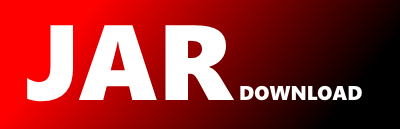
com.pulumi.azurenative.iotoperations.outputs.ClientConfigResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperations.outputs;
import com.pulumi.azurenative.iotoperations.outputs.SubscriberQueueLimitResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ClientConfigResponse {
/**
* @return Upper bound of a client's Keep Alive, in seconds.
*
*/
private @Nullable Integer maxKeepAliveSeconds;
/**
* @return Upper bound of Message Expiry Interval, in seconds.
*
*/
private @Nullable Integer maxMessageExpirySeconds;
/**
* @return Max message size for a packet in Bytes.
*
*/
private @Nullable Integer maxPacketSizeBytes;
/**
* @return Upper bound of Receive Maximum that a client can request in the CONNECT packet.
*
*/
private @Nullable Integer maxReceiveMaximum;
/**
* @return Upper bound of Session Expiry Interval, in seconds.
*
*/
private @Nullable Integer maxSessionExpirySeconds;
/**
* @return The limit on the number of queued messages for a subscriber.
*
*/
private @Nullable SubscriberQueueLimitResponse subscriberQueueLimit;
private ClientConfigResponse() {}
/**
* @return Upper bound of a client's Keep Alive, in seconds.
*
*/
public Optional maxKeepAliveSeconds() {
return Optional.ofNullable(this.maxKeepAliveSeconds);
}
/**
* @return Upper bound of Message Expiry Interval, in seconds.
*
*/
public Optional maxMessageExpirySeconds() {
return Optional.ofNullable(this.maxMessageExpirySeconds);
}
/**
* @return Max message size for a packet in Bytes.
*
*/
public Optional maxPacketSizeBytes() {
return Optional.ofNullable(this.maxPacketSizeBytes);
}
/**
* @return Upper bound of Receive Maximum that a client can request in the CONNECT packet.
*
*/
public Optional maxReceiveMaximum() {
return Optional.ofNullable(this.maxReceiveMaximum);
}
/**
* @return Upper bound of Session Expiry Interval, in seconds.
*
*/
public Optional maxSessionExpirySeconds() {
return Optional.ofNullable(this.maxSessionExpirySeconds);
}
/**
* @return The limit on the number of queued messages for a subscriber.
*
*/
public Optional subscriberQueueLimit() {
return Optional.ofNullable(this.subscriberQueueLimit);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ClientConfigResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer maxKeepAliveSeconds;
private @Nullable Integer maxMessageExpirySeconds;
private @Nullable Integer maxPacketSizeBytes;
private @Nullable Integer maxReceiveMaximum;
private @Nullable Integer maxSessionExpirySeconds;
private @Nullable SubscriberQueueLimitResponse subscriberQueueLimit;
public Builder() {}
public Builder(ClientConfigResponse defaults) {
Objects.requireNonNull(defaults);
this.maxKeepAliveSeconds = defaults.maxKeepAliveSeconds;
this.maxMessageExpirySeconds = defaults.maxMessageExpirySeconds;
this.maxPacketSizeBytes = defaults.maxPacketSizeBytes;
this.maxReceiveMaximum = defaults.maxReceiveMaximum;
this.maxSessionExpirySeconds = defaults.maxSessionExpirySeconds;
this.subscriberQueueLimit = defaults.subscriberQueueLimit;
}
@CustomType.Setter
public Builder maxKeepAliveSeconds(@Nullable Integer maxKeepAliveSeconds) {
this.maxKeepAliveSeconds = maxKeepAliveSeconds;
return this;
}
@CustomType.Setter
public Builder maxMessageExpirySeconds(@Nullable Integer maxMessageExpirySeconds) {
this.maxMessageExpirySeconds = maxMessageExpirySeconds;
return this;
}
@CustomType.Setter
public Builder maxPacketSizeBytes(@Nullable Integer maxPacketSizeBytes) {
this.maxPacketSizeBytes = maxPacketSizeBytes;
return this;
}
@CustomType.Setter
public Builder maxReceiveMaximum(@Nullable Integer maxReceiveMaximum) {
this.maxReceiveMaximum = maxReceiveMaximum;
return this;
}
@CustomType.Setter
public Builder maxSessionExpirySeconds(@Nullable Integer maxSessionExpirySeconds) {
this.maxSessionExpirySeconds = maxSessionExpirySeconds;
return this;
}
@CustomType.Setter
public Builder subscriberQueueLimit(@Nullable SubscriberQueueLimitResponse subscriberQueueLimit) {
this.subscriberQueueLimit = subscriberQueueLimit;
return this;
}
public ClientConfigResponse build() {
final var _resultValue = new ClientConfigResponse();
_resultValue.maxKeepAliveSeconds = maxKeepAliveSeconds;
_resultValue.maxMessageExpirySeconds = maxMessageExpirySeconds;
_resultValue.maxPacketSizeBytes = maxPacketSizeBytes;
_resultValue.maxReceiveMaximum = maxReceiveMaximum;
_resultValue.maxSessionExpirySeconds = maxSessionExpirySeconds;
_resultValue.subscriberQueueLimit = subscriberQueueLimit;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy