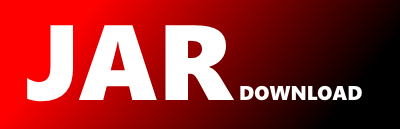
com.pulumi.azurenative.netapp.outputs.GetVolumeResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.netapp.outputs;
import com.pulumi.azurenative.netapp.outputs.MountTargetPropertiesResponse;
import com.pulumi.azurenative.netapp.outputs.PlacementKeyValuePairsResponse;
import com.pulumi.azurenative.netapp.outputs.SystemDataResponse;
import com.pulumi.azurenative.netapp.outputs.VolumePropertiesResponseDataProtection;
import com.pulumi.azurenative.netapp.outputs.VolumePropertiesResponseExportPolicy;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetVolumeResult {
/**
* @return Actual throughput in MiB/s for auto qosType volumes calculated based on size and serviceLevel
*
*/
private Double actualThroughputMibps;
/**
* @return Specifies whether the volume is enabled for Azure VMware Solution (AVS) datastore purpose
*
*/
private @Nullable String avsDataStore;
/**
* @return UUID v4 or resource identifier used to identify the Backup.
*
*/
private @Nullable String backupId;
/**
* @return Unique Baremetal Tenant Identifier.
*
*/
private String baremetalTenantId;
/**
* @return Pool Resource Id used in case of creating a volume through volume group
*
*/
private @Nullable String capacityPoolResourceId;
/**
* @return When a volume is being restored from another volume's snapshot, will show the percentage completion of this cloning process. When this value is empty/null there is no cloning process currently happening on this volume. This value will update every 5 minutes during cloning.
*
*/
private Integer cloneProgress;
/**
* @return Specifies whether Cool Access(tiering) is enabled for the volume.
*
*/
private @Nullable Boolean coolAccess;
/**
* @return Specifies the number of days after which data that is not accessed by clients will be tiered.
*
*/
private @Nullable Integer coolnessPeriod;
/**
* @return A unique file path for the volume. Used when creating mount targets
*
*/
private String creationToken;
/**
* @return DataProtection type volumes include an object containing details of the replication
*
*/
private @Nullable VolumePropertiesResponseDataProtection dataProtection;
/**
* @return Data store resource unique identifier
*
*/
private List dataStoreResourceId;
/**
* @return Default group quota for volume in KiBs. If isDefaultQuotaEnabled is set, the minimum value of 4 KiBs applies.
*
*/
private @Nullable Double defaultGroupQuotaInKiBs;
/**
* @return Default user quota for volume in KiBs. If isDefaultQuotaEnabled is set, the minimum value of 4 KiBs applies .
*
*/
private @Nullable Double defaultUserQuotaInKiBs;
/**
* @return If enabled (true) the snapshot the volume was created from will be automatically deleted after the volume create operation has finished. Defaults to false
*
*/
private @Nullable Boolean deleteBaseSnapshot;
/**
* @return Flag indicating whether subvolume operations are enabled on the volume
*
*/
private @Nullable String enableSubvolumes;
/**
* @return Specifies if the volume is encrypted or not. Only available on volumes created or updated after 2022-01-01.
*
*/
private Boolean encrypted;
/**
* @return Source of key used to encrypt data in volume. Applicable if NetApp account has encryption.keySource = 'Microsoft.KeyVault'. Possible values (case-insensitive) are: 'Microsoft.NetApp, Microsoft.KeyVault'
*
*/
private @Nullable String encryptionKeySource;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Set of export policy rules
*
*/
private @Nullable VolumePropertiesResponseExportPolicy exportPolicy;
/**
* @return Flag indicating whether file access logs are enabled for the volume, based on active diagnostic settings present on the volume.
*
*/
private String fileAccessLogs;
/**
* @return Unique FileSystem Identifier.
*
*/
private String fileSystemId;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Specifies if default quota is enabled for the volume.
*
*/
private @Nullable Boolean isDefaultQuotaEnabled;
/**
* @return Specifies whether volume is a Large Volume or Regular Volume.
*
*/
private @Nullable Boolean isLargeVolume;
/**
* @return Restoring
*
*/
private @Nullable Boolean isRestoring;
/**
* @return Describe if a volume is KerberosEnabled. To be use with swagger version 2020-05-01 or later
*
*/
private @Nullable Boolean kerberosEnabled;
/**
* @return The resource ID of private endpoint for KeyVault. It must reside in the same VNET as the volume. Only applicable if encryptionKeySource = 'Microsoft.KeyVault'.
*
*/
private @Nullable String keyVaultPrivateEndpointResourceId;
/**
* @return Specifies whether LDAP is enabled or not for a given NFS volume.
*
*/
private @Nullable Boolean ldapEnabled;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return Maximum number of files allowed. Needs a service request in order to be changed. Only allowed to be changed if volume quota is more than 4TiB.
*
*/
private Double maximumNumberOfFiles;
/**
* @return List of mount targets
*
*/
private List mountTargets;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Basic network, or Standard features available to the volume.
*
*/
private @Nullable String networkFeatures;
/**
* @return Network Sibling Set ID for the the group of volumes sharing networking resources.
*
*/
private String networkSiblingSetId;
/**
* @return Id of the snapshot or backup that the volume is restored from.
*
*/
private String originatingResourceId;
/**
* @return Application specific placement rules for the particular volume
*
*/
private @Nullable List placementRules;
/**
* @return Set of protocol types, default NFSv3, CIFS for SMB protocol
*
*/
private @Nullable List protocolTypes;
/**
* @return The availability zone where the volume is provisioned. This refers to the logical availability zone where the volume resides.
*
*/
private String provisionedAvailabilityZone;
/**
* @return Azure lifecycle management
*
*/
private String provisioningState;
/**
* @return Proximity placement group associated with the volume
*
*/
private @Nullable String proximityPlacementGroup;
/**
* @return The security style of volume, default unix, defaults to ntfs for dual protocol or CIFS protocol
*
*/
private @Nullable String securityStyle;
/**
* @return The service level of the file system
*
*/
private @Nullable String serviceLevel;
/**
* @return Enables access based enumeration share property for SMB Shares. Only applicable for SMB/DualProtocol volume
*
*/
private @Nullable String smbAccessBasedEnumeration;
/**
* @return Enables continuously available share property for smb volume. Only applicable for SMB volume
*
*/
private @Nullable Boolean smbContinuouslyAvailable;
/**
* @return Enables encryption for in-flight smb3 data. Only applicable for SMB/DualProtocol volume. To be used with swagger version 2020-08-01 or later
*
*/
private @Nullable Boolean smbEncryption;
/**
* @return Enables non browsable property for SMB Shares. Only applicable for SMB/DualProtocol volume
*
*/
private @Nullable String smbNonBrowsable;
/**
* @return If enabled (true) the volume will contain a read-only snapshot directory which provides access to each of the volume's snapshots (defaults to true).
*
*/
private @Nullable Boolean snapshotDirectoryVisible;
/**
* @return UUID v4 or resource identifier used to identify the Snapshot.
*
*/
private @Nullable String snapshotId;
/**
* @return Provides storage to network proximity information for the volume.
*
*/
private String storageToNetworkProximity;
/**
* @return The Azure Resource URI for a delegated subnet. Must have the delegation Microsoft.NetApp/volumes
*
*/
private String subnetId;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return T2 network information
*
*/
private String t2Network;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
private @Nullable Double throughputMibps;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return UNIX permissions for NFS volume accepted in octal 4 digit format. First digit selects the set user ID(4), set group ID (2) and sticky (1) attributes. Second digit selects permission for the owner of the file: read (4), write (2) and execute (1). Third selects permissions for other users in the same group. the fourth for other users not in the group. 0755 - gives read/write/execute permissions to owner and read/execute to group and other users.
*
*/
private @Nullable String unixPermissions;
/**
* @return Maximum storage quota allowed for a file system in bytes. This is a soft quota used for alerting only. Minimum size is 100 GiB. Upper limit is 100TiB, 500Tib for LargeVolume. Specified in bytes.
*
*/
private Double usageThreshold;
/**
* @return Volume Group Name
*
*/
private String volumeGroupName;
/**
* @return Volume spec name is the application specific designation or identifier for the particular volume in a volume group for e.g. data, log
*
*/
private @Nullable String volumeSpecName;
/**
* @return What type of volume is this. For destination volumes in Cross Region Replication, set type to DataProtection
*
*/
private @Nullable String volumeType;
/**
* @return Availability Zone
*
*/
private @Nullable List zones;
private GetVolumeResult() {}
/**
* @return Actual throughput in MiB/s for auto qosType volumes calculated based on size and serviceLevel
*
*/
public Double actualThroughputMibps() {
return this.actualThroughputMibps;
}
/**
* @return Specifies whether the volume is enabled for Azure VMware Solution (AVS) datastore purpose
*
*/
public Optional avsDataStore() {
return Optional.ofNullable(this.avsDataStore);
}
/**
* @return UUID v4 or resource identifier used to identify the Backup.
*
*/
public Optional backupId() {
return Optional.ofNullable(this.backupId);
}
/**
* @return Unique Baremetal Tenant Identifier.
*
*/
public String baremetalTenantId() {
return this.baremetalTenantId;
}
/**
* @return Pool Resource Id used in case of creating a volume through volume group
*
*/
public Optional capacityPoolResourceId() {
return Optional.ofNullable(this.capacityPoolResourceId);
}
/**
* @return When a volume is being restored from another volume's snapshot, will show the percentage completion of this cloning process. When this value is empty/null there is no cloning process currently happening on this volume. This value will update every 5 minutes during cloning.
*
*/
public Integer cloneProgress() {
return this.cloneProgress;
}
/**
* @return Specifies whether Cool Access(tiering) is enabled for the volume.
*
*/
public Optional coolAccess() {
return Optional.ofNullable(this.coolAccess);
}
/**
* @return Specifies the number of days after which data that is not accessed by clients will be tiered.
*
*/
public Optional coolnessPeriod() {
return Optional.ofNullable(this.coolnessPeriod);
}
/**
* @return A unique file path for the volume. Used when creating mount targets
*
*/
public String creationToken() {
return this.creationToken;
}
/**
* @return DataProtection type volumes include an object containing details of the replication
*
*/
public Optional dataProtection() {
return Optional.ofNullable(this.dataProtection);
}
/**
* @return Data store resource unique identifier
*
*/
public List dataStoreResourceId() {
return this.dataStoreResourceId;
}
/**
* @return Default group quota for volume in KiBs. If isDefaultQuotaEnabled is set, the minimum value of 4 KiBs applies.
*
*/
public Optional defaultGroupQuotaInKiBs() {
return Optional.ofNullable(this.defaultGroupQuotaInKiBs);
}
/**
* @return Default user quota for volume in KiBs. If isDefaultQuotaEnabled is set, the minimum value of 4 KiBs applies .
*
*/
public Optional defaultUserQuotaInKiBs() {
return Optional.ofNullable(this.defaultUserQuotaInKiBs);
}
/**
* @return If enabled (true) the snapshot the volume was created from will be automatically deleted after the volume create operation has finished. Defaults to false
*
*/
public Optional deleteBaseSnapshot() {
return Optional.ofNullable(this.deleteBaseSnapshot);
}
/**
* @return Flag indicating whether subvolume operations are enabled on the volume
*
*/
public Optional enableSubvolumes() {
return Optional.ofNullable(this.enableSubvolumes);
}
/**
* @return Specifies if the volume is encrypted or not. Only available on volumes created or updated after 2022-01-01.
*
*/
public Boolean encrypted() {
return this.encrypted;
}
/**
* @return Source of key used to encrypt data in volume. Applicable if NetApp account has encryption.keySource = 'Microsoft.KeyVault'. Possible values (case-insensitive) are: 'Microsoft.NetApp, Microsoft.KeyVault'
*
*/
public Optional encryptionKeySource() {
return Optional.ofNullable(this.encryptionKeySource);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Set of export policy rules
*
*/
public Optional exportPolicy() {
return Optional.ofNullable(this.exportPolicy);
}
/**
* @return Flag indicating whether file access logs are enabled for the volume, based on active diagnostic settings present on the volume.
*
*/
public String fileAccessLogs() {
return this.fileAccessLogs;
}
/**
* @return Unique FileSystem Identifier.
*
*/
public String fileSystemId() {
return this.fileSystemId;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Specifies if default quota is enabled for the volume.
*
*/
public Optional isDefaultQuotaEnabled() {
return Optional.ofNullable(this.isDefaultQuotaEnabled);
}
/**
* @return Specifies whether volume is a Large Volume or Regular Volume.
*
*/
public Optional isLargeVolume() {
return Optional.ofNullable(this.isLargeVolume);
}
/**
* @return Restoring
*
*/
public Optional isRestoring() {
return Optional.ofNullable(this.isRestoring);
}
/**
* @return Describe if a volume is KerberosEnabled. To be use with swagger version 2020-05-01 or later
*
*/
public Optional kerberosEnabled() {
return Optional.ofNullable(this.kerberosEnabled);
}
/**
* @return The resource ID of private endpoint for KeyVault. It must reside in the same VNET as the volume. Only applicable if encryptionKeySource = 'Microsoft.KeyVault'.
*
*/
public Optional keyVaultPrivateEndpointResourceId() {
return Optional.ofNullable(this.keyVaultPrivateEndpointResourceId);
}
/**
* @return Specifies whether LDAP is enabled or not for a given NFS volume.
*
*/
public Optional ldapEnabled() {
return Optional.ofNullable(this.ldapEnabled);
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return Maximum number of files allowed. Needs a service request in order to be changed. Only allowed to be changed if volume quota is more than 4TiB.
*
*/
public Double maximumNumberOfFiles() {
return this.maximumNumberOfFiles;
}
/**
* @return List of mount targets
*
*/
public List mountTargets() {
return this.mountTargets;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Basic network, or Standard features available to the volume.
*
*/
public Optional networkFeatures() {
return Optional.ofNullable(this.networkFeatures);
}
/**
* @return Network Sibling Set ID for the the group of volumes sharing networking resources.
*
*/
public String networkSiblingSetId() {
return this.networkSiblingSetId;
}
/**
* @return Id of the snapshot or backup that the volume is restored from.
*
*/
public String originatingResourceId() {
return this.originatingResourceId;
}
/**
* @return Application specific placement rules for the particular volume
*
*/
public List placementRules() {
return this.placementRules == null ? List.of() : this.placementRules;
}
/**
* @return Set of protocol types, default NFSv3, CIFS for SMB protocol
*
*/
public List protocolTypes() {
return this.protocolTypes == null ? List.of() : this.protocolTypes;
}
/**
* @return The availability zone where the volume is provisioned. This refers to the logical availability zone where the volume resides.
*
*/
public String provisionedAvailabilityZone() {
return this.provisionedAvailabilityZone;
}
/**
* @return Azure lifecycle management
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Proximity placement group associated with the volume
*
*/
public Optional proximityPlacementGroup() {
return Optional.ofNullable(this.proximityPlacementGroup);
}
/**
* @return The security style of volume, default unix, defaults to ntfs for dual protocol or CIFS protocol
*
*/
public Optional securityStyle() {
return Optional.ofNullable(this.securityStyle);
}
/**
* @return The service level of the file system
*
*/
public Optional serviceLevel() {
return Optional.ofNullable(this.serviceLevel);
}
/**
* @return Enables access based enumeration share property for SMB Shares. Only applicable for SMB/DualProtocol volume
*
*/
public Optional smbAccessBasedEnumeration() {
return Optional.ofNullable(this.smbAccessBasedEnumeration);
}
/**
* @return Enables continuously available share property for smb volume. Only applicable for SMB volume
*
*/
public Optional smbContinuouslyAvailable() {
return Optional.ofNullable(this.smbContinuouslyAvailable);
}
/**
* @return Enables encryption for in-flight smb3 data. Only applicable for SMB/DualProtocol volume. To be used with swagger version 2020-08-01 or later
*
*/
public Optional smbEncryption() {
return Optional.ofNullable(this.smbEncryption);
}
/**
* @return Enables non browsable property for SMB Shares. Only applicable for SMB/DualProtocol volume
*
*/
public Optional smbNonBrowsable() {
return Optional.ofNullable(this.smbNonBrowsable);
}
/**
* @return If enabled (true) the volume will contain a read-only snapshot directory which provides access to each of the volume's snapshots (defaults to true).
*
*/
public Optional snapshotDirectoryVisible() {
return Optional.ofNullable(this.snapshotDirectoryVisible);
}
/**
* @return UUID v4 or resource identifier used to identify the Snapshot.
*
*/
public Optional snapshotId() {
return Optional.ofNullable(this.snapshotId);
}
/**
* @return Provides storage to network proximity information for the volume.
*
*/
public String storageToNetworkProximity() {
return this.storageToNetworkProximity;
}
/**
* @return The Azure Resource URI for a delegated subnet. Must have the delegation Microsoft.NetApp/volumes
*
*/
public String subnetId() {
return this.subnetId;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return T2 network information
*
*/
public String t2Network() {
return this.t2Network;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
public Optional throughputMibps() {
return Optional.ofNullable(this.throughputMibps);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return UNIX permissions for NFS volume accepted in octal 4 digit format. First digit selects the set user ID(4), set group ID (2) and sticky (1) attributes. Second digit selects permission for the owner of the file: read (4), write (2) and execute (1). Third selects permissions for other users in the same group. the fourth for other users not in the group. 0755 - gives read/write/execute permissions to owner and read/execute to group and other users.
*
*/
public Optional unixPermissions() {
return Optional.ofNullable(this.unixPermissions);
}
/**
* @return Maximum storage quota allowed for a file system in bytes. This is a soft quota used for alerting only. Minimum size is 100 GiB. Upper limit is 100TiB, 500Tib for LargeVolume. Specified in bytes.
*
*/
public Double usageThreshold() {
return this.usageThreshold;
}
/**
* @return Volume Group Name
*
*/
public String volumeGroupName() {
return this.volumeGroupName;
}
/**
* @return Volume spec name is the application specific designation or identifier for the particular volume in a volume group for e.g. data, log
*
*/
public Optional volumeSpecName() {
return Optional.ofNullable(this.volumeSpecName);
}
/**
* @return What type of volume is this. For destination volumes in Cross Region Replication, set type to DataProtection
*
*/
public Optional volumeType() {
return Optional.ofNullable(this.volumeType);
}
/**
* @return Availability Zone
*
*/
public List zones() {
return this.zones == null ? List.of() : this.zones;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetVolumeResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double actualThroughputMibps;
private @Nullable String avsDataStore;
private @Nullable String backupId;
private String baremetalTenantId;
private @Nullable String capacityPoolResourceId;
private Integer cloneProgress;
private @Nullable Boolean coolAccess;
private @Nullable Integer coolnessPeriod;
private String creationToken;
private @Nullable VolumePropertiesResponseDataProtection dataProtection;
private List dataStoreResourceId;
private @Nullable Double defaultGroupQuotaInKiBs;
private @Nullable Double defaultUserQuotaInKiBs;
private @Nullable Boolean deleteBaseSnapshot;
private @Nullable String enableSubvolumes;
private Boolean encrypted;
private @Nullable String encryptionKeySource;
private String etag;
private @Nullable VolumePropertiesResponseExportPolicy exportPolicy;
private String fileAccessLogs;
private String fileSystemId;
private String id;
private @Nullable Boolean isDefaultQuotaEnabled;
private @Nullable Boolean isLargeVolume;
private @Nullable Boolean isRestoring;
private @Nullable Boolean kerberosEnabled;
private @Nullable String keyVaultPrivateEndpointResourceId;
private @Nullable Boolean ldapEnabled;
private String location;
private Double maximumNumberOfFiles;
private List mountTargets;
private String name;
private @Nullable String networkFeatures;
private String networkSiblingSetId;
private String originatingResourceId;
private @Nullable List placementRules;
private @Nullable List protocolTypes;
private String provisionedAvailabilityZone;
private String provisioningState;
private @Nullable String proximityPlacementGroup;
private @Nullable String securityStyle;
private @Nullable String serviceLevel;
private @Nullable String smbAccessBasedEnumeration;
private @Nullable Boolean smbContinuouslyAvailable;
private @Nullable Boolean smbEncryption;
private @Nullable String smbNonBrowsable;
private @Nullable Boolean snapshotDirectoryVisible;
private @Nullable String snapshotId;
private String storageToNetworkProximity;
private String subnetId;
private SystemDataResponse systemData;
private String t2Network;
private @Nullable Map tags;
private @Nullable Double throughputMibps;
private String type;
private @Nullable String unixPermissions;
private Double usageThreshold;
private String volumeGroupName;
private @Nullable String volumeSpecName;
private @Nullable String volumeType;
private @Nullable List zones;
public Builder() {}
public Builder(GetVolumeResult defaults) {
Objects.requireNonNull(defaults);
this.actualThroughputMibps = defaults.actualThroughputMibps;
this.avsDataStore = defaults.avsDataStore;
this.backupId = defaults.backupId;
this.baremetalTenantId = defaults.baremetalTenantId;
this.capacityPoolResourceId = defaults.capacityPoolResourceId;
this.cloneProgress = defaults.cloneProgress;
this.coolAccess = defaults.coolAccess;
this.coolnessPeriod = defaults.coolnessPeriod;
this.creationToken = defaults.creationToken;
this.dataProtection = defaults.dataProtection;
this.dataStoreResourceId = defaults.dataStoreResourceId;
this.defaultGroupQuotaInKiBs = defaults.defaultGroupQuotaInKiBs;
this.defaultUserQuotaInKiBs = defaults.defaultUserQuotaInKiBs;
this.deleteBaseSnapshot = defaults.deleteBaseSnapshot;
this.enableSubvolumes = defaults.enableSubvolumes;
this.encrypted = defaults.encrypted;
this.encryptionKeySource = defaults.encryptionKeySource;
this.etag = defaults.etag;
this.exportPolicy = defaults.exportPolicy;
this.fileAccessLogs = defaults.fileAccessLogs;
this.fileSystemId = defaults.fileSystemId;
this.id = defaults.id;
this.isDefaultQuotaEnabled = defaults.isDefaultQuotaEnabled;
this.isLargeVolume = defaults.isLargeVolume;
this.isRestoring = defaults.isRestoring;
this.kerberosEnabled = defaults.kerberosEnabled;
this.keyVaultPrivateEndpointResourceId = defaults.keyVaultPrivateEndpointResourceId;
this.ldapEnabled = defaults.ldapEnabled;
this.location = defaults.location;
this.maximumNumberOfFiles = defaults.maximumNumberOfFiles;
this.mountTargets = defaults.mountTargets;
this.name = defaults.name;
this.networkFeatures = defaults.networkFeatures;
this.networkSiblingSetId = defaults.networkSiblingSetId;
this.originatingResourceId = defaults.originatingResourceId;
this.placementRules = defaults.placementRules;
this.protocolTypes = defaults.protocolTypes;
this.provisionedAvailabilityZone = defaults.provisionedAvailabilityZone;
this.provisioningState = defaults.provisioningState;
this.proximityPlacementGroup = defaults.proximityPlacementGroup;
this.securityStyle = defaults.securityStyle;
this.serviceLevel = defaults.serviceLevel;
this.smbAccessBasedEnumeration = defaults.smbAccessBasedEnumeration;
this.smbContinuouslyAvailable = defaults.smbContinuouslyAvailable;
this.smbEncryption = defaults.smbEncryption;
this.smbNonBrowsable = defaults.smbNonBrowsable;
this.snapshotDirectoryVisible = defaults.snapshotDirectoryVisible;
this.snapshotId = defaults.snapshotId;
this.storageToNetworkProximity = defaults.storageToNetworkProximity;
this.subnetId = defaults.subnetId;
this.systemData = defaults.systemData;
this.t2Network = defaults.t2Network;
this.tags = defaults.tags;
this.throughputMibps = defaults.throughputMibps;
this.type = defaults.type;
this.unixPermissions = defaults.unixPermissions;
this.usageThreshold = defaults.usageThreshold;
this.volumeGroupName = defaults.volumeGroupName;
this.volumeSpecName = defaults.volumeSpecName;
this.volumeType = defaults.volumeType;
this.zones = defaults.zones;
}
@CustomType.Setter
public Builder actualThroughputMibps(Double actualThroughputMibps) {
if (actualThroughputMibps == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "actualThroughputMibps");
}
this.actualThroughputMibps = actualThroughputMibps;
return this;
}
@CustomType.Setter
public Builder avsDataStore(@Nullable String avsDataStore) {
this.avsDataStore = avsDataStore;
return this;
}
@CustomType.Setter
public Builder backupId(@Nullable String backupId) {
this.backupId = backupId;
return this;
}
@CustomType.Setter
public Builder baremetalTenantId(String baremetalTenantId) {
if (baremetalTenantId == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "baremetalTenantId");
}
this.baremetalTenantId = baremetalTenantId;
return this;
}
@CustomType.Setter
public Builder capacityPoolResourceId(@Nullable String capacityPoolResourceId) {
this.capacityPoolResourceId = capacityPoolResourceId;
return this;
}
@CustomType.Setter
public Builder cloneProgress(Integer cloneProgress) {
if (cloneProgress == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "cloneProgress");
}
this.cloneProgress = cloneProgress;
return this;
}
@CustomType.Setter
public Builder coolAccess(@Nullable Boolean coolAccess) {
this.coolAccess = coolAccess;
return this;
}
@CustomType.Setter
public Builder coolnessPeriod(@Nullable Integer coolnessPeriod) {
this.coolnessPeriod = coolnessPeriod;
return this;
}
@CustomType.Setter
public Builder creationToken(String creationToken) {
if (creationToken == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "creationToken");
}
this.creationToken = creationToken;
return this;
}
@CustomType.Setter
public Builder dataProtection(@Nullable VolumePropertiesResponseDataProtection dataProtection) {
this.dataProtection = dataProtection;
return this;
}
@CustomType.Setter
public Builder dataStoreResourceId(List dataStoreResourceId) {
if (dataStoreResourceId == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "dataStoreResourceId");
}
this.dataStoreResourceId = dataStoreResourceId;
return this;
}
public Builder dataStoreResourceId(String... dataStoreResourceId) {
return dataStoreResourceId(List.of(dataStoreResourceId));
}
@CustomType.Setter
public Builder defaultGroupQuotaInKiBs(@Nullable Double defaultGroupQuotaInKiBs) {
this.defaultGroupQuotaInKiBs = defaultGroupQuotaInKiBs;
return this;
}
@CustomType.Setter
public Builder defaultUserQuotaInKiBs(@Nullable Double defaultUserQuotaInKiBs) {
this.defaultUserQuotaInKiBs = defaultUserQuotaInKiBs;
return this;
}
@CustomType.Setter
public Builder deleteBaseSnapshot(@Nullable Boolean deleteBaseSnapshot) {
this.deleteBaseSnapshot = deleteBaseSnapshot;
return this;
}
@CustomType.Setter
public Builder enableSubvolumes(@Nullable String enableSubvolumes) {
this.enableSubvolumes = enableSubvolumes;
return this;
}
@CustomType.Setter
public Builder encrypted(Boolean encrypted) {
if (encrypted == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "encrypted");
}
this.encrypted = encrypted;
return this;
}
@CustomType.Setter
public Builder encryptionKeySource(@Nullable String encryptionKeySource) {
this.encryptionKeySource = encryptionKeySource;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder exportPolicy(@Nullable VolumePropertiesResponseExportPolicy exportPolicy) {
this.exportPolicy = exportPolicy;
return this;
}
@CustomType.Setter
public Builder fileAccessLogs(String fileAccessLogs) {
if (fileAccessLogs == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "fileAccessLogs");
}
this.fileAccessLogs = fileAccessLogs;
return this;
}
@CustomType.Setter
public Builder fileSystemId(String fileSystemId) {
if (fileSystemId == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "fileSystemId");
}
this.fileSystemId = fileSystemId;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isDefaultQuotaEnabled(@Nullable Boolean isDefaultQuotaEnabled) {
this.isDefaultQuotaEnabled = isDefaultQuotaEnabled;
return this;
}
@CustomType.Setter
public Builder isLargeVolume(@Nullable Boolean isLargeVolume) {
this.isLargeVolume = isLargeVolume;
return this;
}
@CustomType.Setter
public Builder isRestoring(@Nullable Boolean isRestoring) {
this.isRestoring = isRestoring;
return this;
}
@CustomType.Setter
public Builder kerberosEnabled(@Nullable Boolean kerberosEnabled) {
this.kerberosEnabled = kerberosEnabled;
return this;
}
@CustomType.Setter
public Builder keyVaultPrivateEndpointResourceId(@Nullable String keyVaultPrivateEndpointResourceId) {
this.keyVaultPrivateEndpointResourceId = keyVaultPrivateEndpointResourceId;
return this;
}
@CustomType.Setter
public Builder ldapEnabled(@Nullable Boolean ldapEnabled) {
this.ldapEnabled = ldapEnabled;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder maximumNumberOfFiles(Double maximumNumberOfFiles) {
if (maximumNumberOfFiles == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "maximumNumberOfFiles");
}
this.maximumNumberOfFiles = maximumNumberOfFiles;
return this;
}
@CustomType.Setter
public Builder mountTargets(List mountTargets) {
if (mountTargets == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "mountTargets");
}
this.mountTargets = mountTargets;
return this;
}
public Builder mountTargets(MountTargetPropertiesResponse... mountTargets) {
return mountTargets(List.of(mountTargets));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder networkFeatures(@Nullable String networkFeatures) {
this.networkFeatures = networkFeatures;
return this;
}
@CustomType.Setter
public Builder networkSiblingSetId(String networkSiblingSetId) {
if (networkSiblingSetId == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "networkSiblingSetId");
}
this.networkSiblingSetId = networkSiblingSetId;
return this;
}
@CustomType.Setter
public Builder originatingResourceId(String originatingResourceId) {
if (originatingResourceId == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "originatingResourceId");
}
this.originatingResourceId = originatingResourceId;
return this;
}
@CustomType.Setter
public Builder placementRules(@Nullable List placementRules) {
this.placementRules = placementRules;
return this;
}
public Builder placementRules(PlacementKeyValuePairsResponse... placementRules) {
return placementRules(List.of(placementRules));
}
@CustomType.Setter
public Builder protocolTypes(@Nullable List protocolTypes) {
this.protocolTypes = protocolTypes;
return this;
}
public Builder protocolTypes(String... protocolTypes) {
return protocolTypes(List.of(protocolTypes));
}
@CustomType.Setter
public Builder provisionedAvailabilityZone(String provisionedAvailabilityZone) {
if (provisionedAvailabilityZone == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "provisionedAvailabilityZone");
}
this.provisionedAvailabilityZone = provisionedAvailabilityZone;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder proximityPlacementGroup(@Nullable String proximityPlacementGroup) {
this.proximityPlacementGroup = proximityPlacementGroup;
return this;
}
@CustomType.Setter
public Builder securityStyle(@Nullable String securityStyle) {
this.securityStyle = securityStyle;
return this;
}
@CustomType.Setter
public Builder serviceLevel(@Nullable String serviceLevel) {
this.serviceLevel = serviceLevel;
return this;
}
@CustomType.Setter
public Builder smbAccessBasedEnumeration(@Nullable String smbAccessBasedEnumeration) {
this.smbAccessBasedEnumeration = smbAccessBasedEnumeration;
return this;
}
@CustomType.Setter
public Builder smbContinuouslyAvailable(@Nullable Boolean smbContinuouslyAvailable) {
this.smbContinuouslyAvailable = smbContinuouslyAvailable;
return this;
}
@CustomType.Setter
public Builder smbEncryption(@Nullable Boolean smbEncryption) {
this.smbEncryption = smbEncryption;
return this;
}
@CustomType.Setter
public Builder smbNonBrowsable(@Nullable String smbNonBrowsable) {
this.smbNonBrowsable = smbNonBrowsable;
return this;
}
@CustomType.Setter
public Builder snapshotDirectoryVisible(@Nullable Boolean snapshotDirectoryVisible) {
this.snapshotDirectoryVisible = snapshotDirectoryVisible;
return this;
}
@CustomType.Setter
public Builder snapshotId(@Nullable String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
@CustomType.Setter
public Builder storageToNetworkProximity(String storageToNetworkProximity) {
if (storageToNetworkProximity == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "storageToNetworkProximity");
}
this.storageToNetworkProximity = storageToNetworkProximity;
return this;
}
@CustomType.Setter
public Builder subnetId(String subnetId) {
if (subnetId == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "subnetId");
}
this.subnetId = subnetId;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder t2Network(String t2Network) {
if (t2Network == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "t2Network");
}
this.t2Network = t2Network;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder throughputMibps(@Nullable Double throughputMibps) {
this.throughputMibps = throughputMibps;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder unixPermissions(@Nullable String unixPermissions) {
this.unixPermissions = unixPermissions;
return this;
}
@CustomType.Setter
public Builder usageThreshold(Double usageThreshold) {
if (usageThreshold == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "usageThreshold");
}
this.usageThreshold = usageThreshold;
return this;
}
@CustomType.Setter
public Builder volumeGroupName(String volumeGroupName) {
if (volumeGroupName == null) {
throw new MissingRequiredPropertyException("GetVolumeResult", "volumeGroupName");
}
this.volumeGroupName = volumeGroupName;
return this;
}
@CustomType.Setter
public Builder volumeSpecName(@Nullable String volumeSpecName) {
this.volumeSpecName = volumeSpecName;
return this;
}
@CustomType.Setter
public Builder volumeType(@Nullable String volumeType) {
this.volumeType = volumeType;
return this;
}
@CustomType.Setter
public Builder zones(@Nullable List zones) {
this.zones = zones;
return this;
}
public Builder zones(String... zones) {
return zones(List.of(zones));
}
public GetVolumeResult build() {
final var _resultValue = new GetVolumeResult();
_resultValue.actualThroughputMibps = actualThroughputMibps;
_resultValue.avsDataStore = avsDataStore;
_resultValue.backupId = backupId;
_resultValue.baremetalTenantId = baremetalTenantId;
_resultValue.capacityPoolResourceId = capacityPoolResourceId;
_resultValue.cloneProgress = cloneProgress;
_resultValue.coolAccess = coolAccess;
_resultValue.coolnessPeriod = coolnessPeriod;
_resultValue.creationToken = creationToken;
_resultValue.dataProtection = dataProtection;
_resultValue.dataStoreResourceId = dataStoreResourceId;
_resultValue.defaultGroupQuotaInKiBs = defaultGroupQuotaInKiBs;
_resultValue.defaultUserQuotaInKiBs = defaultUserQuotaInKiBs;
_resultValue.deleteBaseSnapshot = deleteBaseSnapshot;
_resultValue.enableSubvolumes = enableSubvolumes;
_resultValue.encrypted = encrypted;
_resultValue.encryptionKeySource = encryptionKeySource;
_resultValue.etag = etag;
_resultValue.exportPolicy = exportPolicy;
_resultValue.fileAccessLogs = fileAccessLogs;
_resultValue.fileSystemId = fileSystemId;
_resultValue.id = id;
_resultValue.isDefaultQuotaEnabled = isDefaultQuotaEnabled;
_resultValue.isLargeVolume = isLargeVolume;
_resultValue.isRestoring = isRestoring;
_resultValue.kerberosEnabled = kerberosEnabled;
_resultValue.keyVaultPrivateEndpointResourceId = keyVaultPrivateEndpointResourceId;
_resultValue.ldapEnabled = ldapEnabled;
_resultValue.location = location;
_resultValue.maximumNumberOfFiles = maximumNumberOfFiles;
_resultValue.mountTargets = mountTargets;
_resultValue.name = name;
_resultValue.networkFeatures = networkFeatures;
_resultValue.networkSiblingSetId = networkSiblingSetId;
_resultValue.originatingResourceId = originatingResourceId;
_resultValue.placementRules = placementRules;
_resultValue.protocolTypes = protocolTypes;
_resultValue.provisionedAvailabilityZone = provisionedAvailabilityZone;
_resultValue.provisioningState = provisioningState;
_resultValue.proximityPlacementGroup = proximityPlacementGroup;
_resultValue.securityStyle = securityStyle;
_resultValue.serviceLevel = serviceLevel;
_resultValue.smbAccessBasedEnumeration = smbAccessBasedEnumeration;
_resultValue.smbContinuouslyAvailable = smbContinuouslyAvailable;
_resultValue.smbEncryption = smbEncryption;
_resultValue.smbNonBrowsable = smbNonBrowsable;
_resultValue.snapshotDirectoryVisible = snapshotDirectoryVisible;
_resultValue.snapshotId = snapshotId;
_resultValue.storageToNetworkProximity = storageToNetworkProximity;
_resultValue.subnetId = subnetId;
_resultValue.systemData = systemData;
_resultValue.t2Network = t2Network;
_resultValue.tags = tags;
_resultValue.throughputMibps = throughputMibps;
_resultValue.type = type;
_resultValue.unixPermissions = unixPermissions;
_resultValue.usageThreshold = usageThreshold;
_resultValue.volumeGroupName = volumeGroupName;
_resultValue.volumeSpecName = volumeSpecName;
_resultValue.volumeType = volumeType;
_resultValue.zones = zones;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy