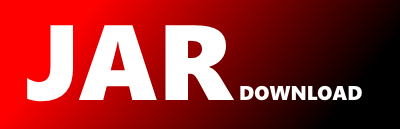
com.pulumi.azurenative.network.outputs.GetFrontDoorResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.BackendPoolResponse;
import com.pulumi.azurenative.network.outputs.BackendPoolsSettingsResponse;
import com.pulumi.azurenative.network.outputs.FrontendEndpointResponse;
import com.pulumi.azurenative.network.outputs.HealthProbeSettingsModelResponse;
import com.pulumi.azurenative.network.outputs.LoadBalancingSettingsModelResponse;
import com.pulumi.azurenative.network.outputs.RoutingRuleResponse;
import com.pulumi.azurenative.network.outputs.RulesEngineResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetFrontDoorResult {
/**
* @return Backend pools available to routing rules.
*
*/
private @Nullable List backendPools;
/**
* @return Settings for all backendPools
*
*/
private @Nullable BackendPoolsSettingsResponse backendPoolsSettings;
/**
* @return The host that each frontendEndpoint must CNAME to.
*
*/
private String cname;
/**
* @return Operational status of the Front Door load balancer. Permitted values are 'Enabled' or 'Disabled'
*
*/
private @Nullable String enabledState;
/**
* @return Key-Value pair representing additional properties for frontdoor.
*
*/
private Map extendedProperties;
/**
* @return A friendly name for the frontDoor
*
*/
private @Nullable String friendlyName;
/**
* @return The Id of the frontdoor.
*
*/
private String frontdoorId;
/**
* @return Frontend endpoints available to routing rules.
*
*/
private @Nullable List frontendEndpoints;
/**
* @return Health probe settings associated with this Front Door instance.
*
*/
private @Nullable List healthProbeSettings;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return Load balancing settings associated with this Front Door instance.
*
*/
private @Nullable List loadBalancingSettings;
/**
* @return Resource location.
*
*/
private @Nullable String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Provisioning state of the Front Door.
*
*/
private String provisioningState;
/**
* @return Resource status of the Front Door.
*
*/
private String resourceState;
/**
* @return Routing rules associated with this Front Door.
*
*/
private @Nullable List routingRules;
/**
* @return Rules Engine Configurations available to routing rules.
*
*/
private List rulesEngines;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
private GetFrontDoorResult() {}
/**
* @return Backend pools available to routing rules.
*
*/
public List backendPools() {
return this.backendPools == null ? List.of() : this.backendPools;
}
/**
* @return Settings for all backendPools
*
*/
public Optional backendPoolsSettings() {
return Optional.ofNullable(this.backendPoolsSettings);
}
/**
* @return The host that each frontendEndpoint must CNAME to.
*
*/
public String cname() {
return this.cname;
}
/**
* @return Operational status of the Front Door load balancer. Permitted values are 'Enabled' or 'Disabled'
*
*/
public Optional enabledState() {
return Optional.ofNullable(this.enabledState);
}
/**
* @return Key-Value pair representing additional properties for frontdoor.
*
*/
public Map extendedProperties() {
return this.extendedProperties;
}
/**
* @return A friendly name for the frontDoor
*
*/
public Optional friendlyName() {
return Optional.ofNullable(this.friendlyName);
}
/**
* @return The Id of the frontdoor.
*
*/
public String frontdoorId() {
return this.frontdoorId;
}
/**
* @return Frontend endpoints available to routing rules.
*
*/
public List frontendEndpoints() {
return this.frontendEndpoints == null ? List.of() : this.frontendEndpoints;
}
/**
* @return Health probe settings associated with this Front Door instance.
*
*/
public List healthProbeSettings() {
return this.healthProbeSettings == null ? List.of() : this.healthProbeSettings;
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return Load balancing settings associated with this Front Door instance.
*
*/
public List loadBalancingSettings() {
return this.loadBalancingSettings == null ? List.of() : this.loadBalancingSettings;
}
/**
* @return Resource location.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Provisioning state of the Front Door.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Resource status of the Front Door.
*
*/
public String resourceState() {
return this.resourceState;
}
/**
* @return Routing rules associated with this Front Door.
*
*/
public List routingRules() {
return this.routingRules == null ? List.of() : this.routingRules;
}
/**
* @return Rules Engine Configurations available to routing rules.
*
*/
public List rulesEngines() {
return this.rulesEngines;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetFrontDoorResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List backendPools;
private @Nullable BackendPoolsSettingsResponse backendPoolsSettings;
private String cname;
private @Nullable String enabledState;
private Map extendedProperties;
private @Nullable String friendlyName;
private String frontdoorId;
private @Nullable List frontendEndpoints;
private @Nullable List healthProbeSettings;
private String id;
private @Nullable List loadBalancingSettings;
private @Nullable String location;
private String name;
private String provisioningState;
private String resourceState;
private @Nullable List routingRules;
private List rulesEngines;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetFrontDoorResult defaults) {
Objects.requireNonNull(defaults);
this.backendPools = defaults.backendPools;
this.backendPoolsSettings = defaults.backendPoolsSettings;
this.cname = defaults.cname;
this.enabledState = defaults.enabledState;
this.extendedProperties = defaults.extendedProperties;
this.friendlyName = defaults.friendlyName;
this.frontdoorId = defaults.frontdoorId;
this.frontendEndpoints = defaults.frontendEndpoints;
this.healthProbeSettings = defaults.healthProbeSettings;
this.id = defaults.id;
this.loadBalancingSettings = defaults.loadBalancingSettings;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.resourceState = defaults.resourceState;
this.routingRules = defaults.routingRules;
this.rulesEngines = defaults.rulesEngines;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder backendPools(@Nullable List backendPools) {
this.backendPools = backendPools;
return this;
}
public Builder backendPools(BackendPoolResponse... backendPools) {
return backendPools(List.of(backendPools));
}
@CustomType.Setter
public Builder backendPoolsSettings(@Nullable BackendPoolsSettingsResponse backendPoolsSettings) {
this.backendPoolsSettings = backendPoolsSettings;
return this;
}
@CustomType.Setter
public Builder cname(String cname) {
if (cname == null) {
throw new MissingRequiredPropertyException("GetFrontDoorResult", "cname");
}
this.cname = cname;
return this;
}
@CustomType.Setter
public Builder enabledState(@Nullable String enabledState) {
this.enabledState = enabledState;
return this;
}
@CustomType.Setter
public Builder extendedProperties(Map extendedProperties) {
if (extendedProperties == null) {
throw new MissingRequiredPropertyException("GetFrontDoorResult", "extendedProperties");
}
this.extendedProperties = extendedProperties;
return this;
}
@CustomType.Setter
public Builder friendlyName(@Nullable String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
@CustomType.Setter
public Builder frontdoorId(String frontdoorId) {
if (frontdoorId == null) {
throw new MissingRequiredPropertyException("GetFrontDoorResult", "frontdoorId");
}
this.frontdoorId = frontdoorId;
return this;
}
@CustomType.Setter
public Builder frontendEndpoints(@Nullable List frontendEndpoints) {
this.frontendEndpoints = frontendEndpoints;
return this;
}
public Builder frontendEndpoints(FrontendEndpointResponse... frontendEndpoints) {
return frontendEndpoints(List.of(frontendEndpoints));
}
@CustomType.Setter
public Builder healthProbeSettings(@Nullable List healthProbeSettings) {
this.healthProbeSettings = healthProbeSettings;
return this;
}
public Builder healthProbeSettings(HealthProbeSettingsModelResponse... healthProbeSettings) {
return healthProbeSettings(List.of(healthProbeSettings));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetFrontDoorResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder loadBalancingSettings(@Nullable List loadBalancingSettings) {
this.loadBalancingSettings = loadBalancingSettings;
return this;
}
public Builder loadBalancingSettings(LoadBalancingSettingsModelResponse... loadBalancingSettings) {
return loadBalancingSettings(List.of(loadBalancingSettings));
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetFrontDoorResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetFrontDoorResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder resourceState(String resourceState) {
if (resourceState == null) {
throw new MissingRequiredPropertyException("GetFrontDoorResult", "resourceState");
}
this.resourceState = resourceState;
return this;
}
@CustomType.Setter
public Builder routingRules(@Nullable List routingRules) {
this.routingRules = routingRules;
return this;
}
public Builder routingRules(RoutingRuleResponse... routingRules) {
return routingRules(List.of(routingRules));
}
@CustomType.Setter
public Builder rulesEngines(List rulesEngines) {
if (rulesEngines == null) {
throw new MissingRequiredPropertyException("GetFrontDoorResult", "rulesEngines");
}
this.rulesEngines = rulesEngines;
return this;
}
public Builder rulesEngines(RulesEngineResponse... rulesEngines) {
return rulesEngines(List.of(rulesEngines));
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetFrontDoorResult", "type");
}
this.type = type;
return this;
}
public GetFrontDoorResult build() {
final var _resultValue = new GetFrontDoorResult();
_resultValue.backendPools = backendPools;
_resultValue.backendPoolsSettings = backendPoolsSettings;
_resultValue.cname = cname;
_resultValue.enabledState = enabledState;
_resultValue.extendedProperties = extendedProperties;
_resultValue.friendlyName = friendlyName;
_resultValue.frontdoorId = frontdoorId;
_resultValue.frontendEndpoints = frontendEndpoints;
_resultValue.healthProbeSettings = healthProbeSettings;
_resultValue.id = id;
_resultValue.loadBalancingSettings = loadBalancingSettings;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.resourceState = resourceState;
_resultValue.routingRules = routingRules;
_resultValue.rulesEngines = rulesEngines;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy