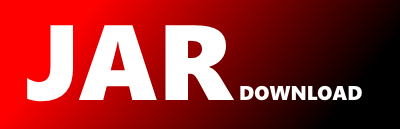
com.pulumi.azurenative.mobilepacketcore.NetworkFunctionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.mobilepacketcore;
import com.pulumi.azurenative.mobilepacketcore.enums.NetworkFunctionAdministrativeState;
import com.pulumi.azurenative.mobilepacketcore.enums.NetworkFunctionType;
import com.pulumi.azurenative.mobilepacketcore.enums.SkuDefinitions;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class NetworkFunctionArgs extends com.pulumi.resources.ResourceArgs {
public static final NetworkFunctionArgs Empty = new NetworkFunctionArgs();
/**
* Capacity of the network function in units of 10000. This represents the session count or the Simultaneously Attached Users (SAU) count as applicable
*
*/
@Import(name="capacity")
private @Nullable Output capacity;
/**
* @return Capacity of the network function in units of 10000. This represents the session count or the Simultaneously Attached Users (SAU) count as applicable
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy