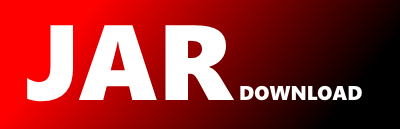
com.pulumi.azurenative.networkcloud.inputs.BgpServiceLoadBalancerConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud.inputs;
import com.pulumi.azurenative.networkcloud.enums.FabricPeeringEnabled;
import com.pulumi.azurenative.networkcloud.inputs.BgpAdvertisementArgs;
import com.pulumi.azurenative.networkcloud.inputs.IpAddressPoolArgs;
import com.pulumi.azurenative.networkcloud.inputs.ServiceLoadBalancerBgpPeerArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BgpServiceLoadBalancerConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final BgpServiceLoadBalancerConfigurationArgs Empty = new BgpServiceLoadBalancerConfigurationArgs();
/**
* The association of IP address pools to the communities and peers, allowing for announcement of IPs.
*
*/
@Import(name="bgpAdvertisements")
private @Nullable Output> bgpAdvertisements;
/**
* @return The association of IP address pools to the communities and peers, allowing for announcement of IPs.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy