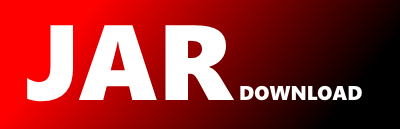
com.pulumi.azurenative.network.inputs.EndpointArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.AlwaysServe;
import com.pulumi.azurenative.network.enums.EndpointMonitorStatus;
import com.pulumi.azurenative.network.enums.EndpointStatus;
import com.pulumi.azurenative.network.inputs.EndpointPropertiesCustomHeadersArgs;
import com.pulumi.azurenative.network.inputs.EndpointPropertiesSubnetsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Class representing a Traffic Manager endpoint.
*
*/
public final class EndpointArgs extends com.pulumi.resources.ResourceArgs {
public static final EndpointArgs Empty = new EndpointArgs();
/**
* If Always Serve is enabled, probing for endpoint health will be disabled and endpoints will be included in the traffic routing method.
*
*/
@Import(name="alwaysServe")
private @Nullable Output> alwaysServe;
/**
* @return If Always Serve is enabled, probing for endpoint health will be disabled and endpoints will be included in the traffic routing method.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy