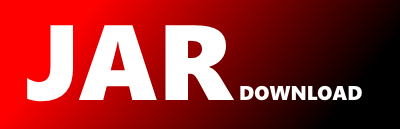
com.pulumi.azurenative.media.outputs.GetStreamingLocatorResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.azurenative.media.outputs.StreamingLocatorContentKeyResponse;
import com.pulumi.azurenative.media.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetStreamingLocatorResult {
/**
* @return Alternative Media ID of this Streaming Locator
*
*/
private @Nullable String alternativeMediaId;
/**
* @return Asset Name
*
*/
private String assetName;
/**
* @return The ContentKeys used by this Streaming Locator.
*
*/
private @Nullable List contentKeys;
/**
* @return The creation time of the Streaming Locator.
*
*/
private String created;
/**
* @return Name of the default ContentKeyPolicy used by this Streaming Locator.
*
*/
private @Nullable String defaultContentKeyPolicyName;
/**
* @return The end time of the Streaming Locator.
*
*/
private @Nullable String endTime;
/**
* @return A list of asset or account filters which apply to this streaming locator
*
*/
private @Nullable List filters;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The start time of the Streaming Locator.
*
*/
private @Nullable String startTime;
/**
* @return The StreamingLocatorId of the Streaming Locator.
*
*/
private @Nullable String streamingLocatorId;
/**
* @return Name of the Streaming Policy used by this Streaming Locator. Either specify the name of Streaming Policy you created or use one of the predefined Streaming Policies. The predefined Streaming Policies available are: 'Predefined_DownloadOnly', 'Predefined_ClearStreamingOnly', 'Predefined_DownloadAndClearStreaming', 'Predefined_ClearKey', 'Predefined_MultiDrmCencStreaming' and 'Predefined_MultiDrmStreaming'
*
*/
private String streamingPolicyName;
/**
* @return The system metadata relating to this resource.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetStreamingLocatorResult() {}
/**
* @return Alternative Media ID of this Streaming Locator
*
*/
public Optional alternativeMediaId() {
return Optional.ofNullable(this.alternativeMediaId);
}
/**
* @return Asset Name
*
*/
public String assetName() {
return this.assetName;
}
/**
* @return The ContentKeys used by this Streaming Locator.
*
*/
public List contentKeys() {
return this.contentKeys == null ? List.of() : this.contentKeys;
}
/**
* @return The creation time of the Streaming Locator.
*
*/
public String created() {
return this.created;
}
/**
* @return Name of the default ContentKeyPolicy used by this Streaming Locator.
*
*/
public Optional defaultContentKeyPolicyName() {
return Optional.ofNullable(this.defaultContentKeyPolicyName);
}
/**
* @return The end time of the Streaming Locator.
*
*/
public Optional endTime() {
return Optional.ofNullable(this.endTime);
}
/**
* @return A list of asset or account filters which apply to this streaming locator
*
*/
public List filters() {
return this.filters == null ? List.of() : this.filters;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The start time of the Streaming Locator.
*
*/
public Optional startTime() {
return Optional.ofNullable(this.startTime);
}
/**
* @return The StreamingLocatorId of the Streaming Locator.
*
*/
public Optional streamingLocatorId() {
return Optional.ofNullable(this.streamingLocatorId);
}
/**
* @return Name of the Streaming Policy used by this Streaming Locator. Either specify the name of Streaming Policy you created or use one of the predefined Streaming Policies. The predefined Streaming Policies available are: 'Predefined_DownloadOnly', 'Predefined_ClearStreamingOnly', 'Predefined_DownloadAndClearStreaming', 'Predefined_ClearKey', 'Predefined_MultiDrmCencStreaming' and 'Predefined_MultiDrmStreaming'
*
*/
public String streamingPolicyName() {
return this.streamingPolicyName;
}
/**
* @return The system metadata relating to this resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetStreamingLocatorResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String alternativeMediaId;
private String assetName;
private @Nullable List contentKeys;
private String created;
private @Nullable String defaultContentKeyPolicyName;
private @Nullable String endTime;
private @Nullable List filters;
private String id;
private String name;
private @Nullable String startTime;
private @Nullable String streamingLocatorId;
private String streamingPolicyName;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetStreamingLocatorResult defaults) {
Objects.requireNonNull(defaults);
this.alternativeMediaId = defaults.alternativeMediaId;
this.assetName = defaults.assetName;
this.contentKeys = defaults.contentKeys;
this.created = defaults.created;
this.defaultContentKeyPolicyName = defaults.defaultContentKeyPolicyName;
this.endTime = defaults.endTime;
this.filters = defaults.filters;
this.id = defaults.id;
this.name = defaults.name;
this.startTime = defaults.startTime;
this.streamingLocatorId = defaults.streamingLocatorId;
this.streamingPolicyName = defaults.streamingPolicyName;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder alternativeMediaId(@Nullable String alternativeMediaId) {
this.alternativeMediaId = alternativeMediaId;
return this;
}
@CustomType.Setter
public Builder assetName(String assetName) {
if (assetName == null) {
throw new MissingRequiredPropertyException("GetStreamingLocatorResult", "assetName");
}
this.assetName = assetName;
return this;
}
@CustomType.Setter
public Builder contentKeys(@Nullable List contentKeys) {
this.contentKeys = contentKeys;
return this;
}
public Builder contentKeys(StreamingLocatorContentKeyResponse... contentKeys) {
return contentKeys(List.of(contentKeys));
}
@CustomType.Setter
public Builder created(String created) {
if (created == null) {
throw new MissingRequiredPropertyException("GetStreamingLocatorResult", "created");
}
this.created = created;
return this;
}
@CustomType.Setter
public Builder defaultContentKeyPolicyName(@Nullable String defaultContentKeyPolicyName) {
this.defaultContentKeyPolicyName = defaultContentKeyPolicyName;
return this;
}
@CustomType.Setter
public Builder endTime(@Nullable String endTime) {
this.endTime = endTime;
return this;
}
@CustomType.Setter
public Builder filters(@Nullable List filters) {
this.filters = filters;
return this;
}
public Builder filters(String... filters) {
return filters(List.of(filters));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetStreamingLocatorResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetStreamingLocatorResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder startTime(@Nullable String startTime) {
this.startTime = startTime;
return this;
}
@CustomType.Setter
public Builder streamingLocatorId(@Nullable String streamingLocatorId) {
this.streamingLocatorId = streamingLocatorId;
return this;
}
@CustomType.Setter
public Builder streamingPolicyName(String streamingPolicyName) {
if (streamingPolicyName == null) {
throw new MissingRequiredPropertyException("GetStreamingLocatorResult", "streamingPolicyName");
}
this.streamingPolicyName = streamingPolicyName;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetStreamingLocatorResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetStreamingLocatorResult", "type");
}
this.type = type;
return this;
}
public GetStreamingLocatorResult build() {
final var _resultValue = new GetStreamingLocatorResult();
_resultValue.alternativeMediaId = alternativeMediaId;
_resultValue.assetName = assetName;
_resultValue.contentKeys = contentKeys;
_resultValue.created = created;
_resultValue.defaultContentKeyPolicyName = defaultContentKeyPolicyName;
_resultValue.endTime = endTime;
_resultValue.filters = filters;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.startTime = startTime;
_resultValue.streamingLocatorId = streamingLocatorId;
_resultValue.streamingPolicyName = streamingPolicyName;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy