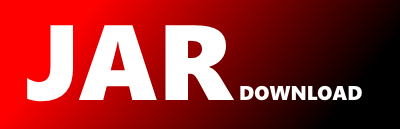
com.pulumi.azurenative.networkcloud.BmcKeySetArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud;
import com.pulumi.azurenative.networkcloud.enums.BmcKeySetPrivilegeLevel;
import com.pulumi.azurenative.networkcloud.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.networkcloud.inputs.KeySetUserArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BmcKeySetArgs extends com.pulumi.resources.ResourceArgs {
public static final BmcKeySetArgs Empty = new BmcKeySetArgs();
/**
* The object ID of Azure Active Directory group that all users in the list must be in for access to be granted. Users that are not in the group will not have access.
*
*/
@Import(name="azureGroupId", required=true)
private Output azureGroupId;
/**
* @return The object ID of Azure Active Directory group that all users in the list must be in for access to be granted. Users that are not in the group will not have access.
*
*/
public Output azureGroupId() {
return this.azureGroupId;
}
/**
* The name of the baseboard management controller key set.
*
*/
@Import(name="bmcKeySetName")
private @Nullable Output bmcKeySetName;
/**
* @return The name of the baseboard management controller key set.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy