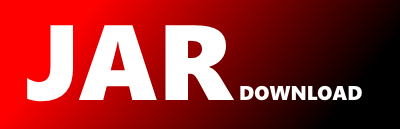
com.pulumi.azurenative.insights.inputs.DataCollectionRuleDestinationsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights.inputs;
import com.pulumi.azurenative.insights.inputs.DestinationsSpecAzureMonitorMetricsArgs;
import com.pulumi.azurenative.insights.inputs.EventHubDestinationArgs;
import com.pulumi.azurenative.insights.inputs.EventHubDirectDestinationArgs;
import com.pulumi.azurenative.insights.inputs.LogAnalyticsDestinationArgs;
import com.pulumi.azurenative.insights.inputs.MonitoringAccountDestinationArgs;
import com.pulumi.azurenative.insights.inputs.StorageBlobDestinationArgs;
import com.pulumi.azurenative.insights.inputs.StorageTableDestinationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The specification of destinations.
*
*/
public final class DataCollectionRuleDestinationsArgs extends com.pulumi.resources.ResourceArgs {
public static final DataCollectionRuleDestinationsArgs Empty = new DataCollectionRuleDestinationsArgs();
/**
* Azure Monitor Metrics destination.
*
*/
@Import(name="azureMonitorMetrics")
private @Nullable Output azureMonitorMetrics;
/**
* @return Azure Monitor Metrics destination.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy