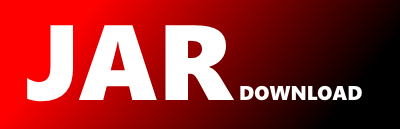
com.pulumi.azurenative.media.inputs.PresetConfigurationsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.inputs;
import com.pulumi.azurenative.media.enums.Complexity;
import com.pulumi.azurenative.media.enums.InterleaveOutput;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* An object of optional configuration settings for encoder.
*
*/
public final class PresetConfigurationsArgs extends com.pulumi.resources.ResourceArgs {
public static final PresetConfigurationsArgs Empty = new PresetConfigurationsArgs();
/**
* Allows you to configure the encoder settings to control the balance between speed and quality. Example: set Complexity as Speed for faster encoding but less compression efficiency.
*
*/
@Import(name="complexity")
private @Nullable Output> complexity;
/**
* @return Allows you to configure the encoder settings to control the balance between speed and quality. Example: set Complexity as Speed for faster encoding but less compression efficiency.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy