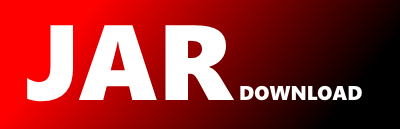
com.pulumi.azurenative.media.inputs.H265VideoArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.inputs;
import com.pulumi.azurenative.media.enums.H265Complexity;
import com.pulumi.azurenative.media.enums.StretchMode;
import com.pulumi.azurenative.media.enums.VideoSyncMode;
import com.pulumi.azurenative.media.inputs.H265LayerArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes all the properties for encoding a video with the H.265 codec.
*
*/
public final class H265VideoArgs extends com.pulumi.resources.ResourceArgs {
public static final H265VideoArgs Empty = new H265VideoArgs();
/**
* Tells the encoder how to choose its encoding settings. Quality will provide for a higher compression ratio but at a higher cost and longer compute time. Speed will produce a relatively larger file but is faster and more economical. The default value is Balanced.
*
*/
@Import(name="complexity")
private @Nullable Output> complexity;
/**
* @return Tells the encoder how to choose its encoding settings. Quality will provide for a higher compression ratio but at a higher cost and longer compute time. Speed will produce a relatively larger file but is faster and more economical. The default value is Balanced.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy