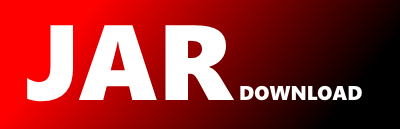
com.pulumi.azurenative.managedservices.outputs.RegistrationAssignmentPropertiesResponseProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.managedservices.outputs;
import com.pulumi.azurenative.managedservices.outputs.AuthorizationResponse;
import com.pulumi.azurenative.managedservices.outputs.EligibleAuthorizationResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class RegistrationAssignmentPropertiesResponseProperties {
/**
* @return The collection of authorization objects describing the access Azure Active Directory principals in the managedBy tenant will receive on the delegated resource in the managed tenant.
*
*/
private @Nullable List authorizations;
/**
* @return The description of the registration definition.
*
*/
private @Nullable String description;
/**
* @return The collection of eligible authorization objects describing the just-in-time access Azure Active Directory principals in the managedBy tenant will receive on the delegated resource in the managed tenant.
*
*/
private @Nullable List eligibleAuthorizations;
/**
* @return The identifier of the managedBy tenant.
*
*/
private @Nullable String managedByTenantId;
/**
* @return The name of the managedBy tenant.
*
*/
private @Nullable String managedByTenantName;
/**
* @return The identifier of the managed tenant.
*
*/
private @Nullable String manageeTenantId;
/**
* @return The name of the managed tenant.
*
*/
private @Nullable String manageeTenantName;
/**
* @return The current provisioning state of the registration definition.
*
*/
private @Nullable String provisioningState;
/**
* @return The name of the registration definition.
*
*/
private @Nullable String registrationDefinitionName;
private RegistrationAssignmentPropertiesResponseProperties() {}
/**
* @return The collection of authorization objects describing the access Azure Active Directory principals in the managedBy tenant will receive on the delegated resource in the managed tenant.
*
*/
public List authorizations() {
return this.authorizations == null ? List.of() : this.authorizations;
}
/**
* @return The description of the registration definition.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return The collection of eligible authorization objects describing the just-in-time access Azure Active Directory principals in the managedBy tenant will receive on the delegated resource in the managed tenant.
*
*/
public List eligibleAuthorizations() {
return this.eligibleAuthorizations == null ? List.of() : this.eligibleAuthorizations;
}
/**
* @return The identifier of the managedBy tenant.
*
*/
public Optional managedByTenantId() {
return Optional.ofNullable(this.managedByTenantId);
}
/**
* @return The name of the managedBy tenant.
*
*/
public Optional managedByTenantName() {
return Optional.ofNullable(this.managedByTenantName);
}
/**
* @return The identifier of the managed tenant.
*
*/
public Optional manageeTenantId() {
return Optional.ofNullable(this.manageeTenantId);
}
/**
* @return The name of the managed tenant.
*
*/
public Optional manageeTenantName() {
return Optional.ofNullable(this.manageeTenantName);
}
/**
* @return The current provisioning state of the registration definition.
*
*/
public Optional provisioningState() {
return Optional.ofNullable(this.provisioningState);
}
/**
* @return The name of the registration definition.
*
*/
public Optional registrationDefinitionName() {
return Optional.ofNullable(this.registrationDefinitionName);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RegistrationAssignmentPropertiesResponseProperties defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List authorizations;
private @Nullable String description;
private @Nullable List eligibleAuthorizations;
private @Nullable String managedByTenantId;
private @Nullable String managedByTenantName;
private @Nullable String manageeTenantId;
private @Nullable String manageeTenantName;
private @Nullable String provisioningState;
private @Nullable String registrationDefinitionName;
public Builder() {}
public Builder(RegistrationAssignmentPropertiesResponseProperties defaults) {
Objects.requireNonNull(defaults);
this.authorizations = defaults.authorizations;
this.description = defaults.description;
this.eligibleAuthorizations = defaults.eligibleAuthorizations;
this.managedByTenantId = defaults.managedByTenantId;
this.managedByTenantName = defaults.managedByTenantName;
this.manageeTenantId = defaults.manageeTenantId;
this.manageeTenantName = defaults.manageeTenantName;
this.provisioningState = defaults.provisioningState;
this.registrationDefinitionName = defaults.registrationDefinitionName;
}
@CustomType.Setter
public Builder authorizations(@Nullable List authorizations) {
this.authorizations = authorizations;
return this;
}
public Builder authorizations(AuthorizationResponse... authorizations) {
return authorizations(List.of(authorizations));
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder eligibleAuthorizations(@Nullable List eligibleAuthorizations) {
this.eligibleAuthorizations = eligibleAuthorizations;
return this;
}
public Builder eligibleAuthorizations(EligibleAuthorizationResponse... eligibleAuthorizations) {
return eligibleAuthorizations(List.of(eligibleAuthorizations));
}
@CustomType.Setter
public Builder managedByTenantId(@Nullable String managedByTenantId) {
this.managedByTenantId = managedByTenantId;
return this;
}
@CustomType.Setter
public Builder managedByTenantName(@Nullable String managedByTenantName) {
this.managedByTenantName = managedByTenantName;
return this;
}
@CustomType.Setter
public Builder manageeTenantId(@Nullable String manageeTenantId) {
this.manageeTenantId = manageeTenantId;
return this;
}
@CustomType.Setter
public Builder manageeTenantName(@Nullable String manageeTenantName) {
this.manageeTenantName = manageeTenantName;
return this;
}
@CustomType.Setter
public Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder registrationDefinitionName(@Nullable String registrationDefinitionName) {
this.registrationDefinitionName = registrationDefinitionName;
return this;
}
public RegistrationAssignmentPropertiesResponseProperties build() {
final var _resultValue = new RegistrationAssignmentPropertiesResponseProperties();
_resultValue.authorizations = authorizations;
_resultValue.description = description;
_resultValue.eligibleAuthorizations = eligibleAuthorizations;
_resultValue.managedByTenantId = managedByTenantId;
_resultValue.managedByTenantName = managedByTenantName;
_resultValue.manageeTenantId = manageeTenantId;
_resultValue.manageeTenantName = manageeTenantName;
_resultValue.provisioningState = provisioningState;
_resultValue.registrationDefinitionName = registrationDefinitionName;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy