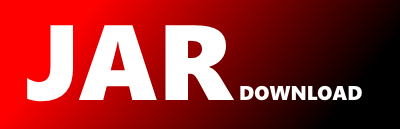
com.pulumi.azurenative.media.inputs.LiveEventPreviewArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.inputs;
import com.pulumi.azurenative.media.inputs.LiveEventEndpointArgs;
import com.pulumi.azurenative.media.inputs.LiveEventPreviewAccessControlArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Live event preview settings.
*
*/
public final class LiveEventPreviewArgs extends com.pulumi.resources.ResourceArgs {
public static final LiveEventPreviewArgs Empty = new LiveEventPreviewArgs();
/**
* The access control for live event preview.
*
*/
@Import(name="accessControl")
private @Nullable Output accessControl;
/**
* @return The access control for live event preview.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy