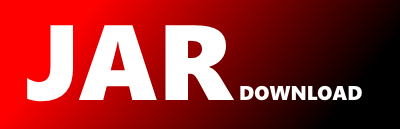
com.pulumi.azurenative.insights.inputs.ThresholdRuleConditionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights.inputs;
import com.pulumi.azurenative.insights.enums.ConditionOperator;
import com.pulumi.azurenative.insights.enums.TimeAggregationOperator;
import com.pulumi.azurenative.insights.inputs.RuleManagementEventDataSourceArgs;
import com.pulumi.azurenative.insights.inputs.RuleMetricDataSourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A rule condition based on a metric crossing a threshold.
*
*/
public final class ThresholdRuleConditionArgs extends com.pulumi.resources.ResourceArgs {
public static final ThresholdRuleConditionArgs Empty = new ThresholdRuleConditionArgs();
/**
* the resource from which the rule collects its data. For this type dataSource will always be of type RuleMetricDataSource.
*
*/
@Import(name="dataSource")
private @Nullable Output> dataSource;
/**
* @return the resource from which the rule collects its data. For this type dataSource will always be of type RuleMetricDataSource.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy