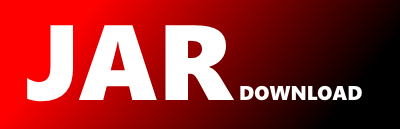
com.pulumi.azurenative.network.outputs.InboundNatRuleResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.NetworkInterfaceIPConfigurationResponse;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InboundNatRuleResponse {
/**
* @return A reference to backendAddressPool resource.
*
*/
private @Nullable SubResourceResponse backendAddressPool;
/**
* @return A reference to a private IP address defined on a network interface of a VM. Traffic sent to the frontend port of each of the frontend IP configurations is forwarded to the backend IP.
*
*/
private NetworkInterfaceIPConfigurationResponse backendIPConfiguration;
/**
* @return The port used for the internal endpoint. Acceptable values range from 1 to 65535.
*
*/
private @Nullable Integer backendPort;
/**
* @return Configures a virtual machine's endpoint for the floating IP capability required to configure a SQL AlwaysOn Availability Group. This setting is required when using the SQL AlwaysOn Availability Groups in SQL server. This setting can't be changed after you create the endpoint.
*
*/
private @Nullable Boolean enableFloatingIP;
/**
* @return Receive bidirectional TCP Reset on TCP flow idle timeout or unexpected connection termination. This element is only used when the protocol is set to TCP.
*
*/
private @Nullable Boolean enableTcpReset;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return A reference to frontend IP addresses.
*
*/
private @Nullable SubResourceResponse frontendIPConfiguration;
/**
* @return The port for the external endpoint. Port numbers for each rule must be unique within the Load Balancer. Acceptable values range from 1 to 65534.
*
*/
private @Nullable Integer frontendPort;
/**
* @return The port range end for the external endpoint. This property is used together with BackendAddressPool and FrontendPortRangeStart. Individual inbound NAT rule port mappings will be created for each backend address from BackendAddressPool. Acceptable values range from 1 to 65534.
*
*/
private @Nullable Integer frontendPortRangeEnd;
/**
* @return The port range start for the external endpoint. This property is used together with BackendAddressPool and FrontendPortRangeEnd. Individual inbound NAT rule port mappings will be created for each backend address from BackendAddressPool. Acceptable values range from 1 to 65534.
*
*/
private @Nullable Integer frontendPortRangeStart;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return The timeout for the TCP idle connection. The value can be set between 4 and 30 minutes. The default value is 4 minutes. This element is only used when the protocol is set to TCP.
*
*/
private @Nullable Integer idleTimeoutInMinutes;
/**
* @return The name of the resource that is unique within the set of inbound NAT rules used by the load balancer. This name can be used to access the resource.
*
*/
private @Nullable String name;
/**
* @return The reference to the transport protocol used by the load balancing rule.
*
*/
private @Nullable String protocol;
/**
* @return The provisioning state of the inbound NAT rule resource.
*
*/
private String provisioningState;
/**
* @return Type of the resource.
*
*/
private String type;
private InboundNatRuleResponse() {}
/**
* @return A reference to backendAddressPool resource.
*
*/
public Optional backendAddressPool() {
return Optional.ofNullable(this.backendAddressPool);
}
/**
* @return A reference to a private IP address defined on a network interface of a VM. Traffic sent to the frontend port of each of the frontend IP configurations is forwarded to the backend IP.
*
*/
public NetworkInterfaceIPConfigurationResponse backendIPConfiguration() {
return this.backendIPConfiguration;
}
/**
* @return The port used for the internal endpoint. Acceptable values range from 1 to 65535.
*
*/
public Optional backendPort() {
return Optional.ofNullable(this.backendPort);
}
/**
* @return Configures a virtual machine's endpoint for the floating IP capability required to configure a SQL AlwaysOn Availability Group. This setting is required when using the SQL AlwaysOn Availability Groups in SQL server. This setting can't be changed after you create the endpoint.
*
*/
public Optional enableFloatingIP() {
return Optional.ofNullable(this.enableFloatingIP);
}
/**
* @return Receive bidirectional TCP Reset on TCP flow idle timeout or unexpected connection termination. This element is only used when the protocol is set to TCP.
*
*/
public Optional enableTcpReset() {
return Optional.ofNullable(this.enableTcpReset);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return A reference to frontend IP addresses.
*
*/
public Optional frontendIPConfiguration() {
return Optional.ofNullable(this.frontendIPConfiguration);
}
/**
* @return The port for the external endpoint. Port numbers for each rule must be unique within the Load Balancer. Acceptable values range from 1 to 65534.
*
*/
public Optional frontendPort() {
return Optional.ofNullable(this.frontendPort);
}
/**
* @return The port range end for the external endpoint. This property is used together with BackendAddressPool and FrontendPortRangeStart. Individual inbound NAT rule port mappings will be created for each backend address from BackendAddressPool. Acceptable values range from 1 to 65534.
*
*/
public Optional frontendPortRangeEnd() {
return Optional.ofNullable(this.frontendPortRangeEnd);
}
/**
* @return The port range start for the external endpoint. This property is used together with BackendAddressPool and FrontendPortRangeEnd. Individual inbound NAT rule port mappings will be created for each backend address from BackendAddressPool. Acceptable values range from 1 to 65534.
*
*/
public Optional frontendPortRangeStart() {
return Optional.ofNullable(this.frontendPortRangeStart);
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return The timeout for the TCP idle connection. The value can be set between 4 and 30 minutes. The default value is 4 minutes. This element is only used when the protocol is set to TCP.
*
*/
public Optional idleTimeoutInMinutes() {
return Optional.ofNullable(this.idleTimeoutInMinutes);
}
/**
* @return The name of the resource that is unique within the set of inbound NAT rules used by the load balancer. This name can be used to access the resource.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The reference to the transport protocol used by the load balancing rule.
*
*/
public Optional protocol() {
return Optional.ofNullable(this.protocol);
}
/**
* @return The provisioning state of the inbound NAT rule resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Type of the resource.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InboundNatRuleResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable SubResourceResponse backendAddressPool;
private NetworkInterfaceIPConfigurationResponse backendIPConfiguration;
private @Nullable Integer backendPort;
private @Nullable Boolean enableFloatingIP;
private @Nullable Boolean enableTcpReset;
private String etag;
private @Nullable SubResourceResponse frontendIPConfiguration;
private @Nullable Integer frontendPort;
private @Nullable Integer frontendPortRangeEnd;
private @Nullable Integer frontendPortRangeStart;
private @Nullable String id;
private @Nullable Integer idleTimeoutInMinutes;
private @Nullable String name;
private @Nullable String protocol;
private String provisioningState;
private String type;
public Builder() {}
public Builder(InboundNatRuleResponse defaults) {
Objects.requireNonNull(defaults);
this.backendAddressPool = defaults.backendAddressPool;
this.backendIPConfiguration = defaults.backendIPConfiguration;
this.backendPort = defaults.backendPort;
this.enableFloatingIP = defaults.enableFloatingIP;
this.enableTcpReset = defaults.enableTcpReset;
this.etag = defaults.etag;
this.frontendIPConfiguration = defaults.frontendIPConfiguration;
this.frontendPort = defaults.frontendPort;
this.frontendPortRangeEnd = defaults.frontendPortRangeEnd;
this.frontendPortRangeStart = defaults.frontendPortRangeStart;
this.id = defaults.id;
this.idleTimeoutInMinutes = defaults.idleTimeoutInMinutes;
this.name = defaults.name;
this.protocol = defaults.protocol;
this.provisioningState = defaults.provisioningState;
this.type = defaults.type;
}
@CustomType.Setter
public Builder backendAddressPool(@Nullable SubResourceResponse backendAddressPool) {
this.backendAddressPool = backendAddressPool;
return this;
}
@CustomType.Setter
public Builder backendIPConfiguration(NetworkInterfaceIPConfigurationResponse backendIPConfiguration) {
if (backendIPConfiguration == null) {
throw new MissingRequiredPropertyException("InboundNatRuleResponse", "backendIPConfiguration");
}
this.backendIPConfiguration = backendIPConfiguration;
return this;
}
@CustomType.Setter
public Builder backendPort(@Nullable Integer backendPort) {
this.backendPort = backendPort;
return this;
}
@CustomType.Setter
public Builder enableFloatingIP(@Nullable Boolean enableFloatingIP) {
this.enableFloatingIP = enableFloatingIP;
return this;
}
@CustomType.Setter
public Builder enableTcpReset(@Nullable Boolean enableTcpReset) {
this.enableTcpReset = enableTcpReset;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("InboundNatRuleResponse", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder frontendIPConfiguration(@Nullable SubResourceResponse frontendIPConfiguration) {
this.frontendIPConfiguration = frontendIPConfiguration;
return this;
}
@CustomType.Setter
public Builder frontendPort(@Nullable Integer frontendPort) {
this.frontendPort = frontendPort;
return this;
}
@CustomType.Setter
public Builder frontendPortRangeEnd(@Nullable Integer frontendPortRangeEnd) {
this.frontendPortRangeEnd = frontendPortRangeEnd;
return this;
}
@CustomType.Setter
public Builder frontendPortRangeStart(@Nullable Integer frontendPortRangeStart) {
this.frontendPortRangeStart = frontendPortRangeStart;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder idleTimeoutInMinutes(@Nullable Integer idleTimeoutInMinutes) {
this.idleTimeoutInMinutes = idleTimeoutInMinutes;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder protocol(@Nullable String protocol) {
this.protocol = protocol;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("InboundNatRuleResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("InboundNatRuleResponse", "type");
}
this.type = type;
return this;
}
public InboundNatRuleResponse build() {
final var _resultValue = new InboundNatRuleResponse();
_resultValue.backendAddressPool = backendAddressPool;
_resultValue.backendIPConfiguration = backendIPConfiguration;
_resultValue.backendPort = backendPort;
_resultValue.enableFloatingIP = enableFloatingIP;
_resultValue.enableTcpReset = enableTcpReset;
_resultValue.etag = etag;
_resultValue.frontendIPConfiguration = frontendIPConfiguration;
_resultValue.frontendPort = frontendPort;
_resultValue.frontendPortRangeEnd = frontendPortRangeEnd;
_resultValue.frontendPortRangeStart = frontendPortRangeStart;
_resultValue.id = id;
_resultValue.idleTimeoutInMinutes = idleTimeoutInMinutes;
_resultValue.name = name;
_resultValue.protocol = protocol;
_resultValue.provisioningState = provisioningState;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy