not returned in the response until the drive is in the Transferring state.
*
*/
private @Nullable String copyStatus;
/**
* @return The drive header hash value.
*
*/
private @Nullable String driveHeaderHash;
/**
* @return The drive's hardware serial number, without spaces.
*
*/
private @Nullable String driveId;
/**
* @return A URI that points to the blob containing the error log for the data transfer operation.
*
*/
private @Nullable String errorLogUri;
/**
* @return The relative path of the manifest file on the drive.
*
*/
private @Nullable String manifestFile;
/**
* @return The Base16-encoded MD5 hash of the manifest file on the drive.
*
*/
private @Nullable String manifestHash;
/**
* @return A URI that points to the blob containing the drive manifest file.
*
*/
private @Nullable String manifestUri;
/**
* @return Percentage completed for the drive.
*
*/
private @Nullable Double percentComplete;
/**
* @return The drive's current state.
*
*/
private @Nullable String state;
/**
* @return A URI that points to the blob containing the verbose log for the data transfer operation.
*
*/
private @Nullable String verboseLogUri;
private DriveStatusResponse() {}
/**
* @return The BitLocker key used to encrypt the drive.
*
*/
public Optional bitLockerKey() {
return Optional.ofNullable(this.bitLockerKey);
}
/**
* @return Bytes successfully transferred for the drive.
*
*/
public Optional bytesSucceeded() {
return Optional.ofNullable(this.bytesSucceeded);
}
/**
* @return Detailed status about the data transfer process. This field is not returned in the response until the drive is in the Transferring state.
*
*/
public Optional copyStatus() {
return Optional.ofNullable(this.copyStatus);
}
/**
* @return The drive header hash value.
*
*/
public Optional driveHeaderHash() {
return Optional.ofNullable(this.driveHeaderHash);
}
/**
* @return The drive's hardware serial number, without spaces.
*
*/
public Optional driveId() {
return Optional.ofNullable(this.driveId);
}
/**
* @return A URI that points to the blob containing the error log for the data transfer operation.
*
*/
public Optional errorLogUri() {
return Optional.ofNullable(this.errorLogUri);
}
/**
* @return The relative path of the manifest file on the drive.
*
*/
public Optional manifestFile() {
return Optional.ofNullable(this.manifestFile);
}
/**
* @return The Base16-encoded MD5 hash of the manifest file on the drive.
*
*/
public Optional manifestHash() {
return Optional.ofNullable(this.manifestHash);
}
/**
* @return A URI that points to the blob containing the drive manifest file.
*
*/
public Optional manifestUri() {
return Optional.ofNullable(this.manifestUri);
}
/**
* @return Percentage completed for the drive.
*
*/
public Optional percentComplete() {
return Optional.ofNullable(this.percentComplete);
}
/**
* @return The drive's current state.
*
*/
public Optional state() {
return Optional.ofNullable(this.state);
}
/**
* @return A URI that points to the blob containing the verbose log for the data transfer operation.
*
*/
public Optional verboseLogUri() {
return Optional.ofNullable(this.verboseLogUri);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DriveStatusResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String bitLockerKey;
private @Nullable Double bytesSucceeded;
private @Nullable String copyStatus;
private @Nullable String driveHeaderHash;
private @Nullable String driveId;
private @Nullable String errorLogUri;
private @Nullable String manifestFile;
private @Nullable String manifestHash;
private @Nullable String manifestUri;
private @Nullable Double percentComplete;
private @Nullable String state;
private @Nullable String verboseLogUri;
public Builder() {}
public Builder(DriveStatusResponse defaults) {
Objects.requireNonNull(defaults);
this.bitLockerKey = defaults.bitLockerKey;
this.bytesSucceeded = defaults.bytesSucceeded;
this.copyStatus = defaults.copyStatus;
this.driveHeaderHash = defaults.driveHeaderHash;
this.driveId = defaults.driveId;
this.errorLogUri = defaults.errorLogUri;
this.manifestFile = defaults.manifestFile;
this.manifestHash = defaults.manifestHash;
this.manifestUri = defaults.manifestUri;
this.percentComplete = defaults.percentComplete;
this.state = defaults.state;
this.verboseLogUri = defaults.verboseLogUri;
}
@CustomType.Setter
public Builder bitLockerKey(@Nullable String bitLockerKey) {
this.bitLockerKey = bitLockerKey;
return this;
}
@CustomType.Setter
public Builder bytesSucceeded(@Nullable Double bytesSucceeded) {
this.bytesSucceeded = bytesSucceeded;
return this;
}
@CustomType.Setter
public Builder copyStatus(@Nullable String copyStatus) {
this.copyStatus = copyStatus;
return this;
}
@CustomType.Setter
public Builder driveHeaderHash(@Nullable String driveHeaderHash) {
this.driveHeaderHash = driveHeaderHash;
return this;
}
@CustomType.Setter
public Builder driveId(@Nullable String driveId) {
this.driveId = driveId;
return this;
}
@CustomType.Setter
public Builder errorLogUri(@Nullable String errorLogUri) {
this.errorLogUri = errorLogUri;
return this;
}
@CustomType.Setter
public Builder manifestFile(@Nullable String manifestFile) {
this.manifestFile = manifestFile;
return this;
}
@CustomType.Setter
public Builder manifestHash(@Nullable String manifestHash) {
this.manifestHash = manifestHash;
return this;
}
@CustomType.Setter
public Builder manifestUri(@Nullable String manifestUri) {
this.manifestUri = manifestUri;
return this;
}
@CustomType.Setter
public Builder percentComplete(@Nullable Double percentComplete) {
this.percentComplete = percentComplete;
return this;
}
@CustomType.Setter
public Builder state(@Nullable String state) {
this.state = state;
return this;
}
@CustomType.Setter
public Builder verboseLogUri(@Nullable String verboseLogUri) {
this.verboseLogUri = verboseLogUri;
return this;
}
public DriveStatusResponse build() {
final var _resultValue = new DriveStatusResponse();
_resultValue.bitLockerKey = bitLockerKey;
_resultValue.bytesSucceeded = bytesSucceeded;
_resultValue.copyStatus = copyStatus;
_resultValue.driveHeaderHash = driveHeaderHash;
_resultValue.driveId = driveId;
_resultValue.errorLogUri = errorLogUri;
_resultValue.manifestFile = manifestFile;
_resultValue.manifestHash = manifestHash;
_resultValue.manifestUri = manifestUri;
_resultValue.percentComplete = percentComplete;
_resultValue.state = state;
_resultValue.verboseLogUri = verboseLogUri;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy