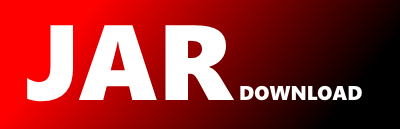
com.pulumi.azurenative.kubernetesconfiguration.Extension Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kubernetesconfiguration;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.kubernetesconfiguration.ExtensionArgs;
import com.pulumi.azurenative.kubernetesconfiguration.outputs.ErrorDetailResponse;
import com.pulumi.azurenative.kubernetesconfiguration.outputs.ExtensionResponseAksAssignedIdentity;
import com.pulumi.azurenative.kubernetesconfiguration.outputs.ExtensionStatusResponse;
import com.pulumi.azurenative.kubernetesconfiguration.outputs.IdentityResponse;
import com.pulumi.azurenative.kubernetesconfiguration.outputs.PlanResponse;
import com.pulumi.azurenative.kubernetesconfiguration.outputs.ScopeResponse;
import com.pulumi.azurenative.kubernetesconfiguration.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The Extension object.
* Azure REST API version: 2023-05-01. Prior API version in Azure Native 1.x: 2020-07-01-preview.
*
* Other available API versions: 2020-07-01-preview, 2022-04-02-preview, 2022-07-01.
*
* ## Example Usage
* ### Create Extension
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.kubernetesconfiguration.Extension;
* import com.pulumi.azurenative.kubernetesconfiguration.ExtensionArgs;
* import com.pulumi.azurenative.kubernetesconfiguration.inputs.ScopeArgs;
* import com.pulumi.azurenative.kubernetesconfiguration.inputs.ScopeClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var extension = new Extension("extension", ExtensionArgs.builder()
* .autoUpgradeMinorVersion(true)
* .clusterName("clusterName1")
* .clusterResourceName("connectedClusters")
* .clusterRp("Microsoft.Kubernetes")
* .configurationProtectedSettings(Map.of("omsagent.secret.key", "secretKeyValue01"))
* .configurationSettings(Map.ofEntries(
* Map.entry("omsagent.env.clusterName", "clusterName1"),
* Map.entry("omsagent.secret.wsid", "fakeTokenPlaceholder")
* ))
* .extensionName("ClusterMonitor")
* .extensionType("azuremonitor-containers")
* .releaseTrain("Preview")
* .resourceGroupName("rg1")
* .scope(ScopeArgs.builder()
* .cluster(ScopeClusterArgs.builder()
* .releaseNamespace("kube-system")
* .build())
* .build())
* .build());
*
* }
* }
*
* }
*
* ### Create Extension with Plan
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.kubernetesconfiguration.Extension;
* import com.pulumi.azurenative.kubernetesconfiguration.ExtensionArgs;
* import com.pulumi.azurenative.kubernetesconfiguration.inputs.PlanArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var extension = new Extension("extension", ExtensionArgs.builder()
* .autoUpgradeMinorVersion(true)
* .clusterName("clusterName1")
* .clusterResourceName("connectedClusters")
* .clusterRp("Microsoft.Kubernetes")
* .extensionName("azureVote")
* .extensionType("azure-vote")
* .plan(PlanArgs.builder()
* .name("azure-vote-standard")
* .product("azure-vote-standard-offer-id")
* .publisher("Microsoft")
* .build())
* .releaseTrain("Preview")
* .resourceGroupName("rg1")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:kubernetesconfiguration:Extension azureVote /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{clusterRp}/{clusterResourceName}/{clusterName}/providers/Microsoft.KubernetesConfiguration/extensions/{extensionName}
* ```
*
*/
@ResourceType(type="azure-native:kubernetesconfiguration:Extension")
public class Extension extends com.pulumi.resources.CustomResource {
/**
* Identity of the Extension resource in an AKS cluster
*
*/
@Export(name="aksAssignedIdentity", refs={ExtensionResponseAksAssignedIdentity.class}, tree="[0]")
private Output* @Nullable */ ExtensionResponseAksAssignedIdentity> aksAssignedIdentity;
/**
* @return Identity of the Extension resource in an AKS cluster
*
*/
public Output> aksAssignedIdentity() {
return Codegen.optional(this.aksAssignedIdentity);
}
/**
* Flag to note if this extension participates in auto upgrade of minor version, or not.
*
*/
@Export(name="autoUpgradeMinorVersion", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> autoUpgradeMinorVersion;
/**
* @return Flag to note if this extension participates in auto upgrade of minor version, or not.
*
*/
public Output> autoUpgradeMinorVersion() {
return Codegen.optional(this.autoUpgradeMinorVersion);
}
/**
* Configuration settings that are sensitive, as name-value pairs for configuring this extension.
*
*/
@Export(name="configurationProtectedSettings", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> configurationProtectedSettings;
/**
* @return Configuration settings that are sensitive, as name-value pairs for configuring this extension.
*
*/
public Output>> configurationProtectedSettings() {
return Codegen.optional(this.configurationProtectedSettings);
}
/**
* Configuration settings, as name-value pairs for configuring this extension.
*
*/
@Export(name="configurationSettings", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> configurationSettings;
/**
* @return Configuration settings, as name-value pairs for configuring this extension.
*
*/
public Output>> configurationSettings() {
return Codegen.optional(this.configurationSettings);
}
/**
* Currently installed version of the extension.
*
*/
@Export(name="currentVersion", refs={String.class}, tree="[0]")
private Output currentVersion;
/**
* @return Currently installed version of the extension.
*
*/
public Output currentVersion() {
return this.currentVersion;
}
/**
* Custom Location settings properties.
*
*/
@Export(name="customLocationSettings", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy