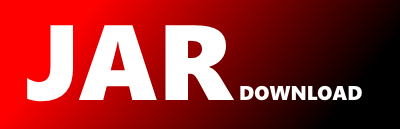
com.pulumi.azurenative.labservices.LabPlanArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.labservices;
import com.pulumi.azurenative.labservices.inputs.AutoShutdownProfileArgs;
import com.pulumi.azurenative.labservices.inputs.ConnectionProfileArgs;
import com.pulumi.azurenative.labservices.inputs.IdentityArgs;
import com.pulumi.azurenative.labservices.inputs.LabPlanNetworkProfileArgs;
import com.pulumi.azurenative.labservices.inputs.SupportInfoArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LabPlanArgs extends com.pulumi.resources.ResourceArgs {
public static final LabPlanArgs Empty = new LabPlanArgs();
/**
* The allowed regions for the lab creator to use when creating labs using this lab plan.
*
*/
@Import(name="allowedRegions")
private @Nullable Output> allowedRegions;
/**
* @return The allowed regions for the lab creator to use when creating labs using this lab plan.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy