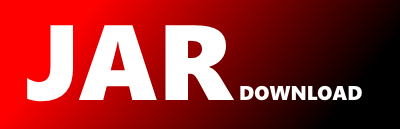
com.pulumi.azurenative.network.outputs.GetLoadBalancerBackendAddressPoolResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.GatewayLoadBalancerTunnelInterfaceResponse;
import com.pulumi.azurenative.network.outputs.LoadBalancerBackendAddressResponse;
import com.pulumi.azurenative.network.outputs.NetworkInterfaceIPConfigurationResponse;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetLoadBalancerBackendAddressPoolResult {
/**
* @return An array of references to IP addresses defined in network interfaces.
*
*/
private List backendIPConfigurations;
/**
* @return Amount of seconds Load Balancer waits for before sending RESET to client and backend address.
*
*/
private @Nullable Integer drainPeriodInSeconds;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return An array of references to inbound NAT rules that use this backend address pool.
*
*/
private List inboundNatRules;
/**
* @return An array of backend addresses.
*
*/
private @Nullable List loadBalancerBackendAddresses;
/**
* @return An array of references to load balancing rules that use this backend address pool.
*
*/
private List loadBalancingRules;
/**
* @return The location of the backend address pool.
*
*/
private @Nullable String location;
/**
* @return The name of the resource that is unique within the set of backend address pools used by the load balancer. This name can be used to access the resource.
*
*/
private @Nullable String name;
/**
* @return A reference to an outbound rule that uses this backend address pool.
*
*/
private SubResourceResponse outboundRule;
/**
* @return An array of references to outbound rules that use this backend address pool.
*
*/
private List outboundRules;
/**
* @return The provisioning state of the backend address pool resource.
*
*/
private String provisioningState;
/**
* @return An array of gateway load balancer tunnel interfaces.
*
*/
private @Nullable List tunnelInterfaces;
/**
* @return Type of the resource.
*
*/
private String type;
/**
* @return A reference to a virtual network.
*
*/
private @Nullable SubResourceResponse virtualNetwork;
private GetLoadBalancerBackendAddressPoolResult() {}
/**
* @return An array of references to IP addresses defined in network interfaces.
*
*/
public List backendIPConfigurations() {
return this.backendIPConfigurations;
}
/**
* @return Amount of seconds Load Balancer waits for before sending RESET to client and backend address.
*
*/
public Optional drainPeriodInSeconds() {
return Optional.ofNullable(this.drainPeriodInSeconds);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return An array of references to inbound NAT rules that use this backend address pool.
*
*/
public List inboundNatRules() {
return this.inboundNatRules;
}
/**
* @return An array of backend addresses.
*
*/
public List loadBalancerBackendAddresses() {
return this.loadBalancerBackendAddresses == null ? List.of() : this.loadBalancerBackendAddresses;
}
/**
* @return An array of references to load balancing rules that use this backend address pool.
*
*/
public List loadBalancingRules() {
return this.loadBalancingRules;
}
/**
* @return The location of the backend address pool.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return The name of the resource that is unique within the set of backend address pools used by the load balancer. This name can be used to access the resource.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return A reference to an outbound rule that uses this backend address pool.
*
*/
public SubResourceResponse outboundRule() {
return this.outboundRule;
}
/**
* @return An array of references to outbound rules that use this backend address pool.
*
*/
public List outboundRules() {
return this.outboundRules;
}
/**
* @return The provisioning state of the backend address pool resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return An array of gateway load balancer tunnel interfaces.
*
*/
public List tunnelInterfaces() {
return this.tunnelInterfaces == null ? List.of() : this.tunnelInterfaces;
}
/**
* @return Type of the resource.
*
*/
public String type() {
return this.type;
}
/**
* @return A reference to a virtual network.
*
*/
public Optional virtualNetwork() {
return Optional.ofNullable(this.virtualNetwork);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetLoadBalancerBackendAddressPoolResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List backendIPConfigurations;
private @Nullable Integer drainPeriodInSeconds;
private String etag;
private @Nullable String id;
private List inboundNatRules;
private @Nullable List loadBalancerBackendAddresses;
private List loadBalancingRules;
private @Nullable String location;
private @Nullable String name;
private SubResourceResponse outboundRule;
private List outboundRules;
private String provisioningState;
private @Nullable List tunnelInterfaces;
private String type;
private @Nullable SubResourceResponse virtualNetwork;
public Builder() {}
public Builder(GetLoadBalancerBackendAddressPoolResult defaults) {
Objects.requireNonNull(defaults);
this.backendIPConfigurations = defaults.backendIPConfigurations;
this.drainPeriodInSeconds = defaults.drainPeriodInSeconds;
this.etag = defaults.etag;
this.id = defaults.id;
this.inboundNatRules = defaults.inboundNatRules;
this.loadBalancerBackendAddresses = defaults.loadBalancerBackendAddresses;
this.loadBalancingRules = defaults.loadBalancingRules;
this.location = defaults.location;
this.name = defaults.name;
this.outboundRule = defaults.outboundRule;
this.outboundRules = defaults.outboundRules;
this.provisioningState = defaults.provisioningState;
this.tunnelInterfaces = defaults.tunnelInterfaces;
this.type = defaults.type;
this.virtualNetwork = defaults.virtualNetwork;
}
@CustomType.Setter
public Builder backendIPConfigurations(List backendIPConfigurations) {
if (backendIPConfigurations == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerBackendAddressPoolResult", "backendIPConfigurations");
}
this.backendIPConfigurations = backendIPConfigurations;
return this;
}
public Builder backendIPConfigurations(NetworkInterfaceIPConfigurationResponse... backendIPConfigurations) {
return backendIPConfigurations(List.of(backendIPConfigurations));
}
@CustomType.Setter
public Builder drainPeriodInSeconds(@Nullable Integer drainPeriodInSeconds) {
this.drainPeriodInSeconds = drainPeriodInSeconds;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerBackendAddressPoolResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder inboundNatRules(List inboundNatRules) {
if (inboundNatRules == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerBackendAddressPoolResult", "inboundNatRules");
}
this.inboundNatRules = inboundNatRules;
return this;
}
public Builder inboundNatRules(SubResourceResponse... inboundNatRules) {
return inboundNatRules(List.of(inboundNatRules));
}
@CustomType.Setter
public Builder loadBalancerBackendAddresses(@Nullable List loadBalancerBackendAddresses) {
this.loadBalancerBackendAddresses = loadBalancerBackendAddresses;
return this;
}
public Builder loadBalancerBackendAddresses(LoadBalancerBackendAddressResponse... loadBalancerBackendAddresses) {
return loadBalancerBackendAddresses(List.of(loadBalancerBackendAddresses));
}
@CustomType.Setter
public Builder loadBalancingRules(List loadBalancingRules) {
if (loadBalancingRules == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerBackendAddressPoolResult", "loadBalancingRules");
}
this.loadBalancingRules = loadBalancingRules;
return this;
}
public Builder loadBalancingRules(SubResourceResponse... loadBalancingRules) {
return loadBalancingRules(List.of(loadBalancingRules));
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder outboundRule(SubResourceResponse outboundRule) {
if (outboundRule == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerBackendAddressPoolResult", "outboundRule");
}
this.outboundRule = outboundRule;
return this;
}
@CustomType.Setter
public Builder outboundRules(List outboundRules) {
if (outboundRules == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerBackendAddressPoolResult", "outboundRules");
}
this.outboundRules = outboundRules;
return this;
}
public Builder outboundRules(SubResourceResponse... outboundRules) {
return outboundRules(List.of(outboundRules));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerBackendAddressPoolResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder tunnelInterfaces(@Nullable List tunnelInterfaces) {
this.tunnelInterfaces = tunnelInterfaces;
return this;
}
public Builder tunnelInterfaces(GatewayLoadBalancerTunnelInterfaceResponse... tunnelInterfaces) {
return tunnelInterfaces(List.of(tunnelInterfaces));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetLoadBalancerBackendAddressPoolResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder virtualNetwork(@Nullable SubResourceResponse virtualNetwork) {
this.virtualNetwork = virtualNetwork;
return this;
}
public GetLoadBalancerBackendAddressPoolResult build() {
final var _resultValue = new GetLoadBalancerBackendAddressPoolResult();
_resultValue.backendIPConfigurations = backendIPConfigurations;
_resultValue.drainPeriodInSeconds = drainPeriodInSeconds;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.inboundNatRules = inboundNatRules;
_resultValue.loadBalancerBackendAddresses = loadBalancerBackendAddresses;
_resultValue.loadBalancingRules = loadBalancingRules;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.outboundRule = outboundRule;
_resultValue.outboundRules = outboundRules;
_resultValue.provisioningState = provisioningState;
_resultValue.tunnelInterfaces = tunnelInterfaces;
_resultValue.type = type;
_resultValue.virtualNetwork = virtualNetwork;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy