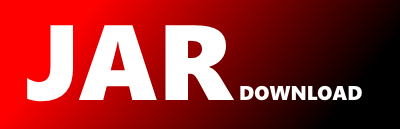
com.pulumi.azurenative.machinelearningservices.outputs.DataFactoryResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices.outputs;
import com.pulumi.azurenative.machinelearningservices.outputs.ErrorResponseResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DataFactoryResponse {
/**
* @return Location for the underlying compute
*
*/
private @Nullable String computeLocation;
/**
* @return The type of compute
* Expected value is 'DataFactory'.
*
*/
private String computeType;
/**
* @return The time at which the compute was created.
*
*/
private String createdOn;
/**
* @return The description of the Machine Learning compute.
*
*/
private @Nullable String description;
/**
* @return Opt-out of local authentication and ensure customers can use only MSI and AAD exclusively for authentication.
*
*/
private @Nullable Boolean disableLocalAuth;
/**
* @return Indicating whether the compute was provisioned by user and brought from outside if true, or machine learning service provisioned it if false.
*
*/
private Boolean isAttachedCompute;
/**
* @return The time at which the compute was last modified.
*
*/
private String modifiedOn;
/**
* @return Errors during provisioning
*
*/
private List provisioningErrors;
/**
* @return The provision state of the cluster. Valid values are Unknown, Updating, Provisioning, Succeeded, and Failed.
*
*/
private String provisioningState;
/**
* @return ARM resource id of the underlying compute
*
*/
private @Nullable String resourceId;
private DataFactoryResponse() {}
/**
* @return Location for the underlying compute
*
*/
public Optional computeLocation() {
return Optional.ofNullable(this.computeLocation);
}
/**
* @return The type of compute
* Expected value is 'DataFactory'.
*
*/
public String computeType() {
return this.computeType;
}
/**
* @return The time at which the compute was created.
*
*/
public String createdOn() {
return this.createdOn;
}
/**
* @return The description of the Machine Learning compute.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Opt-out of local authentication and ensure customers can use only MSI and AAD exclusively for authentication.
*
*/
public Optional disableLocalAuth() {
return Optional.ofNullable(this.disableLocalAuth);
}
/**
* @return Indicating whether the compute was provisioned by user and brought from outside if true, or machine learning service provisioned it if false.
*
*/
public Boolean isAttachedCompute() {
return this.isAttachedCompute;
}
/**
* @return The time at which the compute was last modified.
*
*/
public String modifiedOn() {
return this.modifiedOn;
}
/**
* @return Errors during provisioning
*
*/
public List provisioningErrors() {
return this.provisioningErrors;
}
/**
* @return The provision state of the cluster. Valid values are Unknown, Updating, Provisioning, Succeeded, and Failed.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return ARM resource id of the underlying compute
*
*/
public Optional resourceId() {
return Optional.ofNullable(this.resourceId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DataFactoryResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String computeLocation;
private String computeType;
private String createdOn;
private @Nullable String description;
private @Nullable Boolean disableLocalAuth;
private Boolean isAttachedCompute;
private String modifiedOn;
private List provisioningErrors;
private String provisioningState;
private @Nullable String resourceId;
public Builder() {}
public Builder(DataFactoryResponse defaults) {
Objects.requireNonNull(defaults);
this.computeLocation = defaults.computeLocation;
this.computeType = defaults.computeType;
this.createdOn = defaults.createdOn;
this.description = defaults.description;
this.disableLocalAuth = defaults.disableLocalAuth;
this.isAttachedCompute = defaults.isAttachedCompute;
this.modifiedOn = defaults.modifiedOn;
this.provisioningErrors = defaults.provisioningErrors;
this.provisioningState = defaults.provisioningState;
this.resourceId = defaults.resourceId;
}
@CustomType.Setter
public Builder computeLocation(@Nullable String computeLocation) {
this.computeLocation = computeLocation;
return this;
}
@CustomType.Setter
public Builder computeType(String computeType) {
if (computeType == null) {
throw new MissingRequiredPropertyException("DataFactoryResponse", "computeType");
}
this.computeType = computeType;
return this;
}
@CustomType.Setter
public Builder createdOn(String createdOn) {
if (createdOn == null) {
throw new MissingRequiredPropertyException("DataFactoryResponse", "createdOn");
}
this.createdOn = createdOn;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder disableLocalAuth(@Nullable Boolean disableLocalAuth) {
this.disableLocalAuth = disableLocalAuth;
return this;
}
@CustomType.Setter
public Builder isAttachedCompute(Boolean isAttachedCompute) {
if (isAttachedCompute == null) {
throw new MissingRequiredPropertyException("DataFactoryResponse", "isAttachedCompute");
}
this.isAttachedCompute = isAttachedCompute;
return this;
}
@CustomType.Setter
public Builder modifiedOn(String modifiedOn) {
if (modifiedOn == null) {
throw new MissingRequiredPropertyException("DataFactoryResponse", "modifiedOn");
}
this.modifiedOn = modifiedOn;
return this;
}
@CustomType.Setter
public Builder provisioningErrors(List provisioningErrors) {
if (provisioningErrors == null) {
throw new MissingRequiredPropertyException("DataFactoryResponse", "provisioningErrors");
}
this.provisioningErrors = provisioningErrors;
return this;
}
public Builder provisioningErrors(ErrorResponseResponse... provisioningErrors) {
return provisioningErrors(List.of(provisioningErrors));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("DataFactoryResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder resourceId(@Nullable String resourceId) {
this.resourceId = resourceId;
return this;
}
public DataFactoryResponse build() {
final var _resultValue = new DataFactoryResponse();
_resultValue.computeLocation = computeLocation;
_resultValue.computeType = computeType;
_resultValue.createdOn = createdOn;
_resultValue.description = description;
_resultValue.disableLocalAuth = disableLocalAuth;
_resultValue.isAttachedCompute = isAttachedCompute;
_resultValue.modifiedOn = modifiedOn;
_resultValue.provisioningErrors = provisioningErrors;
_resultValue.provisioningState = provisioningState;
_resultValue.resourceId = resourceId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy