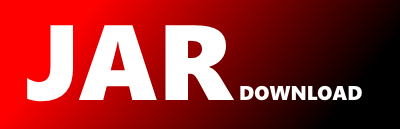
com.pulumi.azurenative.network.inputs.LoadBalancingRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.LoadDistribution;
import com.pulumi.azurenative.network.enums.TransportProtocol;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A load balancing rule for a load balancer.
*
*/
public final class LoadBalancingRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final LoadBalancingRuleArgs Empty = new LoadBalancingRuleArgs();
/**
* A reference to a pool of DIPs. Inbound traffic is randomly load balanced across IPs in the backend IPs.
*
*/
@Import(name="backendAddressPool")
private @Nullable Output backendAddressPool;
/**
* @return A reference to a pool of DIPs. Inbound traffic is randomly load balanced across IPs in the backend IPs.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy